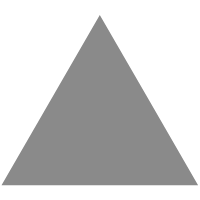
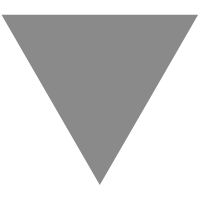
How to Change the Class of an Element Using JavaScript
source link: https://www.laravelcode.com/post/how-to-change-the-class-of-an-element-using-javascript
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
How to Change the Class of an Element Using JavaScript
Use the classList
Property
In modern browsers you can use the DOM element's classList
property to add, remove or toggle CSS classes to the HTML elements dynamically with JavaScript.
The following example will show you how to change the class of a DIV element onclick of the button. It works all major browsers such as Chrome, Firefox, Microsoft Edge, Safari, etc.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8">
<title>Change an Element's Class with JavaScript</title>
<style>
.highlight{
background: yellow;
}
.bordered{
border: 5px solid #000;
}
.padded{
padding: 20px;
}
.hide{
display: none;
}
</style>
</head>
<body>
<div id="content">
<h1>This is a heading</h1>
<p>This is a paragraph of text.</p>
</div>
<p>
<button type="button" onclick="addSingleClass()">Add Highlight Class</button>
<button type="button" onclick="addMultipleClass()">Add Bordered and Padded Class</button>
</p>
<p>
<button type="button" onclick="removeSingleClass()">Remove Highlight Class</button>
<button type="button" onclick="removeMultipleClass()">Remove Bordered and Padded Class</button>
</p>
<p>
<button type="button" onclick="toggleClass()">Toggle Hide Class</button>
</p>
<script>
// Selecting element
var elem = document.getElementById("content");
function addSingleClass(){
elem.classList.add("highlight"); // Add a highlight class
}
function addMultipleClass(){
elem.classList.add("bordered", "padded"); // Add bordered and padded class
}
function removeSingleClass(){
elem.classList.remove("highlight"); // Remove highlight class
}
function removeMultipleClass(){
elem.classList.remove("bordered", "padded"); // Remove bordered and padded class
}
function toggleClass(){
elem.classList.toggle("hide"); // Toggle hide class
}
</script>
</body>
</html>
Alternatively, to support older browsers you can use the className
property. The following example demonstrates how to add or replace CSS classes using this property.
<div id="info">Some important information!</div>
<script>
// Selecting element
var elem = document.getElementById("info");
elem.className = "note"; // Add or replace all classes with note class
elem.className += " highlight"; // Add a new class highlight
elem.className += " bordered padded"; // Add two new classes bordered and padded
elem.className = ""; // Remove all classes
</script>
Author : Harsukh Makwana

Hi, My name is Harsukh Makwana. i have been work with many programming language like php, python, javascript, node, react, anguler, etc.. since last 5 year. if you have any issue or want me hire then contact me on [email protected]
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK