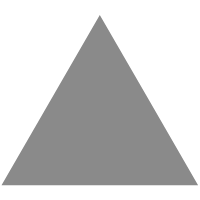
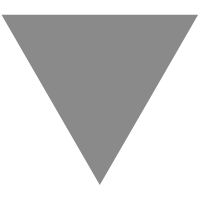
How to Remove All Whitespace Characters From a String in C#?
source link: https://code-maze.com/replace-whitespaces-string-csharp/
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
How to Remove All Whitespace Characters From a String in C#?
Want to build great APIs? Or become even better at it? Check our Ultimate ASP.NET Core Web API program and learn how to create a full production-ready ASP.NET Core API using only the latest .NET technologies. Bonus materials (Security book, Docker book, and other bonus files) are included in the Premium package!
In this article, we’re going to look at how to remove all whitespace characters from a string in C#. Strings often have trailing or leading whitespaces that we would like to remove, or we might want to remove all whitespaces in between too. Let’s take a look at several ways in which we can remove whitespace characters from a string.
Let’s start.
Use Regex to Remove Whitespaces
Regular expressions are very powerful in finding and replacing characters in a string, and we can easily use them to replace all whitespaces with an empty string.
Whitespace characters include not only the space character (" "
) but also the tab character (\t
) and the Carriage Return Line Feed (CRLF) characters (\r\n
).
Let’s create a class RemoveWhitespaceMethods
in which we will keep all our different methods to remove whitespaces. Let’s now also create a new static method RemoveWhitespacesUsingRegex()
within this class.
In this method, let’s create a string variable named source
containing multiple space and tab characters, and then use the Regex.Replace()
method to return a new string, where all occurrences of whitespace characters are replaced with an empty string:
public static string RemoveWhitespacesUsingRegex(string source) { return Regex.Replace(source, @"\s+", String.Empty); }
Now, let’s use this method to remove all whitespaces:
var sourceRegex = " \t hello worl d"; var resultRegex = RemoveWhitespaceMethods.RemoveWhitespacesUsingRegex(sourceRegex); Console.WriteLine(resultRegex); // prints 'helloworld'
Use LINQ to Remove Whitespace Characters
We can also use LINQ to remove all whitespace characters. This method not only removes leading, trailing, and whitespaces in between but also tabs and new lines, as these are also considered whitespace characters.
Let’s again make a new method called RemoveWhitespacesUsingLinq()
taking a string source
as a parameter. Within this method, we use the Where()
method from LINQ. Within the Where()
method, we pass in an expression that determines whether a given character is whitespace or not using char.IsWhiteSpace()
.
Finally, the String.Concat()
method combines the non-whitespace characters into a new string:
public static string RemoveWhitespacesUsingLinq(string source) { return String.Concat(source.Where(c => !char.IsWhiteSpace(c))); }
Now, let’s use this method to remove whitespaces in our string:
var sourceLinq = "hello \t world\r\n123"; var resultLinq = RemoveWhitespaceMethods.RemoveWhitespacesUsingLinq(sourceLinq); Console.WriteLine(resultLinq); //prints 'helloworld123'
Use String.Replace() to Remove Whitespaces
The String.Replace()
is a straightforward way of replacing all occurrences of a character within a string, with a different character, for instance, to replace a comma with a semicolon. In this case, we want to replace the whitespace characters with an empty string, i.e. with String.Empty
.
As the String.Replace()
method only replaces just a single character, we need to apply this multiple times to the output so the tab character (\t
) and the Carriage Return Line Feed (CRLF) characters (\r\n
) are replaced with an empty string as well.
Let’s create a method called RemoveWhitepacesUsingReplace()
which returns a new string with the whitespace characters removed. Keep in mind that this is suboptimal, as the String.Replace()
method creates a new object every time, so from a performance point of view other methods might be preferred:
public static string RemoveWhitespacesUsingReplace(string source) { return source.Replace(" ", "").Replace("\t", "").Replace("\r", "").Replace("\n", ""); }
Usage is similar to the examples before:
var sourceReplace = " Hello World!"; var resultReplace = RemoveWhitespaceMethods.RemoveWhitespacesUsingReplace(sourceReplace); Console.WriteLine(resultReplace); // prints 'HelloWorld!'
Use String.Trim() to Remove Whitespace Characters
The String.Trim()
method is slightly different, as this only removes leading and trailing whitespace characters, while whitespaces in the middle are unaffected. Both space characters, as well as tabs and carriage returns and line feeds are trimmed from the beginning and end of the string.
Often, one is interested in only removing leading and trailing whitespaces, in which this simple method can be useful. Let’s look at how we can trim the whitespace characters (including tabs for instance) from the beginning and end of the string while leaving the spaces in between unaffected. As earlier, we put this in a method, in this case RemoveLeadingAndTrailingWhitespacesUsingTrim()
:
public static string RemoveLeadingAndTrailingWhitespacesUsingTrim(string source) { return source.Trim(); }
Let’s also take a look at how this method works.
You can see that leading and trailing spaces are gone, but the space in between words is unaffected:
var sourceTrim = " \t John Doe "; var resultTrim = RemoveWhitespaceMethods.RemoveLeadingAndTrailingWhitespacesUsingTrim(sourceTrim); Console.WriteLine(resultTrim); //prints 'John Doe'
Use String.Split() and String.Join() to Remove Whitespaces
The String.Split()
method returns a string array where the elements in this array are delimited by a specified string. The String.Join()
method takes an array of strings and combines them into a new string.
To see how those two methods are combined to remove whitespaces, let’s make a static method called RemoveWhitespacesUsingSplitJoin()
:
public static string RemoveWhitespacesUsingSplitJoin(string source) { return String.Join("", source.Split(default(string[]), StringSplitOptions.RemoveEmptyEntries)); }
For the String.Split()
method, there are several overloads. In this case, we use Split(String[], StringSplitOptions)
. We pass in default(string[])
in the String.Split()
method because we want to pass in a null for the String[] separator
parameter, which is then interpreted as using whitespace characters as delimiters. We also pass StringSplitOptions.RemoveEmptyEntries
as the second argument, ensuring that empty entries are removed from the resulting array.
Then, we pass the output of the String.Split()
method into the String.Join()
method. We want to join the substrings without any space or commas in between, so we pass an empty string as the first argument.
Let’s also take a look at how we can use this, and it is similar to the other examples:
var sourceSplitJoin= " \t Hello World "; var resultSplitJoin = RemoveWhitespaceMethods.RemoveWhitespacesUsingSplitJoin(sourceSplitJoin); Console.WriteLine(resultSplitJoin); //prints 'HelloWorld'
Use StringBuilder to Remove Whitespace Characters
The last method we will look at is the StringBuilder. Let’s make a static method called RemoveWhitespacesUsingStringBuilder()
to remove all whitespace characters.
public static string RemoveWhitespacesUsingStringBuilder(string source) { var builder = new StringBuilder(source.Length); for (int i = 0; i < source.Length; i++) { char c = source[i]; if (!char.IsWhiteSpace(c)) builder.Append(c); } return builder.ToString(); }
Using the input string source
, we create a StringBuilder
and then loop over all characters of our source
string. If the character does not equal to any whitespace character, we append it to our StringBuilder
. After looping over all the characters, we create a new string from our builder.
Now, let’s use our method to create a new string without whitespace characters:
var sourceStringBuilder = " Hello World! \r\n"; var resultStringBuilder = RemoveWhitespaceMethods.RemoveWhitespacesUsingStringBuilder(sourceStringBuilder); Console.WriteLine(resultStringBuilder); //prints "HelloWorld!"
Conclusion
In this article, we explored different methods of removing whitespace characters in a string. We saw that different methods are leading to more or less the same result. You can prefer one method over the other depending on your preference.
Want to build great APIs? Or become even better at it? Check our Ultimate ASP.NET Core Web API program and learn how to create a full production-ready ASP.NET Core API using only the latest .NET technologies. Bonus materials (Security book, Docker book, and other bonus files) are included in the Premium package!
Share:
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK