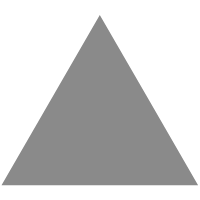
7
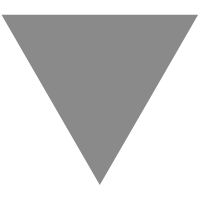
Matter js social links - JSFiddle - Code Playground
source link: https://jsfiddle.net/flol3622/2x8baywj/8/
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
Matter js social links
xxxxxxxxxx
<header class="container">
<h1>My links</h1>
</header>
<canvas id="canvas" style="position: absolute; top:0px; left:0px; height: 100%; width:100%;"></canvas>
<div class="bubble" data-url="http://www.example.com">
<img src="https://img.icons8.com/color/70/null/whatsapp--v1.png" />
<p>WhatsApp</p>
</div>
<div class="bubble" data-url="http://www.example.com">
<img src="https://img.icons8.com/color/70/null/facebook-messenger--v1.png" />
<p>Messenger</p>
</div>
<div class="bubble" data-url="http://www.example.com">
<img src="https://img.icons8.com/color/70/null/facebook.png" />
<p>Facebook</p>
</div>
<div class="bubble" data-url="http://www.example.com">
<img src="https://img.icons8.com/color/70/null/linkedin.png" />
<p>LinkedIn</p>
</div>
<div class="bubble" data-url="http://www.example.com">
<img src="https://img.icons8.com/ios-glyphs/70/null/github.png" />
<p>GitHub</p>
</div>
<div class="bubble" data-url="http://www.example.com">
<img src="https://img.icons8.com/color/70/null/youtube-play.png" />
<p>YouTube</p>
</div>
<div class="bubble" data-url="http://www.example.com">
<img src="https://img.icons8.com/ios/60/null/new-post--v1.png" style="height: 50px; padding: 5px" />
<p>Mail</p>
</div>
<div class="bubble" data-url="http://www.example.com">
<img src="https://img.icons8.com/color/70/null/instagram-new--v1.png" />
<p>Instagram</p>
</div>
<div class="bubble" data-url="http://www.example.com">
<img src="https://img.icons8.com/ios/96/null/phone--v1.png" style="height: 40px; padding: 5px" />
<p>Phone</p>
</div>
xxxxxxxxxx
// module aliases
var Engine = Matter.Engine,
Render = Matter.Render,
World = Matter.World,
Bodies = Matter.Bodies,
Events = Matter.Events;
// create an engine
var engine = Engine.create();
// create a renderer
var render = Render.create({
canvas: document.getElementById("canvas"),
engine: engine,
options: {
wireframes: false,
width: window.innerWidth,
height: window.innerHeight,
background: "#00",
},
});
// get the canvas size
var canvas = render.canvas;
// create boundaries around the edge of the canvas
var boundaries = [
// top boundary
Bodies.rectangle(canvas.width / 2, 0, canvas.width, 5, {
isStatic: true,
render: {
fillStyle: "#FFFFFF",
},
}),
// bottom boundary
Bodies.rectangle(canvas.width / 2, canvas.height, canvas.width, 5, {
isStatic: true,
render: {
fillStyle: "#FFFFFF",
},
}),
// left boundary
Bodies.rectangle(0, canvas.height / 2, 5, canvas.height, {
isStatic: true,
render: {
fillStyle: "#FFFFFF",
},
}),
// right boundary
Bodies.rectangle(canvas.width, canvas.height / 2, 5, canvas.height, {
isStatic: true,
render: {
fillStyle: "#FFFFFF",
},
}),
];
// add the boundaries to the world
World.add(engine.world, boundaries);
// create necessary bodies
var bodiesDom = document.getElementsByClassName("bubble");
var bodies = [];
for (var i = 0, l = bodiesDom.length; i < l; i++) {
var body = Bodies.circle(
Math.floor(Math.random() * canvas.width),
Math.floor(Math.random() * canvas.height),
50,
{
restitution: 0.4,
// style the body
render: {
fillStyle: "#ffffff00",
// strokeStyle: "blue",
// lineWidth: 10,
},
// add custom link to body
url: bodiesDom[i].dataset.url,
}
);
bodiesDom[i].id = body.id;
bodies.push(body);
}
console.log(bodies);
World.add(engine.world, bodies);
// add mouse control (dragging)
var mouse = Matter.Mouse.create(render.canvas),
mouseConstraint = Matter.MouseConstraint.create(engine, {
mouse: mouse,
constraint: {
stiffness: 0.2,
render: {
visible: false,
},
},
});
World.add(engine.world, mouseConstraint);
// add mouse control (clicking)
var startTime = null;
Events.on(mouseConstraint, "mousedown", function (event) {
startTime = Date.now();
});
Events.on(mouseConstraint, "mouseup", function (event) {
var endTime = Date.now();
var timeInterval = endTime - startTime;
var maxTimeInterval = 100; // maximum time interval in milliseconds
if (timeInterval <= maxTimeInterval) {
// Execute the action you want to perform on click
var mouseConstraint = event.source;
var bodies = engine.world.bodies;
if (!mouseConstraint.bodyB) {
for (i = 0; i < bodies.length; i++) {
var body = bodies[i];
if (
Matter.Bounds.contains(body.bounds, mouseConstraint.mouse.position)
) {
var bodyUrl = body.url;
console.log("Body.Url >> " + bodyUrl);
// Hyperlinking feature
if (bodyUrl != undefined) {
window.open(bodyUrl, "_blank");
console.log("Hyperlink was opened");
}
break;
}
}
}
}
});
// run the engine
Engine.run(engine);
// run the renderer
Render.run(render);
// update position divs with position bodies
window.requestAnimationFrame(update);
function update() {
for (var i = 0, l = bodiesDom.length; i < l; i++) {
var bodyDom = bodiesDom[i];
var body = null;
for (var j = 0, k = bodies.length; j < k; j++) {
if (bodies[j].id == bodyDom.id) {
body = bodies[j];
break;
}
}
if (body === null) continue;
if (body.position.x < bodyDom.offsetWidth / 2) {
body.position.x = bodyDom.offsetWidth / 2;
}
if (body.position.x > window.innerWidth - bodyDom.offsetWidth / 2) {
body.position.x = window.innerWidth - bodyDom.offsetWidth / 2;
}
if (body.position.y < bodyDom.offsetHeight / 2) {
body.position.y = bodyDom.offsetHeight / 2;
}
if (body.position.y > window.innerHeight - bodyDom.offsetHeight / 2) {
body.position.y = window.innerHeight - bodyDom.offsetHeight / 2;
}
bodyDom.style.transform =
"translate( " +
(body.position.x - bodyDom.offsetWidth / 2) +
"px, " +
(body.position.y - bodyDom.offsetHeight / 2) +
"px )";
}
window.requestAnimationFrame(update);
}
xxxxxxxxxx
.bubble {
position: absolute;
top: 0px;
left: 0px;
font-size: 30px;
-webkit-touch-callout: none;
-webkit-user-select: none;
-khtml-user-select: none;
-moz-user-select: none;
-ms-user-select: none;
user-select: none;
pointer-events: none;
display: flex;
flex-direction: column;
align-items: center;
height: 100px;
width: 100px;
border-radius: 50%;
padding-top: 9px;
border: 1px solid rgb(77, 77, 77);
background-color: rgba(255, 255, 255, 0.719);
}
.bubble img {
max-height: 48px;
max-width: 48px;
}
.bubble p {
font-size: 15px;
}
.clickable {
pointer-events: auto;
}
295px
Console (beta)
- JSFiddle Console (beta). Turn on/off in Editor settings.
- ☁️ "Running fiddle"
- [{ angle: 0, anglePrev: 0, angularSpeed: 0, angularVelocity: 0, area: 7777.744665999999, axes: [{ x: -0.970952042258738, y: -0.239274176696078 }, { x: -0.8854431390332113, y: -0.46474772461951164 }, { x: -0.748538767034939, y: -0.6630910301352396 }, { x: -0.5680489228149688, y: -0.8229947881297631 }, { x: -0.35459420851145496, y: -0.9350202924483163 }, { x: -0.12054195746334154, y: -0.992708233314757 }, { x: 0.12054195746334154, y: -0.992708233314757 }, { x: 0.35459420851145496, y: -0.9350202924483163 }, { x: 0.5680489228149688, y: -0.8229947881297631 }, { x: 0.748538767034939, y: -0.6630910301352396 }, { x: 0.8854431390332113, y: -0.46474772461951164 }, { x: 0.970952042258738, y: -0.239274176696078 }, { x: 1, y: 0 }], bounds: { max: { ... }, min: { ... } }, circleRadius: 50, collisionFilter: { category: 1, group: 0, mask: 4294967295 }, constraintImpulse: { angle: 0, x: 0, y: 0 }, density: 0.001, force: { x: 0, y: 0 }, friction: 0.1, frictionAir: 0.01, frictionStatic: 0.5, id: 5, inertia: 38511.973373149114, inverseInertia: 0.000025965950648927504, inverseMass: 0.128571975931718, isSensor: false, isSleeping: false, isStatic: false, label: "Circle Body", mass: 7.7777446659999985, motion: 0, parent: [circular object Object], parts: [[circular object Object]], plugin: { ... }, position: { x: 144, y: 122 }, positionImpulse: { x: 0, y: 0 }, positionPrev: { x: 144, y: 122 }, render: { fillStyle: "#ffffff00", lineWidth: 0, opacity: 1, sprite: { ... }, strokeStyle: "#000", visible: true }, restitution: 0.4, sleepCounter: 0, sleepThreshold: 60, slop: 0.05, speed: 0, timeScale: 1, torque: 0, totalContacts: 0, type: "body", url: "http://www.example.com", velocity: { x: 0, y: 0 }, vertices: [{ body: [circular object Object], index: 0, isInternal: false, x: 193.635, y: 128.027 }, { body: [circular object Object], index: 1, isInternal: false, x: 190.751, y: 139.73 }, { body: [circular object Object], index: 2, isInternal: false, x: 185.149, y: 150.403 }, { body: [circular object Object], index: 3, isInternal: false, x: 177.156, y: 159.426 }, { body: [circular object Object], index: 4, isInternal: false, x: 167.236, y: 166.273 }, { body: [circular object Object], index: 5, isInternal: false, x: 155.966, y: 170.547 }, { body: [circular object Object], index: 6, isInternal: false, x: 144, y: 172 }, { body: [circular object Object], index: 7, isInternal: false, x: 132.034, y: 170.547 }, { body: [circular object Object], index: 8, isInternal: false, x: 120.764, y: 166.273 }, { body: [circular object Object], index: 9, isInternal: false, x: 110.844, y: 159.426 }, { body: [circular object Object], index: 10, isInternal: false, x: 102.851, y: 150.403 }, { body: [circular object Object], index: 11, isInternal: false, x: 97.249, y: 139.73 }, { body: [circular object Object], index: 12, isInternal: false, x: 94.36500000000001, y: 128.027 }, { body: [circular object Object], index: 13, isInternal: false, x: 94.36500000000001, y: 115.973 }, { body: [circular object Object], index: 14, isInternal: false, x: 97.249, y: 104.27 }, { body: [circular object Object], index: 15, isInternal: false, x: 102.851, y: 93.59700000000001 }, { body: [circular object Object], index: 16, isInternal: false, x: 110.844, y: 84.574 }, { body: [circular object Object], index: 17, isInternal: false, x: 120.764, y: 77.727 }, { body: [circular object Object], index: 18, isInternal: false, x: 132.034, y: 73.453 }, { body: [circular object Object], index: 19, isInternal: false, x: 144, y: 72 }, { body: [circular object Object], index: 20, isInternal: false, x: 155.966, y: 73.453 }, { body: [circular object Object], index: 21, isInternal: false, x: 167.236, y: 77.727 }, { body: [circular object Object], index: 22, isInternal: false, x: 177.156, y: 84.574 }, { body: [circular object Object], index: 23, isInternal: false, x: 185.149, y: 93.59700000000001 }, { body: [circular object Object], index: 24, isInternal: false, x: 190.751, y: 104.27 }, { body: [circular object Object], index: 25, isInternal: false, x: 193.635, y: 115.973 }] }, { angle: 0, anglePrev: 0, angularSpeed: 0, angularVelocity: 0, area: 7777.744665999999, axes: [{ x: -0.970952042258738, y: -0.239274176696078 }, { x: -0.8854431390332113, y: -0.46474772461951164 }, { x: -0.748538767034939, y: -0.6630910301352396 }, { x: -0.5680489228149688, y: -0.8229947881297631 }, { x: -0.35459420851145496, y: -0.9350202924483163 }, { x: -0.12054195746334154, y: -0.992708233314757 }, { x: 0.12054195746334154, y: -0.992708233314757 }, { x: 0.35459420851145496, y: -0.9350202924483163 }, { x: 0.5680489228149688, y: -0.8229947881297631 }, { x: 0.748538767034939, y: -0.6630910301352396 }, { x: 0.8854431390332113, y: -0.46474772461951164 }, { x: 0.970952042258738, y: -0.239274176696078 }, { x: 1, y: 0 }], bounds: { max: { ... }, min: { ... } }, circleRadius: 50, collisionFilter: { category: 1, group: 0, mask: 4294967295 }, constraintImpulse: { angle: 0, x: 0, y: 0 }, density: 0.001, force: { x: 0, y: 0 }, friction: 0.1, frictionAir: 0.01, frictionStatic: 0.5, id: 6, inertia: 38511.973373149114, inverseInertia: 0.000025965950648927504, inverseMass: 0.128571975931718, isSensor: false, isSleeping: false, isStatic: false, label: "Circle Body", mass: 7.7777446659999985, motion: 0, parent: [circular object Object], parts: [[circular object Object]], plugin: { ... }, position: { x: 260, y: 60 }, positionImpulse: { x: 0, y: 0 }, positionPrev: { x: 260, y: 60 }, render: { fillStyle: "#ffffff00", lineWidth: 0, opacity: 1, sprite: { ... }, strokeStyle: "#000", visible: true }, restitution: 0.4, sleepCounter: 0, sleepThreshold: 60, slop: 0.05, speed: 0, timeScale: 1, torque: 0, totalContacts: 0, type: "body", url: "http://www.example.com", velocity: { x: 0, y: 0 }, vertices: [{ body: [circular object Object], index: 0, isInternal: false, x: 309.635, y: 66.027 }, { body: [circular object Object], index: 1, isInternal: false, x: 306.751, y: 77.73 }, { body: [circular object Object], index: 2, isInternal: false, x: 301.149, y: 88.40299999999999 }, { body: [circular object Object], index: 3, isInternal: false, x: 293.156, y: 97.426 }, { body: [circular object Object], index: 4, isInternal: false, x: 283.236, y: 104.273 }, { body: [circular object Object], index: 5, isInternal: false, x: 271.966, y: 108.547 }, { body: [circular object Object], index: 6, isInternal: false, x: 260, y: 110 }, { body: [circular object Object], index: 7, isInternal: false, x: 248.034, y: 108.547 }, { body: [circular object Object], index: 8, isInternal: false, x: 236.764, y: 104.273 }, { body: [circular object Object], index: 9, isInternal: false, x: 226.844, y: 97.426 }, { body: [circular object Object], index: 10, isInternal: false, x: 218.851, y: 88.40299999999999 }, { body: [circular object Object], index: 11, isInternal: false, x: 213.249, y: 77.73 }, { body: [circular object Object], index: 12, isInternal: false, x: 210.365, y: 66.027 }, { body: [circular object Object], index: 13, isInternal: false, x: 210.365, y: 53.973 }, { body: [circular object Object], index: 14, isInternal: false, x: 213.249, y: 42.269999999999996 }, { body: [circular object Object], index: 15, isInternal: false, x: 218.851, y: 31.597 }, { body: [circular object Object], index: 16, isInternal: false, x: 226.844, y: 22.573999999999998 }, { body: [circular object Object], index: 17, isInternal: false, x: 236.764, y: 15.726999999999997 }, { body: [circular object Object], index: 18, isInternal: false, x: 248.034, y: 11.453000000000003 }, { body: [circular object Object], index: 19, isInternal: false, x: 260, y: 10 }, { body: [circular object Object], index: 20, isInternal: false, x: 271.966, y: 11.453000000000003 }, { body: [circular object Object], index: 21, isInternal: false, x: 283.236, y: 15.726999999999997 }, { body: [circular object Object], index: 22, isInternal: false, x: 293.156, y: 22.573999999999998 }, { body: [circular object Object], index: 23, isInternal: false, x: 301.149, y: 31.597 }, { body: [circular object Object], index: 24, isInternal: false, x: 306.751, y: 42.269999999999996 }, { body: [circular object Object], index: 25, isInternal: false, x: 309.635, y: 53.973 }] }, { angle: 0, anglePrev: 0, angularSpeed: 0, angularVelocity: 0, area: 7777.744665999999, axes: [{ x: -0.970952042258738, y: -0.239274176696078 }, { x: -0.8854431390332113, y: -0.46474772461951164 }, { x: -0.748538767034939, y: -0.6630910301352396 }, { x: -0.5680489228149688, y: -0.8229947881297631 }, { x: -0.35459420851145496, y: -0.9350202924483163 }, { x: -0.12054195746334154, y: -0.992708233314757 }, { x: 0.12054195746334154, y: -0.992708233314757 }, { x: 0.35459420851145496, y: -0.9350202924483163 }, { x: 0.5680489228149688, y: -0.8229947881297631 }, { x: 0.748538767034939, y: -0.6630910301352396 }, { x: 0.8854431390332113, y: -0.46474772461951164 }, { x: 0.970952042258738, y: -0.239274176696078 }, { x: 1, y: 0 }], bounds: { max: { ... }, min: { ... } }, circleRadius: 50, collisionFilter: { category: 1, group: 0, mask: 4294967295 }, constraintImpulse: { angle: 0, x: 0, y: 0 }, density: 0.001, force: { x: 0, y: 0 }, friction: 0.1, frictionAir: 0.01, frictionStatic: 0.5, id: 7, inertia: 38511.973373149114, inverseInertia: 0.000025965950648927504, inverseMass: 0.128571975931718, isSensor: false, isSleeping: false, isStatic: false, label: "Circle Body", mass: 7.7777446659999985, motion: 0, parent: [circular object Object], parts: [[circular object Object]], plugin: { ... }, position: { x: 31, y: 198 }, positionImpulse: { x: 0, y: 0 }, positionPrev: { x: 31, y: 198 }, render: { fillStyle: "#ffffff00", lineWidth: 0, opacity: 1, sprite: { ... }, strokeStyle: "#000", visible: true }, restitution: 0.4, sleepCounter: 0, sleepThreshold: 60, slop: 0.05, speed: 0, timeScale: 1, torque: 0, totalContacts: 0, type: "body", url: "http://www.example.com", velocity: { x: 0, y: 0 }, vertices: [{ body: [circular object Object], index: 0, isInternal: false, x: 80.63499999999999, y: 204.027 }, { body: [circular object Object], index: 1, isInternal: false, x: 77.751, y: 215.73 }, { body: [circular object Object], index: 2, isInternal: false, x: 72.149, y: 226.403 }, { body: [circular object Object], index: 3, isInternal: false, x: 64.156, y: 235.426 }, { body: [circular object Object], index: 4, isInternal: false, x: 54.236000000000004, y: 242.273 }, { body: [circular object Object], index: 5, isInternal: false, x: 42.966, y: 246.547 }, { body: [circular object Object], index: 6, isInternal: false, x: 31, y: 248 }, { body: [circular object Object], index: 7, isInternal: false, x: 19.034, y: 246.547 }, { body: [circular object Object], index: 8, isInternal: false, x: 7.763999999999999, y: 242.273 }, { body: [circular object Object], index: 9, isInternal: false, x: -2.155999999999999, y: 235.426 }, { body: [circular object Object], index: 10, isInternal: false, x: -10.149000000000001, y: 226.403 }, { body: [circular object Object], index: 11, isInternal: false, x: -15.750999999999998, y: 215.73 }, { body: [circular object Object], index: 12, isInternal: false, x: -18.634999999999998, y: 204.027 }, { body: [circular object Object], index: 13, isInternal: false, x: -18.634999999999998, y: 191.973 }, { body: [circular object Object], index: 14, isInternal: false, x: -15.750999999999998, y: 180.27 }, { body: [circular object Object], index: 15, isInternal: false, x: -10.149000000000001, y: 169.597 }, { body: [circular object Object], index: 16, isInternal: false, x: -2.155999999999999, y: 160.574 }, { body: [circular object Object], index: 17, isInternal: false, x: 7.763999999999999, y: 153.727 }, { body: [circular object Object], index: 18, isInternal: false, x: 19.034, y: 149.453 }, { body: [circular object Object], index: 19, isInternal: false, x: 31, y: 148 }, { body: [circular object Object], index: 20, isInternal: false, x: 42.966, y: 149.453 }, { body: [circular object Object], index: 21, isInternal: false, x: 54.236000000000004, y: 153.727 }, { body: [circular object Object], index: 22, isInternal: false, x: 64.156, y: 160.574 }, { body: [circular object Object], index: 23, isInternal: false, x: 72.149, y: 169.597 }, { body: [circular object Object], index: 24, isInternal: false, x: 77.751, y: 180.27 }, { body: [circular object Object], index: 25, isInternal: false, x: 80.63499999999999, y: 191.973 }] }, { angle: 0, anglePrev: 0, angularSpeed: 0, angularVelocity: 0, area: 7777.744665999999, axes: [{ x: -0.970952042258738, y: -0.239274176696078 }, { x: -0.8854431390332113, y: -0.46474772461951164 }, { x: -0.748538767034939, y: -0.6630910301352396 }, { x: -0.5680489228149688, y: -0.8229947881297631 }, { x: -0.35459420851145496, y: -0.9350202924483163 }, { x: -0.12054195746334154, y: -0.992708233314757 }, { x: 0.12054195746334154, y: -0.992708233314757 }, { x: 0.35459420851145496, y: -0.9350202924483163 }, { x: 0.5680489228149688, y: -0.8229947881297631 }, { x: 0.748538767034939, y: -0.6630910301352396 }, { x: 0.8854431390332113, y: -0.46474772461951164 }, { x: 0.970952042258738, y: -0.239274176696078 }, { x: 1, y: 0 }], bounds: { max: { ... }, min: { ... } }, circleRadius: 50, collisionFilter: { category: 1, group: 0, mask: 4294967295 }, constraintImpulse: { angle: 0, x: 0, y: 0 }, density: 0.001, force: { x: 0, y: 0 }, friction: 0.1, frictionAir: 0.01, frictionStatic: 0.5, id: 8, inertia: 38511.973373149114, inverseInertia: 0.000025965950648927504, inverseMass: 0.128571975931718, isSensor: false, isSleeping: false, isStatic: false, label: "Circle Body", mass: 7.7777446659999985, motion: 0, parent: [circular object Object], parts: [[circular object Object]], plugin: { ... }, position: { x: 247, y: 15 }, positionImpulse: { x: 0, y: 0 }, positionPrev: { x: 247, y: 15 }, render: { fillStyle: "#ffffff00", lineWidth: 0, opacity: 1, sprite: { ... }, strokeStyle: "#000", visible: true }, restitution: 0.4, sleepCounter: 0, sleepThreshold: 60, slop: 0.05, speed: 0, timeScale: 1, torque: 0, totalContacts: 0, type: "body", url: "http://www.example.com", velocity: { x: 0, y: 0 }, vertices: [{ body: [circular object Object], index: 0, isInternal: false, x: 296.635, y: 21.027 }, { body: [circular object Object], index: 1, isInternal: false, x: 293.751, y: 32.730000000000004 }, { body: [circular object Object], index: 2, isInternal: false, x: 288.149, y: 43.403 }, { body: [circular object Object], index: 3, isInternal: false, x: 280.156, y: 52.426 }, { body: [circular object Object], index: 4, isInternal: false, x: 270.236, y: 59.273 }, { body: [circular object Object], index: 5, isInternal: false, x: 258.966, y: 63.547 }, { body: [circular object Object], index: 6, isInternal: false, x: 247, y: 65 }, { body: [circular object Object], index: 7, isInternal: false, x: 235.034, y: 63.547 }, { body: [circular object Object], index: 8, isInternal: false, x: 223.764, y: 59.273 }, { body: [circular object Object], index: 9, isInternal: false, x: 213.844, y: 52.426 }, { body: [circular object Object], index: 10, isInternal: false, x: 205.851, y: 43.403 }, { body: [circular object Object], index: 11, isInternal: false, x: 200.249, y: 32.730000000000004 }, { body: [circular object Object], index: 12, isInternal: false, x: 197.365, y: 21.027 }, { body: [circular object Object], index: 13, isInternal: false, x: 197.365, y: 8.973 }, { body: [circular object Object], index: 14, isInternal: false, x: 200.249, y: -2.7300000000000004 }, { body: [circular object Object], index: 15, isInternal: false, x: 205.851, y: -13.402999999999999 }, { body: [circular object Object], index: 16, isInternal: false, x: 213.844, y: -22.426000000000002 }, { body: [circular object Object], index: 17, isInternal: false, x: 223.764, y: -29.273000000000003 }, { body: [circular object Object], index: 18, isInternal: false, x: 235.034, y: -33.547 }, { body: [circular object Object], index: 19, isInternal: false, x: 247, y: -35 }, { body: [circular object Object], index: 20, isInternal: false, x: 258.966, y: -33.547 }, { body: [circular object Object], index: 21, isInternal: false, x: 270.236, y: -29.273000000000003 }, { body: [circular object Object], index: 22, isInternal: false, x: 280.156, y: -22.426000000000002 }, { body: [circular object Object], index: 23, isInternal: false, x: 288.149, y: -13.402999999999999 }, { body: [circular object Object], index: 24, isInternal: false, x: 293.751, y: -2.7300000000000004 }, { body: [circular object Object], index: 25, isInternal: false, x: 296.635, y: 8.973 }] }, { angle: 0, anglePrev: 0, angularSpeed: 0, angularVelocity: 0, area: 7777.744665999999, axes: [{ x: -0.970952042258738, y: -0.239274176696078 }, { x: -0.8854431390332113, y: -0.46474772461951164 }, { x: -0.748538767034939, y: -0.6630910301352396 }, { x: -0.5680489228149688, y: -0.8229947881297631 }, { x: -0.35459420851145496, y: -0.9350202924483163 }, { x: -0.12054195746334154, y: -0.992708233314757 }, { x: 0.12054195746334154, y: -0.992708233314757 }, { x: 0.35459420851145496, y: -0.9350202924483163 }, { x: 0.5680489228149688, y: -0.8229947881297631 }, { x: 0.748538767034939, y: -0.6630910301352396 }, { x: 0.8854431390332113, y: -0.46474772461951164 }, { x: 0.970952042258738, y: -0.239274176696078 }, { x: 1, y: 0 }], bounds: { max: { ... }, min: { ... } }, circleRadius: 50, collisionFilter: { category: 1, group: 0, mask: 4294967295 }, constraintImpulse: { angle: 0, x: 0, y: 0 }, density: 0.001, force: { x: 0, y: 0 }, friction: 0.1, frictionAir: 0.01, frictionStatic: 0.5, id: 9, inertia: 38511.973373149114, inverseInertia: 0.000025965950648927504, inverseMass: 0.128571975931718, isSensor: false, isSleeping: false, isStatic: false, label: "Circle Body", mass: 7.7777446659999985, motion: 0, parent: [circular object Object], parts: [[circular object Object]], plugin: { ... }, position: { x: 258, y: 0 }, positionImpulse: { x: 0, y: 0 }, positionPrev: { x: 258, y: 0 }, render: { fillStyle: "#ffffff00", lineWidth: 0, opacity: 1, sprite: { ... }, strokeStyle: "#000", visible: true }, restitution: 0.4, sleepCounter: 0, sleepThreshold: 60, slop: 0.05, speed: 0, timeScale: 1, torque: 0, totalContacts: 0, type: "body", url: "http://www.example.com", velocity: { x: 0, y: 0 }, vertices: [{ body: [circular object Object], index: 0, isInternal: false, x: 307.635, y: 6.027000000000001 }, { body: [circular object Object], index: 1, isInternal: false, x: 304.751, y: 17.73 }, { body: [circular object Object], index: 2, isInternal: false, x: 299.149, y: 28.403 }, { body: [circular object Object], index: 3, isInternal: false, x: 291.156, y: 37.426 }, { body: [circular object Object], index: 4, isInternal: false, x: 281.236, y: 44.273 }, { body: [circular object Object], index: 5, isInternal: false, x: 269.966, y: 48.547 }, { body: [circular object Object], index: 6, isInternal: false, x: 258, y: 50 }, { body: [circular object Object], index: 7, isInternal: false, x: 246.034, y: 48.547 }, { body: [circular object Object], index: 8, isInternal: false, x: 234.764, y: 44.273 }, { body: [circular object Object], index: 9, isInternal: false, x: 224.844, y: 37.426 }, { body: [circular object Object], index: 10, isInternal: false, x: 216.851, y: 28.403 }, { body: [circular object Object], index: 11, isInternal: false, x: 211.249, y: 17.73 }, { body: [circular object Object], index: 12, isInternal: false, x: 208.365, y: 6.027000000000001 }, { body: [circular object Object], index: 13, isInternal: false, x: 208.365, y: -6.026999999999999 }, { body: [circular object Object], index: 14, isInternal: false, x: 211.249, y: -17.73 }, { body: [circular object Object], index: 15, isInternal: false, x: 216.851, y: -28.403 }, { body: [circular object Object], index: 16, isInternal: false, x: 224.844, y: -37.426 }, { body: [circular object Object], index: 17, isInternal: false, x: 234.764, y: -44.273 }, { body: [circular object Object], index: 18, isInternal: false, x: 246.034, y: -48.547 }, { body: [circular object Object], index: 19, isInternal: false, x: 258, y: -50 }, { body: [circular object Object], index: 20, isInternal: false, x: 269.966, y: -48.547 }, { body: [circular object Object], index: 21, isInternal: false, x: 281.236, y: -44.273 }, { body: [circular object Object], index: 22, isInternal: false, x: 291.156, y: -37.426 }, { body: [circular object Object], index: 23, isInternal: false, x: 299.149, y: -28.403 }, { body: [circular object Object], index: 24, isInternal: false, x: 304.751, y: -17.73 }, { body: [circular object Object], index: 25, isInternal: false, x: 307.635, y: -6.026999999999999 }] }, { angle: 0, anglePrev: 0, angularSpeed: 0, angularVelocity: 0, area: 7777.744665999999, axes: [{ x: -0.970952042258738, y: -0.239274176696078 }, { x: -0.8854431390332113, y: -0.46474772461951164 }, { x: -0.748538767034939, y: -0.6630910301352396 }, { x: -0.5680489228149688, y: -0.8229947881297631 }, { x: -0.35459420851145496, y: -0.9350202924483163 }, { x: -0.12054195746334154, y: -0.992708233314757 }, { x: 0.12054195746334154, y: -0.992708233314757 }, { x: 0.35459420851145496, y: -0.9350202924483163 }, { x: 0.5680489228149688, y: -0.8229947881297631 }, { x: 0.748538767034939, y: -0.6630910301352396 }, { x: 0.8854431390332113, y: -0.46474772461951164 }, { x: 0.970952042258738, y: -0.239274176696078 }, { x: 1, y: 0 }], bounds: { max: { ... }, min: { ... } }, circleRadius: 50, collisionFilter: { category: 1, group: 0, mask: 4294967295 }, constraintImpulse: { angle: 0, x: 0, y: 0 }, density: 0.001, force: { x: 0, y: 0 }, friction: 0.1, frictionAir: 0.01, frictionStatic: 0.5, id: 10, inertia: 38511.973373149114, inverseInertia: 0.000025965950648927504, inverseMass: 0.128571975931718, isSensor: false, isSleeping: false, isStatic: false, label: "Circle Body", mass: 7.7777446659999985, motion: 0, parent: [circular object Object], parts: [[circular object Object]], plugin: { ... }, position: { x: 38, y: 19 }, positionImpulse: { x: 0, y: 0 }, positionPrev: { x: 38, y: 19 }, render: { fillStyle: "#ffffff00", lineWidth: 0, opacity: 1, sprite: { ... }, strokeStyle: "#000", visible: true }, restitution: 0.4, sleepCounter: 0, sleepThreshold: 60, slop: 0.05, speed: 0, timeScale: 1, torque: 0, totalContacts: 0, type: "body", url: "http://www.example.com", velocity: { x: 0, y: 0 }, vertices: [{ body: [circular object Object], index: 0, isInternal: false, x: 87.63499999999999, y: 25.027 }, { body: [circular object Object], index: 1, isInternal: false, x: 84.751, y: 36.730000000000004 }, { body: [circular object Object], index: 2, isInternal: false, x: 79.149, y: 47.403 }, { body: [circular object Object], index: 3, isInternal: false, x: 71.156, y: 56.426 }, { body: [circular object Object], index: 4, isInternal: false, x: 61.236000000000004, y: 63.273 }, { body: [circular object Object], index: 5, isInternal: false, x: 49.966, y: 67.547 }, { body: [circular object Object], index: 6, isInternal: false, x: 38, y: 69 }, { body: [circular object Object], index: 7, isInternal: false, x: 26.034, y: 67.547 }, { body: [circular object Object], index: 8, isInternal: false, x: 14.764, y: 63.273 }, { body: [circular object Object], index: 9, isInternal: false, x: 4.844000000000001, y: 56.426 }, { body: [circular object Object], index: 10, isInternal: false, x: -3.149000000000001, y: 47.403 }, { body: [circular object Object], index: 11, isInternal: false, x: -8.750999999999998, y: 36.730000000000004 }, { body: [circular object Object], index: 12, isInternal: false, x: -11.634999999999998, y: 25.027 }, { body: [circular object Object], index: 13, isInternal: false, x: -11.634999999999998, y: 12.973 }, { body: [circular object Object], index: 14, isInternal: false, x: -8.750999999999998, y: 1.2699999999999996 }, { body: [circular object Object], index: 15, isInternal: false, x: -3.149000000000001, y: -9.402999999999999 }, { body: [circular object Object], index: 16, isInternal: false, x: 4.844000000000001, y: -18.426000000000002 }, { body: [circular object Object], index: 17, isInternal: false, x: 14.764, y: -25.273000000000003 }, { body: [circular object Object], index: 18, isInternal: false, x: 26.034, y: -29.546999999999997 }, { body: [circular object Object], index: 19, isInternal: false, x: 38, y: -31 }, { body: [circular object Object], index: 20, isInternal: false, x: 49.966, y: -29.546999999999997 }, { body: [circular object Object], index: 21, isInternal: false, x: 61.236000000000004, y: -25.273000000000003 }, { body: [circular object Object], index: 22, isInternal: false, x: 71.156, y: -18.426000000000002 }, { body: [circular object Object], index: 23, isInternal: false, x: 79.149, y: -9.402999999999999 }, { body: [circular object Object], index: 24, isInternal: false, x: 84.751, y: 1.2699999999999996 }, { body: [circular object Object], index: 25, isInternal: false, x: 87.63499999999999, y: 12.973 }] }, { angle: 0, anglePrev: 0, angularSpeed: 0, angularVelocity: 0, area: 7777.744665999999, axes: [{ x: -0.970952042258738, y: -0.239274176696078 }, { x: -0.8854431390332113, y: -0.46474772461951164 }, { x: -0.748538767034939, y: -0.6630910301352396 }, { x: -0.5680489228149688, y: -0.8229947881297631 }, { x: -0.35459420851145496, y: -0.9350202924483163 }, { x: -0.12054195746334154, y: -0.992708233314757 }, { x: 0.12054195746334154, y: -0.992708233314757 }, { x: 0.35459420851145496, y: -0.9350202924483163 }, { x: 0.5680489228149688, y: -0.8229947881297631 }, { x: 0.748538767034939, y: -0.6630910301352396 }, { x: 0.8854431390332113, y: -0.46474772461951164 }, { x: 0.970952042258738, y: -0.239274176696078 }, { x: 1, y: 0 }], bounds: { max: { ... }, min: { ... } }, circleRadius: 50, collisionFilter: { category: 1, group: 0, mask: 4294967295 }, constraintImpulse: { angle: 0, x: 0, y: 0 }, density: 0.001, force: { x: 0, y: 0 }, friction: 0.1, frictionAir: 0.01, frictionStatic: 0.5, id: 11, inertia: 38511.973373149114, inverseInertia: 0.000025965950648927504, inverseMass: 0.128571975931718, isSensor: false, isSleeping: false, isStatic: false, label: "Circle Body", mass: 7.7777446659999985, motion: 0, parent: [circular object Object], parts: [[circular object Object]], plugin: { ... }, position: { x: 261, y: 121 }, positionImpulse: { x: 0, y: 0 }, positionPrev: { x: 261, y: 121 }, render: { fillStyle: "#ffffff00", lineWidth: 0, opacity: 1, sprite: { ... }, strokeStyle: "#000", visible: true }, restitution: 0.4, sleepCounter: 0, sleepThreshold: 60, slop: 0.05, speed: 0, timeScale: 1, torque: 0, totalContacts: 0, type: "body", url: "http://www.example.com", velocity: { x: 0, y: 0 }, vertices: [{ body: [circular object Object], index: 0, isInternal: false, x: 310.635, y: 127.027 }, { body: [circular object Object], index: 1, isInternal: false, x: 307.751, y: 138.73 }, { body: [circular object Object], index: 2, isInternal: false, x: 302.149, y: 149.403 }, { body: [circular object Object], index: 3, isInternal: false, x: 294.156, y: 158.426 }, { body: [circular object Object], index: 4, isInternal: false, x: 284.236, y: 165.273 }, { body: [circular object Object], index: 5, isInternal: false, x: 272.966, y: 169.547 }, { body: [circular object Object], index: 6, isInternal: false, x: 261, y: 171 }, { body: [circular object Object], index: 7, isInternal: false, x: 249.034, y: 169.547 }, { body: [circular object Object], index: 8, isInternal: false, x: 237.764, y: 165.273 }, { body: [circular object Object], index: 9, isInternal: false, x: 227.844, y: 158.426 }, { body: [circular object Object], index: 10, isInternal: false, x: 219.851, y: 149.403 }, { body: [circular object Object], index: 11, isInternal: false, x: 214.249, y: 138.73 }, { body: [circular object Object], index: 12, isInternal: false, x: 211.365, y: 127.027 }, { body: [circular object Object], index: 13, isInternal: false, x: 211.365, y: 114.973 }, { body: [circular object Object], index: 14, isInternal: false, x: 214.249, y: 103.27 }, { body: [circular object Object], index: 15, isInternal: false, x: 219.851, y: 92.59700000000001 }, { body: [circular object Object], index: 16, isInternal: false, x: 227.844, y: 83.574 }, { body: [circular object Object], index: 17, isInternal: false, x: 237.764, y: 76.727 }, { body: [circular object Object], index: 18, isInternal: false, x: 249.034, y: 72.453 }, { body: [circular object Object], index: 19, isInternal: false, x: 261, y: 71 }, { body: [circular object Object], index: 20, isInternal: false, x: 272.966, y: 72.453 }, { body: [circular object Object], index: 21, isInternal: false, x: 284.236, y: 76.727 }, { body: [circular object Object], index: 22, isInternal: false, x: 294.156, y: 83.574 }, { body: [circular object Object], index: 23, isInternal: false, x: 302.149, y: 92.59700000000001 }, { body: [circular object Object], index: 24, isInternal: false, x: 307.751, y: 103.27 }, { body: [circular object Object], index: 25, isInternal: false, x: 310.635, y: 114.973 }] }, { angle: 0, anglePrev: 0, angularSpeed: 0, angularVelocity: 0, area: 7777.744665999999, axes: [{ x: -0.970952042258738, y: -0.239274176696078 }, { x: -0.8854431390332113, y: -0.46474772461951164 }, { x: -0.748538767034939, y: -0.6630910301352396 }, { x: -0.5680489228149688, y: -0.8229947881297631 }, { x: -0.35459420851145496, y: -0.9350202924483163 }, { x: -0.12054195746334154, y: -0.992708233314757 }, { x: 0.12054195746334154, y: -0.992708233314757 }, { x: 0.35459420851145496, y: -0.9350202924483163 }, { x: 0.5680489228149688, y: -0.8229947881297631 }, { x: 0.748538767034939, y: -0.6630910301352396 }, { x: 0.8854431390332113, y: -0.46474772461951164 }, { x: 0.970952042258738, y: -0.239274176696078 }, { x: 1, y: 0 }], bounds: { max: { ... }, min: { ... } }, circleRadius: 50, collisionFilter: { category: 1, group: 0, mask: 4294967295 }, constraintImpulse: { angle: 0, x: 0, y: 0 }, density: 0.001, force: { x: 0, y: 0 }, friction: 0.1, frictionAir: 0.01, frictionStatic: 0.5, id: 12, inertia: 38511.973373149114, inverseInertia: 0.000025965950648927504, inverseMass: 0.128571975931718, isSensor: false, isSleeping: false, isStatic: false, label: "Circle Body", mass: 7.7777446659999985, motion: 0, parent: [circular object Object], parts: [[circular object Object]], plugin: { ... }, position: { x: 148, y: 68 }, positionImpulse: { x: 0, y: 0 }, positionPrev: { x: 148, y: 68 }, render: { fillStyle: "#ffffff00", lineWidth: 0, opacity: 1, sprite: { ... }, strokeStyle: "#000", visible: true }, restitution: 0.4, sleepCounter: 0, sleepThreshold: 60, slop: 0.05, speed: 0, timeScale: 1, torque: 0, totalContacts: 0, type: "body", url: "http://www.example.com", velocity: { x: 0, y: 0 }, vertices: [{ body: [circular object Object], index: 0, isInternal: false, x: 197.635, y: 74.027 }, { body: [circular object Object], index: 1, isInternal: false, x: 194.751, y: 85.73 }, { body: [circular object Object], index: 2, isInternal: false, x: 189.149, y: 96.40299999999999 }, { body: [circular object Object], index: 3, isInternal: false, x: 181.156, y: 105.426 }, { body: [circular object Object], index: 4, isInternal: false, x: 171.236, y: 112.273 }, { body: [circular object Object], index: 5, isInternal: false, x: 159.966, y: 116.547 }, { body: [circular object Object], index: 6, isInternal: false, x: 148, y: 118 }, { body: [circular object Object], index: 7, isInternal: false, x: 136.034, y: 116.547 }, { body: [circular object Object], index: 8, isInternal: false, x: 124.764, y: 112.273 }, { body: [circular object Object], index: 9, isInternal: false, x: 114.844, y: 105.426 }, { body: [circular object Object], index: 10, isInternal: false, x: 106.851, y: 96.40299999999999 }, { body: [circular object Object], index: 11, isInternal: false, x: 101.249, y: 85.73 }, { body: [circular object Object], index: 12, isInternal: false, x: 98.36500000000001, y: 74.027 }, { body: [circular object Object], index: 13, isInternal: false, x: 98.36500000000001, y: 61.973 }, { body: [circular object Object], index: 14, isInternal: false, x: 101.249, y: 50.269999999999996 }, { body: [circular object Object], index: 15, isInternal: false, x: 106.851, y: 39.597 }, { body: [circular object Object], index: 16, isInternal: false, x: 114.844, y: 30.573999999999998 }, { body: [circular object Object], index: 17, isInternal: false, x: 124.764, y: 23.726999999999997 }, { body: [circular object Object], index: 18, isInternal: false, x: 136.034, y: 19.453000000000003 }, { body: [circular object Object], index: 19, isInternal: false, x: 148, y: 18 }, { body: [circular object Object], index: 20, isInternal: false, x: 159.966, y: 19.453000000000003 }, { body: [circular object Object], index: 21, isInternal: false, x: 171.236, y: 23.726999999999997 }, { body: [circular object Object], index: 22, isInternal: false, x: 181.156, y: 30.573999999999998 }, { body: [circular object Object], index: 23, isInternal: false, x: 189.149, y: 39.597 }, { body: [circular object Object], index: 24, isInternal: false, x: 194.751, y: 50.269999999999996 }, { body: [circular object Object], index: 25, isInternal: false, x: 197.635, y: 61.973 }] }, { angle: 0, anglePrev: 0, angularSpeed: 0, angularVelocity: 0, area: 7777.744665999999, axes: [{ x: -0.970952042258738, y: -0.239274176696078 }, { x: -0.8854431390332113, y: -0.46474772461951164 }, { x: -0.748538767034939, y: -0.6630910301352396 }, { x: -0.5680489228149688, y: -0.8229947881297631 }, { x: -0.35459420851145496, y: -0.9350202924483163 }, { x: -0.12054195746334154, y: -0.992708233314757 }, { x: 0.12054195746334154, y: -0.992708233314757 }, { x: 0.35459420851145496, y: -0.9350202924483163 }, { x: 0.5680489228149688, y: -0.8229947881297631 }, { x: 0.748538767034939, y: -0.6630910301352396 }, { x: 0.8854431390332113, y: -0.46474772461951164 }, { x: 0.970952042258738, y: -0.239274176696078 }, { x: 1, y: 0 }], bounds: { max: { ... }, min: { ... } }, circleRadius: 50, collisionFilter: { category: 1, group: 0, mask: 4294967295 }, constraintImpulse: { angle: 0, x: 0, y: 0 }, density: 0.001, force: { x: 0, y: 0 }, friction: 0.1, frictionAir: 0.01, frictionStatic: 0.5, id: 13, inertia: 38511.973373149114, inverseInertia: 0.000025965950648927504, inverseMass: 0.128571975931718, isSensor: false, isSleeping: false, isStatic: false, label: "Circle Body", mass: 7.7777446659999985, motion: 0, parent: [circular object Object], parts: [[circular object Object]], plugin: { ... }, position: { x: 40, y: 189 }, positionImpulse: { x: 0, y: 0 }, positionPrev: { x: 40, y: 189 }, render: { fillStyle: "#ffffff00", lineWidth: 0, opacity: 1, sprite: { ... }, strokeStyle: "#000", visible: true }, restitution: 0.4, sleepCounter: 0, sleepThreshold: 60, slop: 0.05, speed: 0, timeScale: 1, torque: 0, totalContacts: 0, type: "body", url: "http://www.example.com", velocity: { x: 0, y: 0 }, vertices: [{ body: [circular object Object], index: 0, isInternal: false, x: 89.63499999999999, y: 195.027 }, { body: [circular object Object], index: 1, isInternal: false, x: 86.751, y: 206.73 }, { body: [circular object Object], index: 2, isInternal: false, x: 81.149, y: 217.403 }, { body: [circular object Object], index: 3, isInternal: false, x: 73.156, y: 226.426 }, { body: [circular object Object], index: 4, isInternal: false, x: 63.236000000000004, y: 233.273 }, { body: [circular object Object], index: 5, isInternal: false, x: 51.966, y: 237.547 }, { body: [circular object Object], index: 6, isInternal: false, x: 40, y: 239 }, { body: [circular object Object], index: 7, isInternal: false, x: 28.034, y: 237.547 }, { body: [circular object Object], index: 8, isInternal: false, x: 16.764, y: 233.273 }, { body: [circular object Object], index: 9, isInternal: false, x: 6.844000000000001, y: 226.426 }, { body: [circular object Object], index: 10, isInternal: false, x: -1.149000000000001, y: 217.403 }, { body: [circular object Object], index: 11, isInternal: false, x: -6.750999999999998, y: 206.73 }, { body: [circular object Object], index: 12, isInternal: false, x: -9.634999999999998, y: 195.027 }, { body: [circular object Object], index: 13, isInternal: false, x: -9.634999999999998, y: 182.973 }, { body: [circular object Object], index: 14, isInternal: false, x: -6.750999999999998, y: 171.27 }, { body: [circular object Object], index: 15, isInternal: false, x: -1.149000000000001, y: 160.597 }, { body: [circular object Object], index: 16, isInternal: false, x: 6.844000000000001, y: 151.574 }, { body: [circular object Object], index: 17, isInternal: false, x: 16.764, y: 144.727 }, { body: [circular object Object], index: 18, isInternal: false, x: 28.034, y: 140.453 }, { body: [circular object Object], index: 19, isInternal: false, x: 40, y: 139 }, { body: [circular object Object], index: 20, isInternal: false, x: 51.966, y: 140.453 }, { body: [circular object Object], index: 21, isInternal: false, x: 63.236000000000004, y: 144.727 }, { body: [circular object Object], index: 22, isInternal: false, x: 73.156, y: 151.574 }, { body: [circular object Object], index: 23, isInternal: false, x: 81.149, y: 160.597 }, { body: [circular object Object], index: 24, isInternal: false, x: 86.751, y: 171.27 }, { body: [circular object Object], index: 25, isInternal: false, x: 89.63499999999999, y: 182.973 }] }]
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK