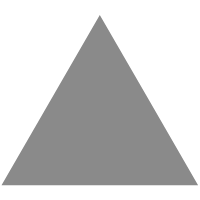
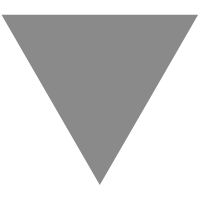
Type Challenges: Implement the Built-In Omit<T, K> Utility Type
source link: https://blog.bitsrc.io/typescript-challenge-implement-the-built-in-omit-t-k-utility-type-5e953d5c94c
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
Type Challenges: Implement the Built-In Omit<T, K> Utility Type
Master TypeScript Omit Generic, Complete Type Challenges Together, and Solidify Your TypeScript Knowledge.
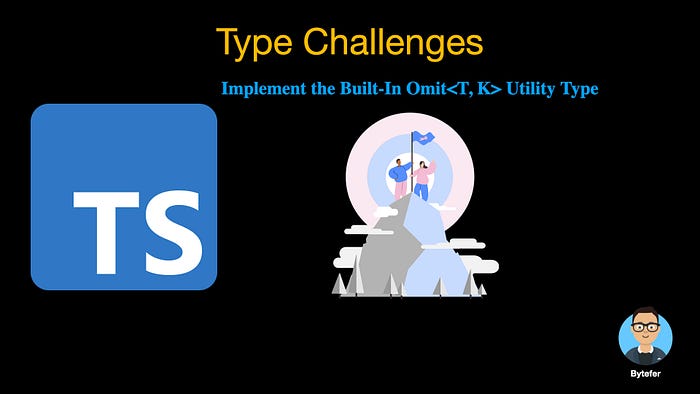
Welcome to the Mastering TypeScript series, there are dozens of articles in this series. To help readers better consolidate their knowledge of TypeScript, I have selected a few dozen challenges from the type-challenges repository on Github to complete the type challenge with you.
With These Articles, You Will Not Be Confused When Learning TypeScript
Through Vivid Animations, You Can Easily Understand the Difficult Points and Core Knowledge of TypeScript! Continuously…
Challenge
Implement the built-in Omit<T, K>
generic without using it. Constructs a type by picking all properties from T
and then removing K
.
For example:
interface Todo {
title: string
description: string
completed: boolean
}type TodoPreview = MyOmit<Todo, 'description' | 'title'>const todo: TodoPreview = {
completed: false,
}
Solution
Our type challenge is to implement the built-in Omit<Type,Keys>
generic, so let’s first understand what Omit<Type,Keys>
generic does.
Omit<Type, Keys>
Constructs a type by picking all properties from Type
and then removing Keys
(string literal or union of string literals).
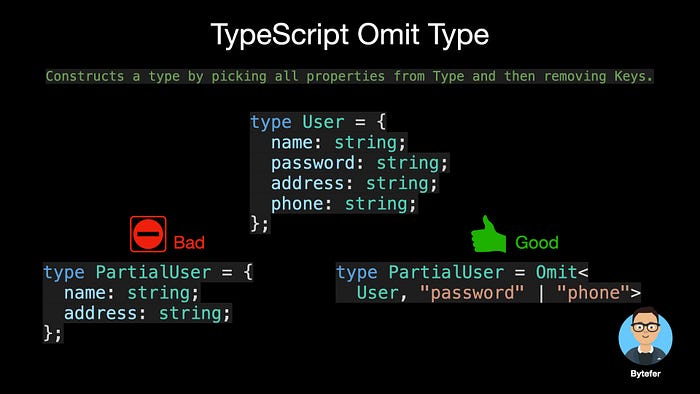
For TypeScript’s Omit
generic, its ability is to convert existing object type into new object type.
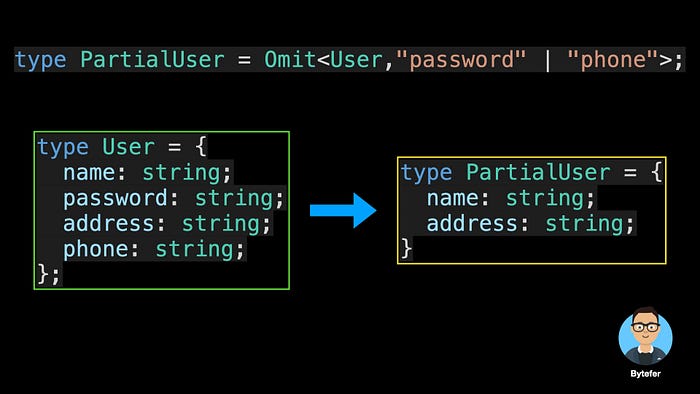
To implement the above type transformation, we need to iterate over the types excluding Keys and return the new object type. For this requirement, we need to use the mapped types provided by TypeScript.
Mapped Types
The syntax for mapped types is as follows:
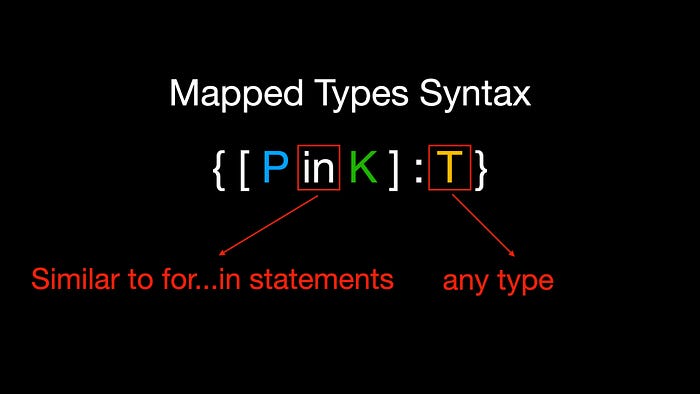
Where P in K is similar to the JavaScript for...in
statement, which is used to iterate through all types in type K, and the T type variable, which is used to represent any type in TypeScript.
After introducing the relevant knowledge, let’s define a MyOmit
generic.
type MyOmit<T, K extends keyof any> = {
[P in Exclude<keyof T, K>]: T[P]
}
In the above code, T
and K
are called type parameters. The keyof
operator is used to get all keys in a type and its return type is a union type. If keyof
operates on an object type, it functions similarly to the Object.keys
method.
And K extends keyof any
is a generic constraint, which is used to constrain that the actual type corresponding to the type parameter K
is a subtype of the union type (string | number | symbol
). T[P]
is used to obtain the type corresponding to the P attribute in the T type, where the value of the type variable P will change continuously during the traversal process.
The Exclude
generic is a built-in utility type in TypeScript, and its role is to constructs a type by excluding from UnionType
all union members that are assignable to ExcludedMembers
.
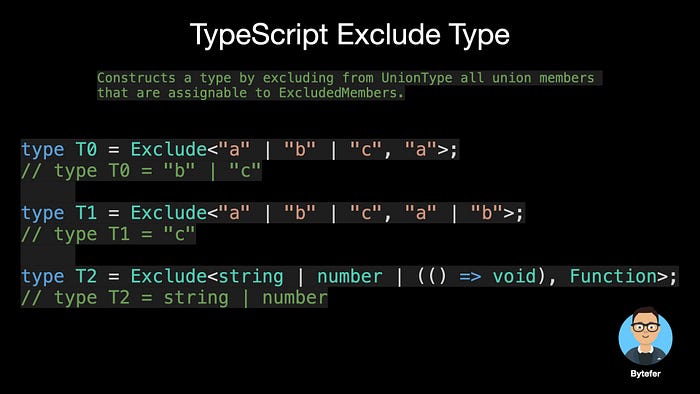
In fact, the implementation of Exclude
generic is not complicated, it uses conditional types internally.
Using TypeScript Conditional Types Like a Pro
Explained with animations. Master TypeScript Conditional Types and understand how TypeScript’s built-in Utility Types…
/**
* Exclude from T those types that are assignable to U.
* typescript/lib/lib.es5.d.ts
*/
type Exclude<T, U> = T extends U ? never : T;
To get a better grasp of Exclude
generic, let’s take a look at how it works:
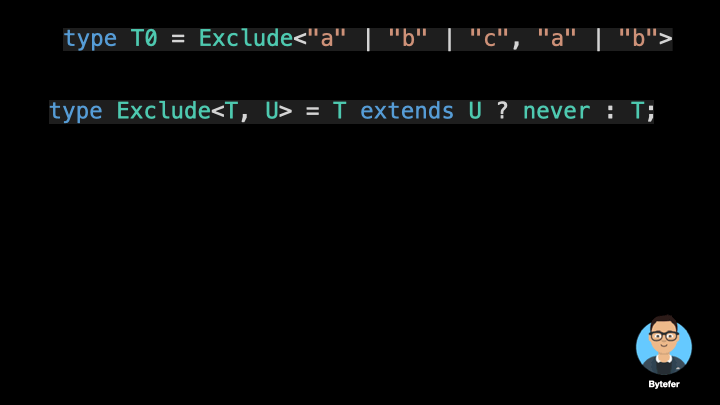
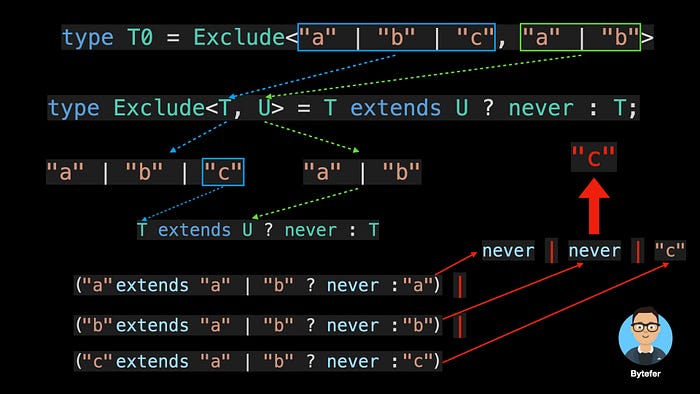
Complete code
Finally, let’s look at the complete code:
interface Todo {
title: string;
description: string;
completed: boolean;
}type MyOmit<T, K extends keyof any> = {
[P in Exclude<keyof T, K>]: T[P];
};type TodoPreview = MyOmit<Todo, "description" | "title">;
const todo: TodoPreview = {
completed: false,
};
The main knowledge involved in this challenge is TypeScript’s mapped types. If you want to learn more about mapped types, you can read the following article:
Using TypeScript Mapped Types Like a Pro
Mapped Types — Explained with animations. Master TypeScript Mapped Types and understand how TypeScript’s built-in…
In addition to the above implementation, we can also use the Pick
generic to implement the MyOmit
generic:
type MyOmit<T, K extends keyof any> = Pick<T, Exclude<keyof T, K>>;
If you encounter unclear content, you can give me a message. If you like to learn TypeScript in the form of animation, you can follow me on Medium or Twitter to read more about TS and JS!
Resources:
With These Articles, You Will Not Be Confused When Learning TypeScript
Through Vivid Animations, You Can Easily Understand the Difficult Points and Core Knowledge of TypeScript! Continuously…
Build apps with reusable components like Lego
Bit’s open-source tool help 250,000+ devs to build apps with components.
Turn any UI, feature, or page into a reusable component — and share it across your applications. It’s easier to collaborate and build faster.
Split apps into components to make app development easier, and enjoy the best experience for the workflows you want:
→ Micro-Frontends
→ Design System
→ Code-Sharing and reuse
→ Monorepo
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK