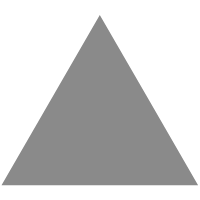
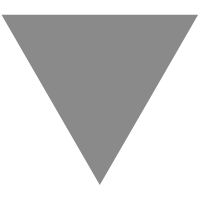
Selenium Handling Checkbox
source link: https://www.geeksforgeeks.org/selenium-handling-checkbox/
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
Selenium Handling Checkbox
Selenium is one of the most powerful tools for testing and automating web applications. the significance lies in its ability to perform repetitive tasks and automate web applications. It allows developers, testers, and QA professionals to interact with web applications and conduct automation tests.
One of the most common tasks in web applications is handling checkboxes in web forms. Checkboxes are graphical user interface elements that allow users to make binary choices by selecting or deselecting a small box. They are commonly used in web forms to gather user preferences or select multiple items from a list.
What is a CheckBox?
Imagine we are designing an online survey form for a website and we want to gather information about the user’s hobbies. We can use checkboxes to allow users to select one or more hobbies from a list of hobbies. Checkboxes are the graphical user interface elements that allow users to select or deselect an item from a list of options, they will enable users to make binary choices either selecting or deselecting an item from a list of options. In this tutorial, we’ll learn how to locate and handle checkboxes in Selenium Web Driver.
Here I’m using a sample website for practice
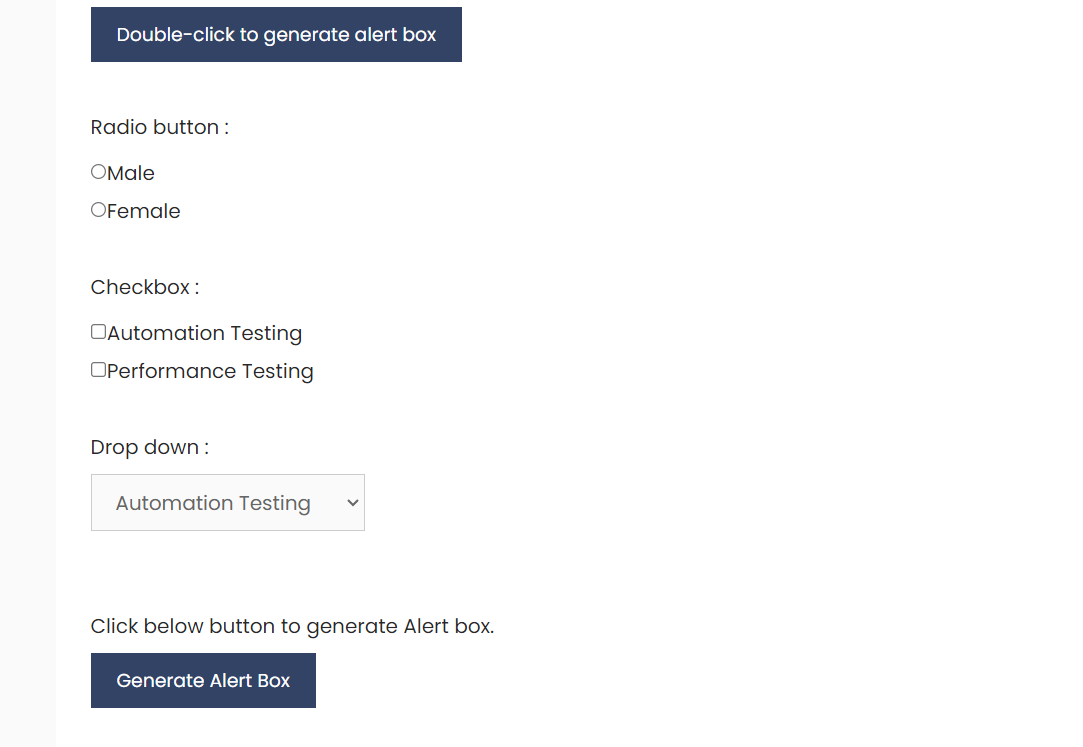
How to handle a Checkbox in Selenium-
To interact or handle a checkbox we first need to locate them on the web page then we check if the checkbox is selected or not and then we select or deselect a checkbox according to our need
Steps to handle checkbox in Selenium-
- Locate the Checkbox
- Check if the checkbox is Selected or Not
- Select or Deselect a Checkbox
1. How to Locate a Checkbox
Selenium provides multiple locators for locating a checkbox, some of them are-
- CLASSNAME
- XPATH
- CSS-SELECTOR
Let’s look into our sample page for a better understanding Here as we can see in our DOM, our checkbox has a class of Automation so we can use CLASS_NAME locator to locate it
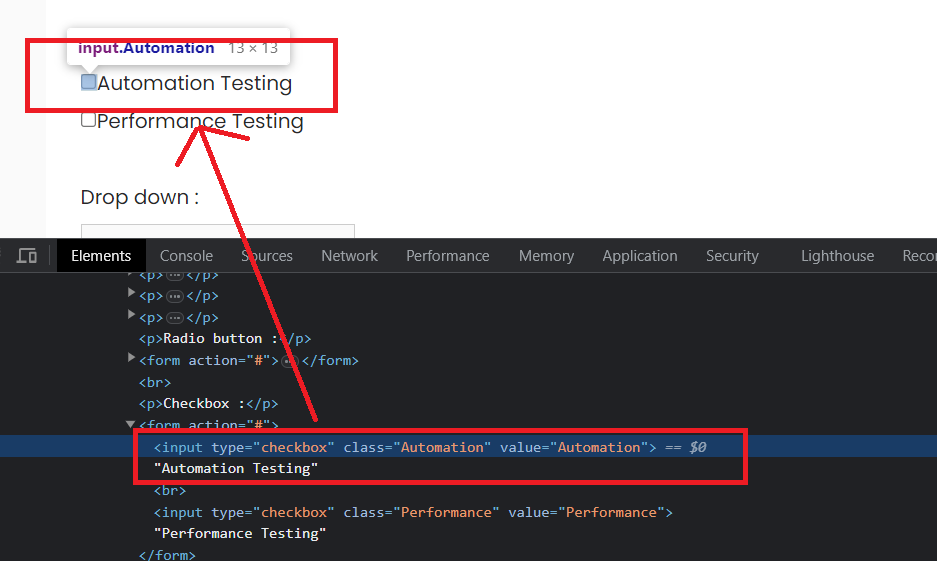
find_element()- is a method in selenium web driver which is used to find elements using a locator. It returns the first element that matches the condition.
find_elements()- find_elements is similar to find_element but instead of returning the first element, find_element returns a list of all the elements that match the condition
By:
We could also use other methods to locate the element such as
- By. ID
- By.NAME
- By.XPATH
- By.CSS_SELECTOR
- Python
# Importing modules from selenium import webdriver from selenium.common import NoSuchElementException from selenium.webdriver.common.by import By # Creating WebDriver instance for Chrome. driver = webdriver.Chrome(); # Define the URL of the webpage to be tested. # Navigating to the webpage. driver.get(url) # Try to locate the checkbox element by its CLASS_NAME try : checkbox = driver.find_element(By.CLASS_NAME, "Automation" ) except NoSuchElementException: # If the element is not found, print "Not Found". print ( "No Found" ) else : # If the element is found, print "Found". print ( "Found" ) # Close the WebDriver when done. driver.quit() |
Here if we have successfully located the element the Process finished with exit code 0
else it will throw a NoSuchElementException exception.
Output:
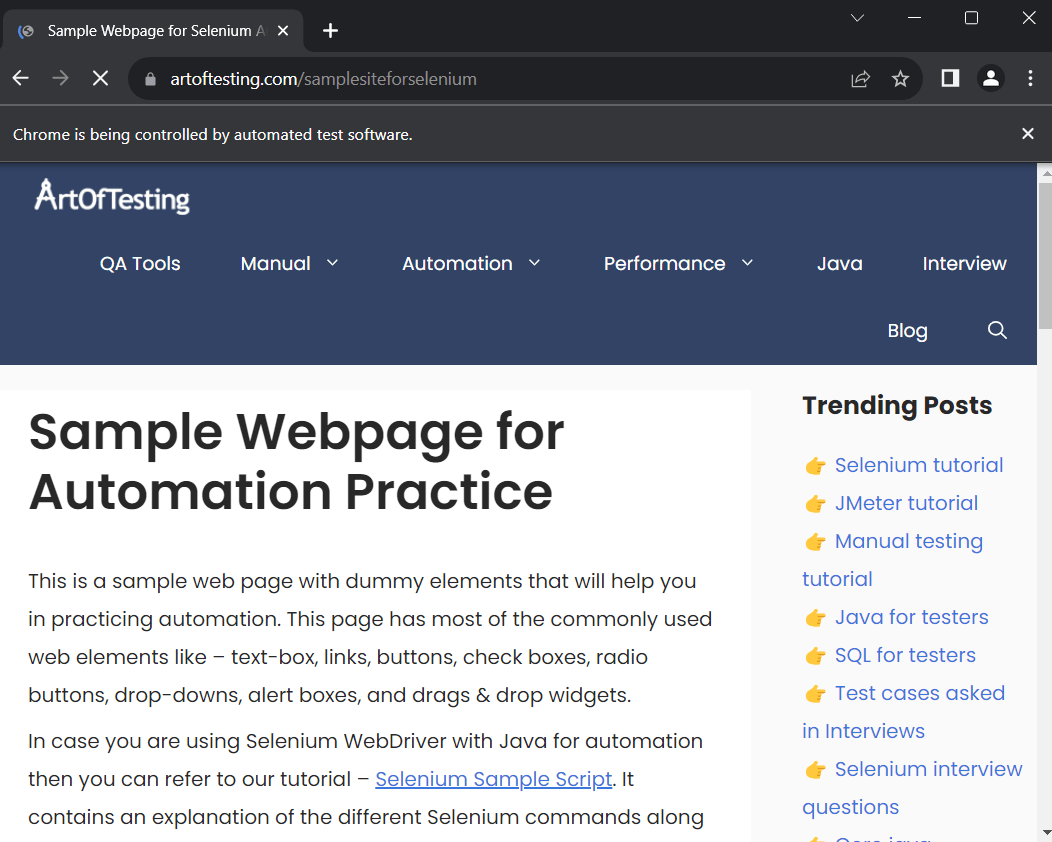
Selenium Handling Checkbox
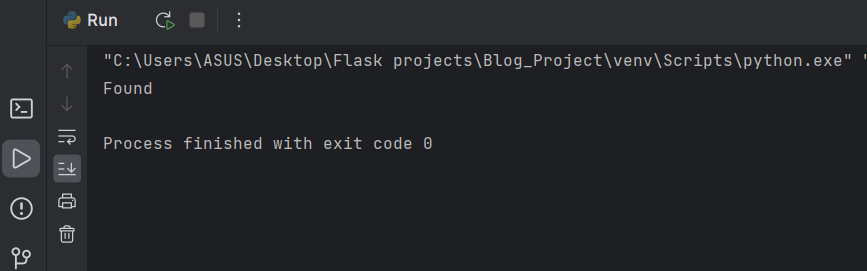
Selenium Handling Checkbox
2. How to check if the Checkbox is Selected or Not?
Selenium has a special method to check if a checkbox is selected or not
- is_selected()– used to check if a checkbox is Selected or Not, return true if it’s selected else false
- Python
# Importing modules. from selenium import webdriver from selenium.webdriver.common.by import By # Creating WebDriver instance for Chrome. driver = webdriver.Chrome() # Define the URL. # Open the webpage in the WebDriver. driver.get(url) # Locate the checkbox element by its class_Name. checkbox = driver.find_element(By.CLASS_NAME, "Automation" ) # Click on the checkbox. checkbox.click() # Check if the checkbox is selected. if checkbox.is_selected(): print ( "Checkbox is selected" ) # Close the WebDriver driver.quit() |
Output: False
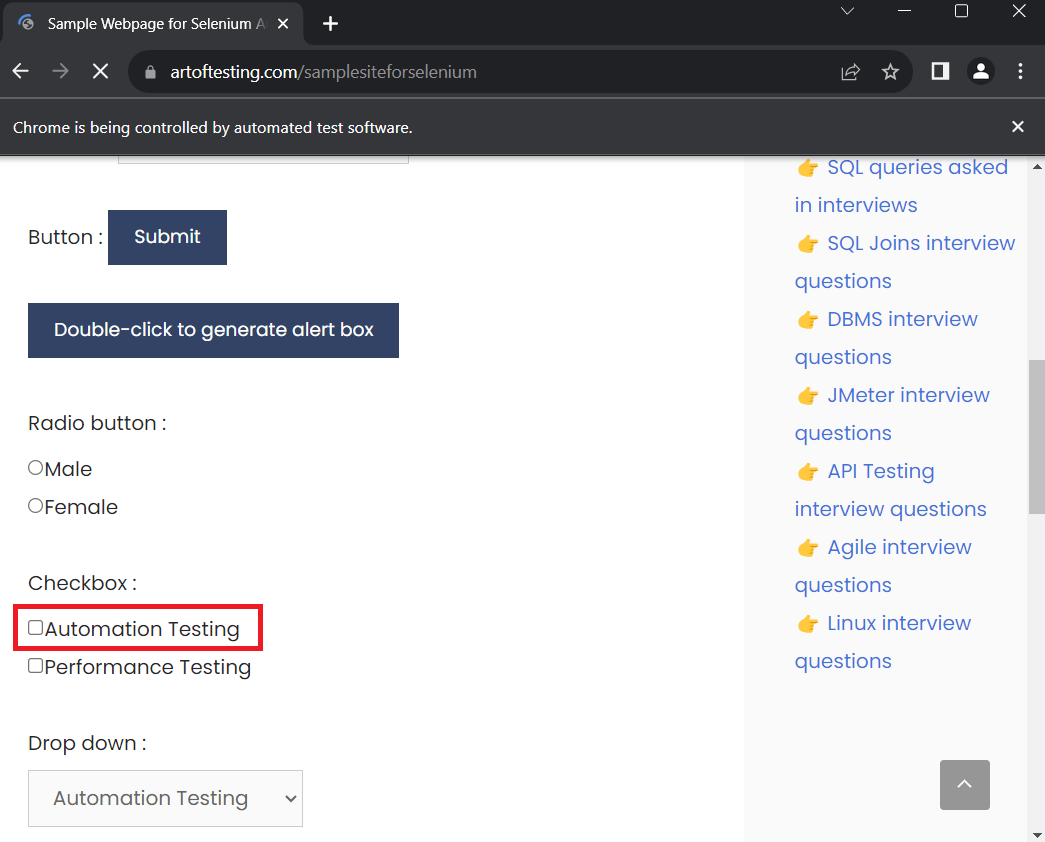
Selenium Handling Checkbox
The output of the following will be false as we have not selected the checkbox
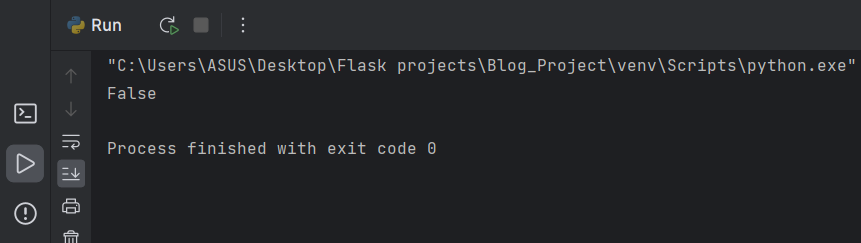
Selenium Handling Checkbox
3. How to select or deselect a checkbox?
Once we find the checkbox, the next thing we want to do is select or deselect the element. But to select or de-select an element we first have to check if it’s enabled or not.
Here are the methods which will help us do so.
- is_enabled()- used to check if an element is enabled or not. Return type- boolean
- click()- used to click an element
- Python
# Importing modules from Selenium from selenium import webdriver from selenium.webdriver.common.by import By # Initializing Chrome WebDriver driver = webdriver.Chrome() # write the URL of the website to be opened # Open the website in the Chrome browser driver.get(url) # Find the checkbox element by its class_Name checkbox = driver.find_element(By.CLASS_NAME, "Automation" ) # Check if the checkbox is enabled if checkbox.is_enabled(): # If the checkbox is enabled print a message (Checkbox is selected) print ( "Checkbox is selected" ) checkbox.click() # Close the browser window driver.quit() |
Output: selected
Here in the if block we check if our checkbox is enabled or not, and if it is enable we use the click() method to select the checkbox.
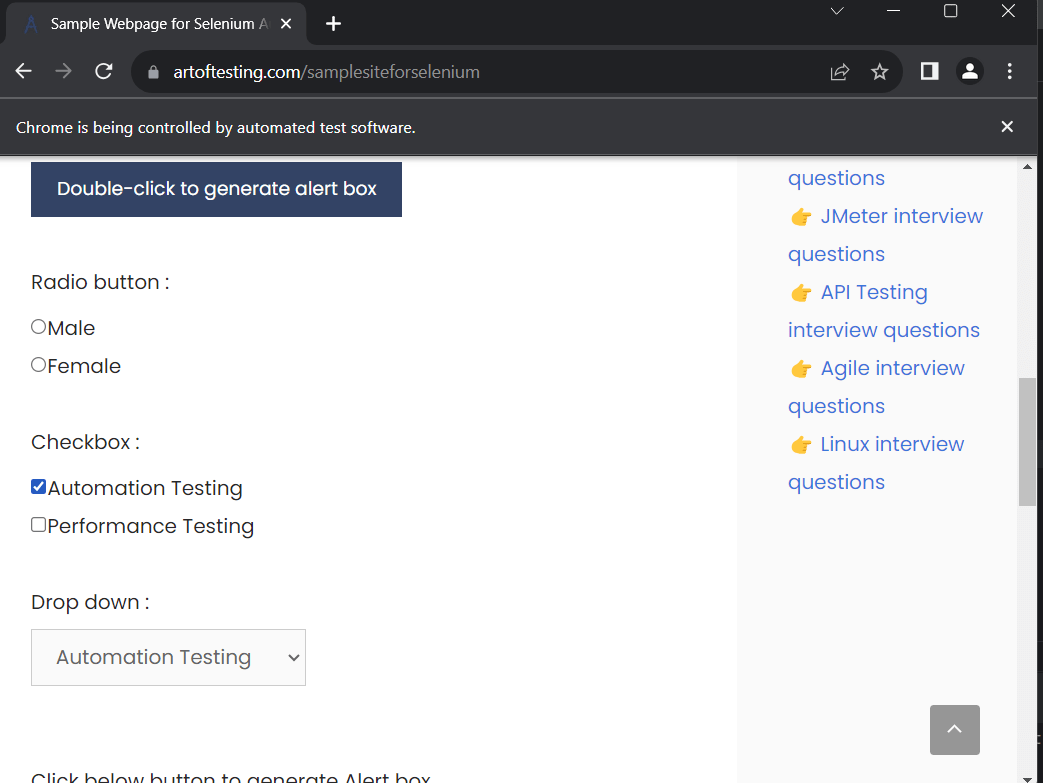
Selenium Handling Checkbox
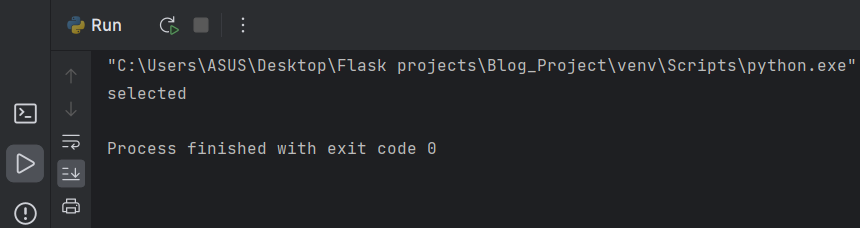
Selenium Handling Checkbox
How to Perform Validations On Checkbox using Selenium WebDriver?
Checkbox validations are important while automating web applications using Selenium. Validating checkboxes ensures that they function correctly and ensures that our web applications are working as expected. In this section, we’ll explore various techniques for performing checkbox validations using Selenium.
Steps to Perform Validations on CheckBox using Selenium WebDriver
STEP-1 Locate the CheckBox
Selenium provides multiple locators for locating a checkbox, some of them are-
- CLASSNAME
- XPATH
- CSS-SELECTOR
Locate the checkbox using one of the following methods with a suitable locator
find_element()– is a method in selenium web driver which is used to find elements using a locator. It returns the first element that matches the condition.
find_elements()– find_elements is similar to find_element but instead of returning the first element , find_element returns a list of all the elements that match the condition
- Python
# Importing necessary modules from Selenium from selenium import webdriver from selenium.common.exceptions import NoSuchElementException from selenium.webdriver.common.by import By # Initialize a Chrome WebDriver driver = webdriver.Chrome() # URL of the website # Open the URL driver.get(url) try : # find an element with the class_Name checkbox = driver.find_element(By.CLASS_NAME, "Automation" ) except NoSuchElementException: # If NoSuchElementException is happened, print "Not Found" print ( "Element not found" ) else : # If element is found, print "Found" print ( "Element found" ) # Close the WebDriver. driver.quit() |
Here if we have successfully located the element the Process finished with exit code 0 else it will throw a NoSuchElementException exception.
Output:
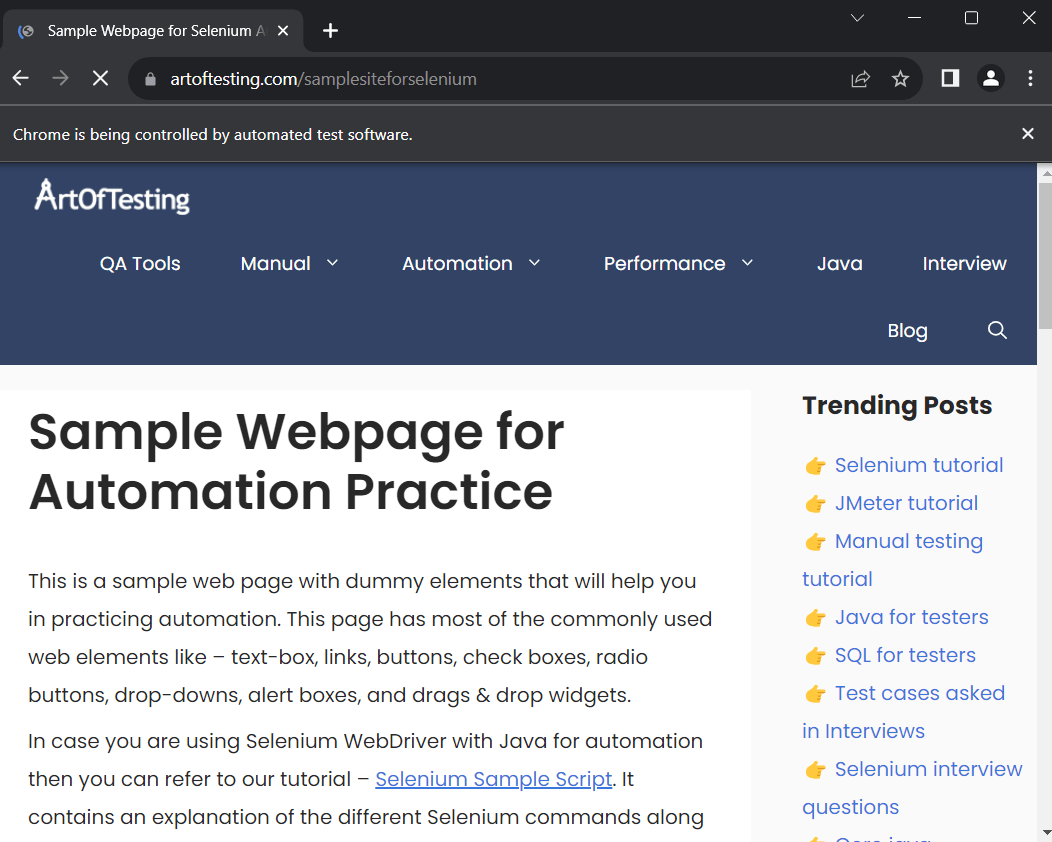
Selenium Handling Checkbox
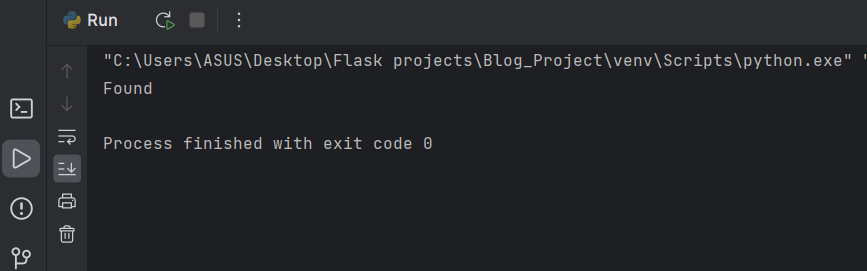
Selenium Handling Checkbox
STEP-2. Perform Actions on the Checkbox.
To interact with the checkbox we can use the ‘click()‘ method to toggle its state.
- Python
# Importing the necessary libraries from selenium from selenium import webdriver from selenium.webdriver.common.by import By # Initialize a Chrome WebDriver instance driver = webdriver.Chrome() # Define the URL # Open URL in the Chrome browser driver.get(url) # Locate the checkbox element by its class_Name checkbox = driver.find_element(By.CLASS_NAME, "Automation" ) # Click on the checkbox element checkbox.click() # Close browser driver.quit() |
The checkbox will be selected if it’s not selected and vice versa.
Output:
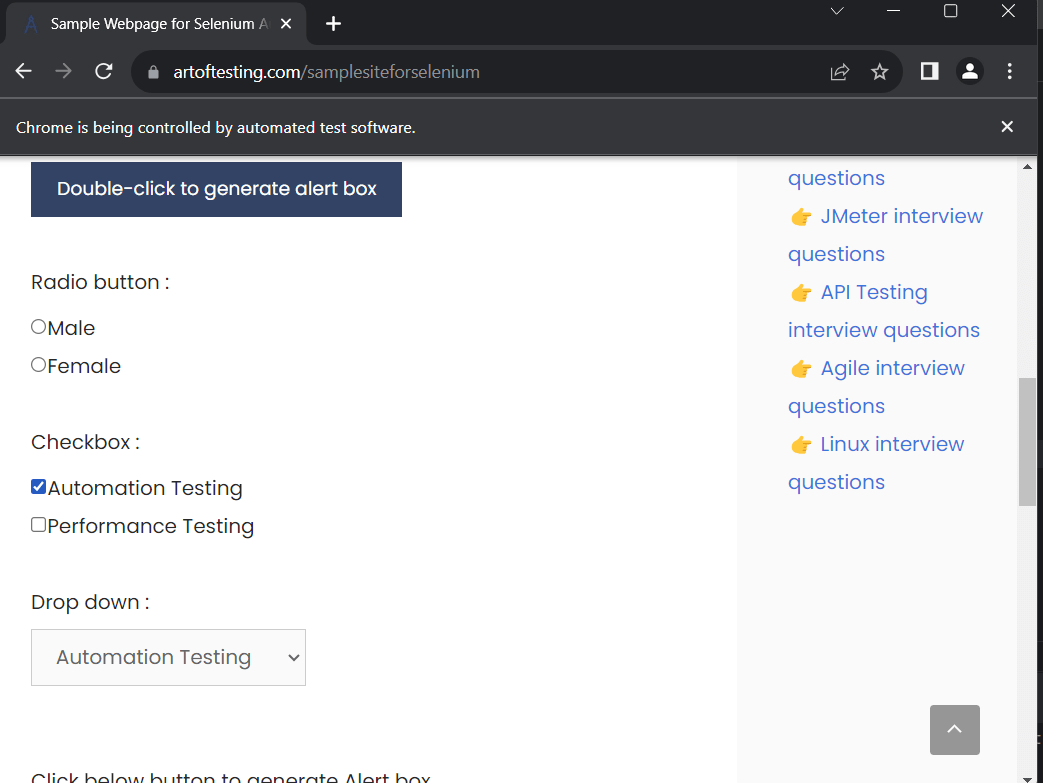
Selenium Handling Checkbox
STEP-3. Validate the Checkbox State
After performing actions on the checkbox we may want to validate its state. Selenium has a special method to validate the state
of the checkbox.
- is_selected()- used to check if a checkbox is Selected or Not, return true if it’s selected else false
- Python
from selenium import webdriver from selenium.webdriver.common.by import By # Step 1: Initialize the WebDriver # ***You need First appropriate WebDriver executable installed for Running Code.*** driver = webdriver.Chrome(); # Step 2: Open a web page with proper URL. driver.get(url) # Most commonly used locators in Selenium: # ID Locator: By.ID # Name Locator: By.NAME # XPath Locator: By.XPATH # CSS Selector Locator: By.CSS_SELECTOR checkbox = driver.find_element(By. ID , "ID_OF_ELEMENT" ) # Replace with the actual ID # Check the checkbox checkbox.click() # Check if the checkbox is selected if (checkbox.is_selected()): print ( "selected" ) # Close the WebDriver driver.quit() |
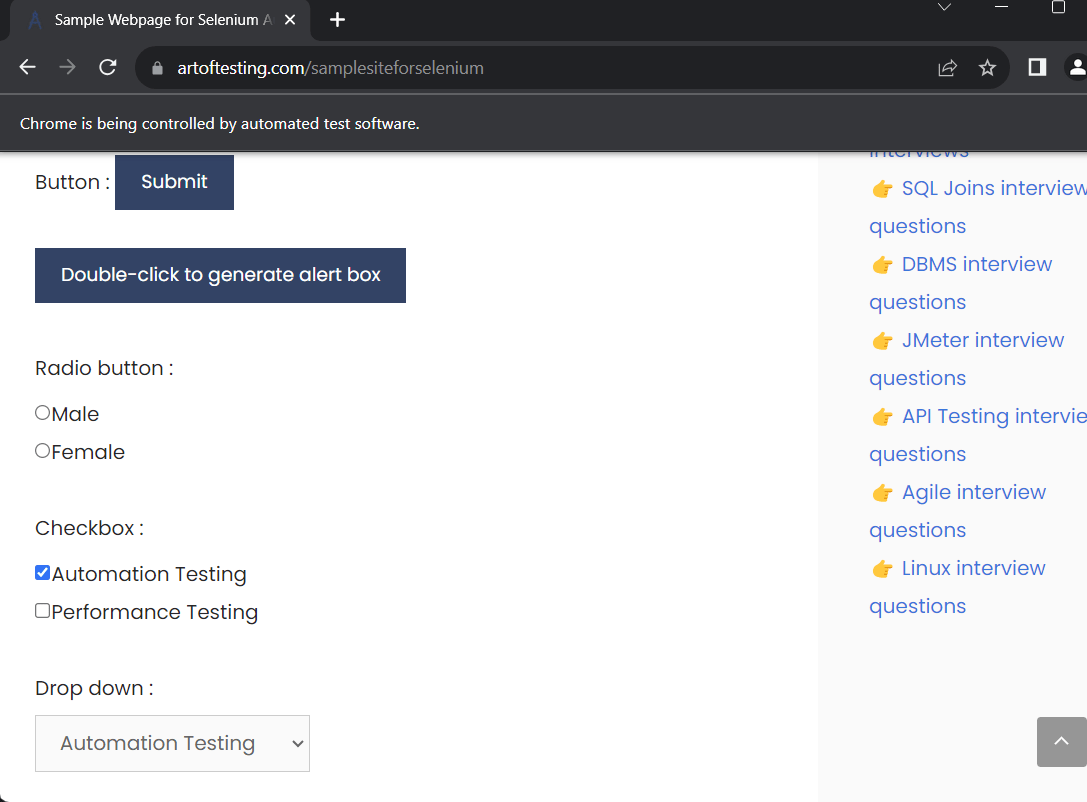
Selenium Handling Checkbox
Output: If the checkbox is selected it will print “checkbox is selected” in the console
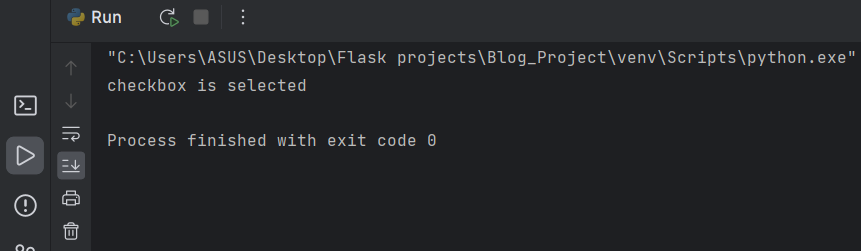
Selenium Handling Checkbox
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK