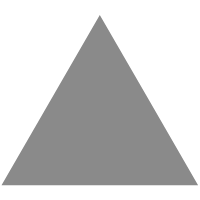
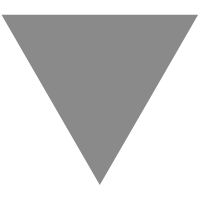
你需要知道的 7 个 Vue3 技巧
source link: https://www.fly63.com/article/detial/12251
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
VNode 钩子
在每个组件或html标签上,我们可以使用一些特殊的(文档没写的)钩子作为事件监听器。这些钩子有:
- onVnodeBeforeMount
- onVnodeMounted
- onVnodeBeforeUpdate
- onVnodeUpdated
- onVnodeBeforeUnmount
- onVnodeUnmounted
我主要是在组件上使用onVnodeMounted,当需要在组件挂载时执行一些代码,或者在更新时使用onVnodeUpdated进行调试,可以确定的是所有这些钩子都能在某些情况下派上用场。
例子:
<script setup>
import { ref } from 'vue'
const count = ref(0)
function onMyComponentMounted() {}
function divThatDisplaysCountWasUpdated() {}
</script>
<template>
<MyComponent @vnodeMounted="onMyComponentMounted" />
<div @vnodeUpdated="divThatDisplaysCountWasUpdated">{{ count }}</div>
</template>
应该注意的是,这些挂钩将一些参数传递给回调函数。它们只传递一个参数,即当前 VNode,除了onVnodeBeforeUpdate传递onVnodeUpdated两个参数,当前 VNode 和前一个 VNode。
我们都知道 Vue 为我们提供的生命周期钩子。但是您知道 Vue 3 为我们提供了两个可用于调试目的的钩子吗?他们是:
onRenderTracked为已跟踪的每个反应性依赖项调用。
<script setup>
import { ref, onRenderTracked } from 'vue'
const count = ref(0)
const count2 = ref(0)
// It will be called twice, once for count and once for count2
onRenderTracked((event) => {
console.log(event)
})
</script>
onRenderTriggered当我们触发反应性更新时被调用,或者如文档所说:“当反应性依赖触发组件的渲染效果重新运行时”。
<script setup>
import { ref, onRenderTriggered } from 'vue'
const count = ref(0)
// It will be called when we update count
onRenderTriggered((event) => {
debugger
})
</script>
从子组件公开插槽
如果您使用第三方组件,您可能会将其实现包装在您自己的“包装器”组件中。这是一个很好的实践和可扩展的解决方案,但那样的话,第三方组件的插槽就会丢失,我们应该找到一种方法将它们暴露给父组件:
WrapperComponent.vue
<template>
<div class="wrapper-of-third-party-component">
<ThirdPartyComponent v-bind="$attrs">
<!-- Expose the slots of the third-party component -->
<template v-for="(_, name) in $slots" #[name]="slotData">
<slot :name="name" v-bind="slotData || {}"></slot>
</template>
</ThirdPartyComponent>
</div>
</template>
现在每个使用的组件都WrapperComponent可以使用ThirdPartyComponent的插槽。
作用域样式和多根节点不能很好地协同工作
在 Vue 3 中,我们终于可以拥有不止“一个根节点”的组件。这很好,但我个人在这样做时遇到了设计限制。假设我们有一个子组件:
<template>
<p class="my-p">First p</p>
<p class="my-p">Second p</p>
</template>
和一个父组件:
<template>
<h1>My awesome component</h1>
<MyChildComponent />
</template>
<style scoped>
// There is no way to style the p tags of MyChildComponent
.my-p { color: red; }
:deep(.my-p) { color: red; }
</style>
无法从多根父组件的作用域样式设置子组件的 p 标签的样式。
所以简而言之,一个多根组件,不能使用作用域样式来定位多根子组件的样式。
解决这个问题的最好方法是包装父组件或子组件(或两者),这样我们就只有一个根元素。
但是如果你绝对需要两者都有多根节点,你可以:
- 使用非作用域样式
<style>
.my-p { color: red; }
</style>
- 使用 css 模块
<template>
<h1>My awesome component</h1>
<MyChildComponent :class="$style.trick" />
</template>
<style module>
.trick {
color: red;
}
</style>
既然我们在这里指定了一个类,那么多根子组件就得显式指定属性 fallthrough 行为。
如果你想要我的意见,除非你绝对需要一个多根节点组件,否则请使用单个根节点并且根本不要处理这个设计限制。
使用 CSS 选择器时要小心
#main-nav > li {}将比 . 慢很多倍.my-li { color: red }。从文档:
由于浏览器呈现各种 CSS 选择器的方式,p { color: red } 在范围内(即与属性选择器结合使用时)会慢很多倍。如果您改用类或 ID,例如在 .example { color: red } 中,那么您几乎可以消除性能损失。
如果您想更深入地研究这个主题,我强烈建议您阅读Efficiently Rendering CSS 。
在 Vue 2 或 Vue 3 的早期版本中,对于具有布尔类型的道具,我们根据顺序有不同的行为:
第一种情况:
props: {
hoverColor: [String, Boolean] // <- defaults to ''
}
第二种情况:
props: {
hoverColor: [Boolean, String] // <- defaults to false
}
不仅如此,如果你像这样传递 prop:
<my-component hover-color></my-component>
在第一种情况下,它将是一个空字符串''。在第二种情况下,它将是true.
如您所见,这有点混乱和不一致。幸运的是,在 Vue 3 中,我们有一个一致且可预测的新行为:
Boolean无论类型出现顺序如何,行为都将适用。
hoverColor: [String, Boolean] // <- defaults to false
hoverColor: [Boolean, String] // <- defaults to false
hoverColor: [Boolean, Number] // <- defaults to false
带有 v-for 的模板引用 - 不能保证顺序
记住这个,这样你就不会浪费数小时的调试时间来弄清楚发生了什么
在下面的代码中:
<script setup>
import { ref } from "vue";
const list = ref([1, 2, 3]);
const itemRefs = ref([]);
</script>
<template>
<ul>
<li v-for="item in list" ref="itemRefs" :key="item">
{{ item }}
</li>
</ul>
</template>
我们在列表数组上循环,并创建 itemRefs 数组。itemRefs不保证与列表数组有相同的顺序。如果你想了解更多这方面的信息,你可以阅读这个issue。
来源:https://dev.to/the_one/vue-3-tipstricks-i-guarantee-you-didnt-know-49ml
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK