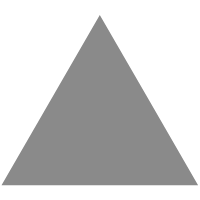
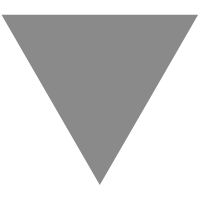
Laravel Excel Import Export with Example.
source link: https://www.laravelcode.com/post/laravel-excel-import-export-with-example
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
Laravel Excel Import Export with Example.
In this article, we will discuss Laravel 5 Export Data Excel/CSV. To achieve this functionality, we are using “Maatwebsite/Laravel-Excel” package. Here I will explain you step by step to export the data to Excel or CSV as per your needs. “Maatwebsite/Laravel-Excel” package is one of a stable package for generating CSV and Excel file. This package also provides the feature to import CSV file to the databaseBefore proceeding, I’m assuming you are familiar with the Basic Laravel installation, Authentication, and the MVC (Model View Controller) structure. If no then first go through the given articles which helps you to understand the basics of Laravel.
Install “Maatwebsite/Laravel-Excel” package
We have to install the package using given composer command.
composer require maatwebsite/excel
You can also use second way to add this package to your project. Add the given code in your composer JSON and run composer update command to install the package.
require: {
...
"maatwebsite/excel": "^3.0"
...
}
After that, you have to update your config/app.php and add provider and aliases array.
'providers' => [
....
Maatwebsite\Excel\ExcelServiceProvider::class,
...
],
'aliases' => [
....
'Excel' => Maatwebsite\Excel\Facades\Excel::class,
...
],
To publish the package config, run the vendor publish command:
php artisan vendor:publish
.This command shows you the available packages. Then you can select the package Maatwebsite to publish the config. This will create a new config file named config/excel.php.
Register Route
Now, add given routes to your routes/web.php.
// List all alrticle
Route::get('/articles','PostsController@index');
// Export to excel
Route::get('/articles/exportExcel','PostsController@exportExcel');
// Export to csv
Route::get('/articles/exportCSV','PostsController@exportCSV');
Create Post Model
Use gave artisan command to create post model.
php artisan make:model Post
After that add fillable in your model.
<?php
namespace App;
use Illuminate\Database\Eloquent\Model;
class Post extends Model
{
public $table = 'post';
protected $fillable = [
'post_title',
'post_content'
];
}
Create a custom export class
Create a new class called PostExport in App/Exports. In this class, we will handle the logic behind export functionality.
<?php
namespace App\Exports;
use App\Post;
use Maatwebsite\Excel\Concerns\FromCollection;
class PostExport implements FromCollection
{
public function collection()
{
return Post::all();
}
}
In this class, create collection function which one returns an array of all the articles.
Create Post Controller
Use given artisan command to create post controller.
php artisan make:controller PostsController
After that create three methods as per the registered routes.
<?php
namespace App\Http\Controllers;
use Illuminate\Http\Request;
use Maatwebsite\Excel\Facades\Excel;
use App\Post;
use App\Exports\ListExport;
class PostsController extends Controller
{
/**
* Get list of articles
*/
public function index()
{
$list = Post::get();
return view('list',compact('list'));
}
/**
* Export to excel
*/
public function exportExcel()
{
return Excel::download(new ListExport, 'list.xlsx');
}
/**
* Export to csv
*/
public function exportCSV()
{
return Excel::download(new ListExport, 'list.csv');
}
}
Finally, Basic export functionality is successfully done. As I already mentioned package provides rich features for import and export data. We will discuss more on this topic. Feel free to comment if any query.
Author : Harsukh Makwana

Hi, My name is Harsukh Makwana. i have been work with many programming language like php, python, javascript, node, react, anguler, etc.. since last 5 year. if you have any issue or want me hire then contact me on [email protected]
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK