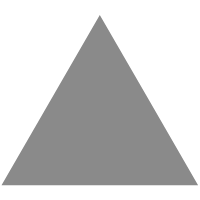
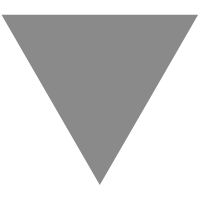
Learn React: Click Functionality and Reusable Components
source link: https://thenewstack.io/learn-react-click-functionality-and-reusable-components/
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
Learn React: Click Functionality and Reusable Components

Welcome to the fourth installment of our learning React.js tutorial series (Part 1), (Part 2) (Part 3). Here’s a link to the GitHub Repo. The Read Me has all the instructions needed to get started plus some helpful links for anyone unfamiliar with GitHub.
Since we are picking right back up where we left off, there aren’t any necessary updates needed on the code files. If you didn’t already add the submit button, that will be the first step today and it will go right in the SubmitComponent.
handleSubmit
The functionality on this one is a little more complicated than our handleChange so the function itself is a little more complicated, but not by much. The submit button is going to do perform the following duties and in this order:
- Add the string value of this.state.text to the array in this.state.tasks
- Set the value of this.state.text back to an empty string
We can keep all of this in the same handleSubmit function since we always want those actions to take place together. In the case of this project, since there are no conditions as to when we would or wouldn’t want to keep the text variable, it’s a very simple set up that doesn’t require any logic. For the purpose of this tutorial, I am not going to add any logic that will disqualify any string entries from getting added to the state.
Since we cannot push directly into state, I chose to make a copy of the current state array and replace the entire value of the state array with an array consisting of the copy followed by this.state.text. It will look something like this:
this.setState({tasks: […this.state.tasks, this.state.text]}) |
This is a great article about making shallow copies of arrays if you want more information on making copies.
The next step in our handleSubmit function is to reset this.state.text to an empty string. Last, bind the function in the constructor and it’s all set. Refresh the browser and give it a shot! The handleSubmit, function binding, and props passing to the SubmitComponent are all shown in the image below.

TaskComponent/Reusable Components
We are up and running in terms of adding tasks from the browser to state. Now it’s time to go back to the TaskComponent and start building out our reusable component.
Before we do, I want to briefly go over what a reusable component is. If you think about the Instagram feed, each image is different but the image is in a box with and that box has optional captions, you can like it, comment, share, bookmark. The UI is all the same but the data is different. That’s a reusable component.
React favors the pattern where the render logic stays in the App component and the reusable component (in this case the TaskComponent) includes the HTML elements and what they look like.
Right now, we need a place to render the text spelling out the task and a checkbox since eventually, we are going to delete the tasks from the page.
If you haven’t already, delete the commented out <h1> tags in the TaskComponent as that was placeholder text from the first iteration of this project.
Since there wasn’t any state passed to the TaskComponent yet, I’m going to add <span> tags with nothing between them to remind myself that I am going to add text there and a checkbox.

Back in the App component, we can erase the current TaskComponent element in the return section as it was just a placeholder and this next step will completely rewrite it.
React favors the pattern where the render logic stays in the App component and the reusable component (in this case the TaskComponent) includes the HTML elements and what they look like. Rather than passing {this.state.tasks} as an array to the task component, we pass each task individually to the task component but do that multiple times via a JavaScript loop or in this case, mapping through the array.
The correct place to map through the array is in the return section in the App component. I wrote my function below the SubmitComponent element. We write the whole thing inside curly braces to let the IDE know to expect JavaScript. One important thing to note — add an index to the current task as an index is needed next week when we add the delete functionality.
The image below details exactly what my map function looks like but as stated above, this isn’t the only way to do it as it can also be done with a loop.

Back in my TaskComponent I’ll make sure to add {this.props.task} between the <span> tags. Refresh the browser and you’ll now see the tasks are added directly from the text input box and onto the page.

I had a hard time understanding why state was mapped through in the App component before it was passed to the TaskComponent when I built my first project like this. I think, for me, the way it stuck was that the majority of the business logic stays together. The reusable components exist to tell React where elements go and in some cases, what they look like.
Next week we’re going to cover the delete functionality which I found to be the hardest one. I’m going to dive a little deeper into how the add functionality works, what the delete functionality is, and why they are called differently.
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK