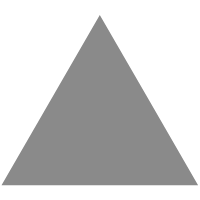
1
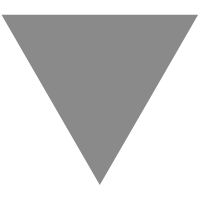
java | IO 任务
source link: https://benpaodewoniu.github.io/2023/01/23/java188/
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
IO 任务
姑苏城外一茅屋,万树梅花月满天
一个简单的 IO
任务。
package com.redisc;
import io.netty.bootstrap.ServerBootstrap;
import io.netty.buffer.ByteBuf;
import io.netty.channel.ChannelHandlerContext;
import io.netty.channel.ChannelInboundHandlerAdapter;
import io.netty.channel.ChannelInitializer;
import io.netty.channel.nio.NioEventLoopGroup;
import io.netty.channel.socket.nio.NioServerSocketChannel;
import io.netty.channel.socket.nio.NioSocketChannel;
import lombok.extern.slf4j.Slf4j;
import java.io.IOException;
import java.nio.charset.Charset;
@Slf4j
public class Run {
public static void main(String[] args) throws IOException {
new ServerBootstrap()
.group(new NioEventLoopGroup())
.channel(NioServerSocketChannel.class)
.childHandler(new ChannelInitializer<NioSocketChannel>() {
@Override
protected void initChannel(NioSocketChannel nioSocketChannel) throws Exception {
nioSocketChannel.pipeline().addLast(new ChannelInboundHandlerAdapter() {
@Override
public void channelRead(ChannelHandlerContext ctx, Object msg) throws Exception {
ByteBuf buffer = (ByteBuf) msg;
log.debug(buffer.toString(Charset.defaultCharset()));
}
});
}
}).bind(8000);
}
}
package com.redisc;
import io.netty.bootstrap.Bootstrap;
import io.netty.channel.Channel;
import io.netty.channel.ChannelInitializer;
import io.netty.channel.nio.NioEventLoopGroup;
import io.netty.channel.socket.nio.NioSocketChannel;
import io.netty.handler.codec.string.StringEncoder;
import java.io.IOException;
import java.net.InetSocketAddress;
public class Client {
public static void main(String[] args) throws IOException, InterruptedException {
Channel channel = new Bootstrap()
.group(new NioEventLoopGroup())
.channel(NioSocketChannel.class)
.handler(new ChannelInitializer<NioSocketChannel>() {
@Override
protected void initChannel(NioSocketChannel nioSocketChannel) throws Exception {
nioSocketChannel.pipeline().addLast(new StringEncoder());
}
}).connect(new InetSocketAddress("localhost", 8000))
.sync().channel();
channel.writeAndFlush("hello");
}
}
在 26
处打个断点。
- 打开服务端
debug
模式开启client
「debug
模式是thread
模式」- 在
26
处,不断的发送信息,然后关闭客户端,重启一个客户端,发送信息,重复3
次
- 在
21:38:33.198 [nioEventLoopGroup-2-2] DEBUG com.redisc.Run - hello // 第一个客户端
21:38:37.001 [nioEventLoopGroup-2-2] DEBUG com.redisc.Run - hello // 第一个客户端
21:38:37.851 [nioEventLoopGroup-2-2] DEBUG com.redisc.Run - hello // 第一个客户端
21:38:40.985 [nioEventLoopGroup-2-2] DEBUG com.redisc.Run - 234 // 第一个客户端
21:38:44.990 [nioEventLoopGroup-2-2] DEBUG com.redisc.Run - hello // 第一个客户端
21:38:54.830 [nioEventLoopGroup-2-3] DEBUG com.redisc.Run - 123 // 第二个客户端
21:38:57.230 [nioEventLoopGroup-2-3] DEBUG com.redisc.Run - 123 // 第二个客户端
21:38:57.499 [nioEventLoopGroup-2-3] DEBUG com.redisc.Run - 123 // 第二个客户端
21:38:57.771 [nioEventLoopGroup-2-3] DEBUG com.redisc.Run - 123 // 第二个客户端
21:39:02.297 [nioEventLoopGroup-2-3] DEBUG com.redisc.Run - hello // 第二个客户端
21:39:09.959 [nioEventLoopGroup-2-4] DEBUG com.redisc.Run - hello // 第三个客户端
21:39:10.785 [nioEventLoopGroup-2-4] DEBUG com.redisc.Run - hello // 第三个客户端
可以发现,每一个客户端发送的信息都是由一个 eventloop
里面的一个单独的处理器处理。
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK