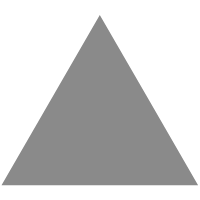
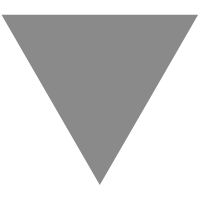
在Tomcat中启用虚拟线程特性 - throwable
source link: https://www.cnblogs.com/throwable/p/16767573.html
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
前提#
趁着国庆前后阅读了虚拟线程相关的源码,写了一篇《虚拟线程 - VirtualThread源码透视》,里面介绍了虚拟线程的实现原理和使用示例。需要准备做一下前期准备:
- 安装
OpenJDK-19
或者Oracle JDK-19
- 准备好嵌入式
Tomcat
的依赖,需要引入三个依赖包,分别是tomcat-embed-core
、tomcat-embed-el
和tomcat-embed-websocket
,版本选用10.1.0+
查看Tomcat
官方文档的CHANGELOG
:
支持Loom
项目的Tomcat
最低版本为10.1.0-M16
,对应的正式版是10.1.0
(当前时间为2022-10-07
前后),低于此版本因为大量API
还没有适配虚拟线程,主要是没有改造监视器锁的引用导致虚拟线程pin
到载体(平台)线程等问题,因此别无他选。另外,重要的提醒说三次:
- 本文是实验性质,在未完全证实改造功能可以应用生产环境前需要谨慎评估,或者先别使用于生产环境
- 本文是实验性质,在未完全证实改造功能可以应用生产环境前需要谨慎评估,或者先别使用于生产环境
- 本文是实验性质,在未完全证实改造功能可以应用生产环境前需要谨慎评估,或者先别使用于生产环境
引入依赖#
引入以下依赖:
<dependency>
<groupId>org.apache.tomcat.embed</groupId>
<artifactId>tomcat-embed-core</artifactId>
<version>10.1.0</version>
</dependency>
<dependency>
<groupId>org.apache.tomcat.embed</groupId>
<artifactId>tomcat-embed-el</artifactId>
<version>10.1.0</version>
</dependency>
<dependency>
<groupId>org.apache.tomcat.embed</groupId>
<artifactId>tomcat-embed-websocket</artifactId>
<version>10.1.0</version>
</dependency>
编程式初始化Tomcat#
为了使用反射调用一些java.base
模块下没开放的依赖包和跟踪虚拟线程栈,程序运行时候加入下面的VM
参数:
--add-opens java.base/java.lang=ALL-UNNAMED --add-opens java.base/java.lang.reflect=ALL-UNNAMED --add-opens java.base/java.util.concurrent=ALL-UNNAMED -Djdk.tracePinnedThreads=full
在IDEA
的运行配置中是这个样子:
接着编写一个HttpServlet
实现:
public class VirtualThreadHandleServlet extends HttpServlet {
private static final DateTimeFormatter FORMATTER = DateTimeFormatter.ofPattern("yyyy-MM-dd HH:mm:ss.SSS");
@Override
protected void service(HttpServletRequest req, HttpServletResponse resp) throws ServletException, IOException {
Thread thread = Thread.currentThread();
System.out.printf("service by thread ==> %s, is virtual ==> %s, carrier thread ==> %s\n",
thread.getName(), thread.isVirtual(), getCurrentCarrierThreadName(thread));
resp.setStatus(HttpServletResponse.SC_OK);
resp.setHeader("Content-Type", "application/json");
String content = "{\"time\":" + "\"" + LocalDateTime.now().format(FORMATTER) + "\"}";
resp.getWriter().write(content);
}
private static String getCurrentCarrierThreadName(Thread currentThread) {
if (currentThread.isVirtual()) {
try {
MethodHandle methodHandle = MethodHandles.privateLookupIn(Thread.class, MethodHandles.lookup())
.findStatic(Thread.class, "currentCarrierThread", MethodType.methodType(Thread.class));
Thread carrierThread = (Thread) methodHandle.invoke();
return carrierThread.getName();
} catch (Throwable e) {
e.printStackTrace();
}
}
return "UNKNOWN";
}
}
该Servlet
实现比较简单,就是在控制台打印一些虚拟线程和载体线程的一些信息,然后返回HTTP
状态码为200
和一个JSON
字符展示当前精确到毫秒的时间。接着编写一个main
方法初始化Tomcat
:
public class EmbedTomcatVirtualThreadDemo {
private static final String SERVLET_NAME = "VirtualThreadHandleServlet";
private static final String SERVLET_PATH = "/*";
/**
* 设置VM参数:
* --add-opens java.base/java.lang=ALL-UNNAMED
* --add-opens java.base/java.lang.reflect=ALL-UNNAMED
* --add-opens java.base/java.util.concurrent=ALL-UNNAMED
* -Djdk.tracePinnedThreads=full
*
* @param args args
* @throws Exception e
*/
public static void main(String[] args) throws Throwable {
String pinMode = System.getProperty("jdk.tracePinnedThreads");
System.out.println("pin mode = " + pinMode);
Tomcat tomcat = new Tomcat();
Context context = tomcat.addContext("", (new File(".")).getAbsolutePath());
Tomcat.addServlet(context, SERVLET_NAME, new VirtualThreadHandleServlet());
context.addServletMappingDecoded(SERVLET_PATH, SERVLET_NAME);
Connector connector = new Connector();
ProtocolHandler protocolHandler = connector.getProtocolHandler();
if (protocolHandler instanceof AbstractProtocol<?> protocol) {
protocol.setAddress(InetAddress.getByName("127.0.0.1"));
protocol.setPort(9091);
ThreadFactory factory = Thread.ofVirtual().name("embed-tomcat-virtualWorker-", 0).factory();
Class<?> klass = Class.forName("java.util.concurrent.ThreadPerTaskExecutor");
MethodHandle methodHandle = MethodHandles.privateLookupIn(klass, MethodHandles.lookup())
.findStatic(klass, "create", MethodType.methodType(klass, new Class[]{ThreadFactory.class}));
ExecutorService executor = (ExecutorService) methodHandle.invoke(factory);
protocol.setExecutor(executor);
}
tomcat.getService().addConnector(connector);
tomcat.start();
}
}
这里VirtualThreadHandleServlet
匹配所有格式的请求路径并且处理所有请求方法类型的请求。默认的虚拟线程调度器没有为虚拟线程设置名称,也就是如果使用Executors.newVirtualThreadPerTaskExecutor()
作为Tomcat
的线程池是最终调用看到的控制台输出的虚拟线程名称是一个空字符串。所以笔者这里用MethodHandle
直接实例化了默认修饰符没有开放访问权限的ThreadPerTaskExecutor
类,基于一个自定义的ThreadFactory
强制构造了一个自定义ThreadPerTaskExecutor
实例。调用main
方法启动后见控制台输出:
这里确认了Tomcat
启动完成侦听127.0.0.1:9091
,通过浏览器或者POSTMAN
发送任意请求例如http://127.0.0.1:9091/foo
就能看到响应结果和控制台输出:
这里的Tomcat
线程池甚至可以设计为一个完全自定义的虚拟线程调度器,可以参考前面一篇文章,这里不再赘述。
暂时无法在SpringBoot体系中使用#
由于Servlet
规范问题,Tomcat
的升级导致一些接口迁移到jakarta.servlet
包中,例如jakarta.servlet.Servlet
,此时SpringBoot
体系即使是最新版本(当前时间为2022-10-07
前后,此时最新版本为2.7.4
)使用的是还是旧的规范,对应的类是javax.servlet.Servlet
,这只是其中一个接口,大部分和HTTP
协议或者Servlet
规范相关的接口都存在这个包升级不兼容的问题,需要等待SpringBoot
升级为embed-tomcat-*-10.1.0+
才能适配虚拟线程。
小结#
Demo
项目仓库:
Github
:https://github.com/zjcscut/framework-mesh/tree/master/tomcat-virtual-thread
(本文完 e-a-20221007 c-1-d)
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK