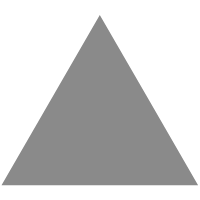
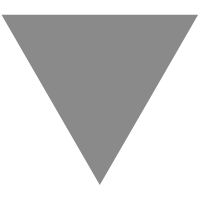
使用Typescript给JavaScript做静态类型检查
source link: https://hateonion.me/posts/aeb8/
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
TypeScript,作为JavaScript的超集,给JavaScript带来了强类型这个非常强大的特性,给前端的开发以及重构带来了很大的便利性。但是,即使TypeScript现在已经可以直接使用用JavaScript编写的模块了,有很多遗留项目想要立马迁移到TypeScript也并非易事。但是好消息是,TypeScript在2.3版本引入了Js Type Checking,从此,不需要任何对于已有代码的侵入,你也可以很好的享受TypeScript带来的一些特性了。
从迁移TypeScript说起
笔者所说的这个项目,是一个运行了接近五年的老项目,代码横跨es3到es6。而且代码风格由于项目的人员流动也各异。由于项目人数越来越多,以前留下的技术债造成的危害也越来越大。而重构,在这样一个臃肿且混乱的项目中显得举步维艰。由于JS的动态性,有的时候你甚至不知道这个方法是某个类下的实例方法还是JavaScript原生或是JQuery所提供的方法。 这个时候,TypeScript由于其提供的强类型能很好的规范代码,方便开发人员进行日常开发和重构,开始进入了我们的视野。 但是马上我们面对上了另一个难题,那就是,我们只想享受TypeScript强类型带来的类型推导的优势,并不想花大功夫去把整个代码库全部重构成TypeScript。 不过好在,TypeScript从2.3版本后就开始支持使用JsDoc的语法,以comment的形式给JavaScript文件提供强类型的支持。Type Checking JavaScript Files · TypeScript
如何开始使用Type Checking
首先你需要在你需要检查的JavaScript的文件头部显式的加上如下comment
|
对变量进行类型检查
如果你需要声名一个变量的类型,你可以这样
|
当你尝试给x赋一个bool值时,编辑器会提示你类型冲突。
对函数进行类型检查
当然某些时候我们最需要的其实是对于函数签名和返回值的强类型声名,以便我们在其他地方使用的时候不会传入错误的参数或者使用类型错误的函数返回值。TypeScript提供了三种语法来声名函数的类型.
|
在日常使用中,如果你想声明一个函数,对于函数做类型检查,你需要使用 @params
@returns
这种declaration 语法。
如果你想确定一个函数表达式的签名,你需要使用 @type
的语法。
对于 @type
语法, 从表现力上,我个人更偏好typscript like syntax
因为表达力最强。但是可惜的是这种语法在webstorm上会被认为是非法语法。
自定义类型
自定义类型有点类似于定义TypeScript中的Interface,TypeScript同样支持两种语法来自定义类型。
|
需要注意的是,第二种语法在webstorm中也不被认为只一个合法的语法。
声明一个class
对于类的property的类型,可以很轻松的进行检查
|
对于类的实例方法,你需要对于函数做出声明.
|
其他的一些特性
|
类型union
|
jsdoc的枚举和其他语言的枚举或者typescript的枚举有所不同,更多的是起到描述属性值为同一种类型的object的作用
|
内联object
|
type checking React
|
三方类型在这里既可以是 npm提供的 @types
包,也可以是自己在项目中通过TypeScript定义的类型。 只要在typeRoots这个编译器选项中指明即可。
如何和项目做集成
IDE和编辑器集成
项目以使用vscode和webstorm为主,但是理论上,只要能支持TypeScript Language Server Protocal的编辑器都不会出现集成上的问题。
vscode
对于vscode,不需要做过多的配置,直接在文件头部加入 // @ts-check
的comment就可以开启TypeScript checking.
WebStorm
对于WebStorm,你需要提供对应的tsconfig.json
文件来显式的打开这个特性。
|
工作流集成
除开IDE和编辑器等开发工具的集成之外,我们还需要把TypeScript Checking JavaScript集成进我们的构建流程之中。TypeScript的CLI提供了非常强大的功能,由于在tsconfig.json
中声名了只做类型检查,不做实际的编译,所以通过一条简单的tsc
命令就能很好的帮助我们在构建中检查我们的代码是否存在构建错误。
凡事都有两面性,Type Checking JS也并非没有缺点。在一段时间的试验后,我发现和直接编写TypeScript相比,Type Checking JS存在以下不爽的地方
1. 使用comments破坏代码语义化
比起类型声明和代码结合在一起,使用comments的形式难免在阅读和编写的时候感觉有些别扭,在代码上也有一定的冗余。
|
2. 语法支持不统一
Webstorm出于某种原因并不支持typescript like的语法,我们只能退而求其次选择closure like的语法。 这让Type Checking JS的写法下降了不止一个档次。
虽然最后聊到了Type Checking JS的一些缺陷和限制,但是这并不妨碍其成为改造老旧项目或者向TypeScript过渡的比较不错的一个方案。最后也希望社区能继续推动和改进这种写法,让更多的老旧项目尽可能多的享受TypeScript带来的各种便利的特性。
Recommend
-
62
-
44
Flow 是 Facebook 出品的,针对 JavaScript 的静态类型检查工具。它可以帮助捕获 JavaScript 开发中的常见错误,而不需要额外地修改原有的代码,比如静态类型转换,空值引用等问题。同...
-
6
Python使用Flake8做代码静态检查的时候如何忽略一些比较长的语句[E501] – Tinyfool的个人网站最近开始在Visul Studio Code里面用Flake8做我的Python项目的代码静态检查,感觉不错,确实可以提升可读性。 但是在我的测试样例里面,我发现有时候我要引入一...
-
3
静态类型在 JavaScript 中是一种负担吗? 2016-09-29 最近我在公司的项目中遇到很多由于类型不匹配所导致的不可预估的问题。比如说,我们的程序产生了一个纯数字的 unique id, 存到数据库中被识别为...
-
5
使用mypy对python程序进行静态检查 自从python3.6开始,允许为参数和返回类型添加 type hint,这使得对python程序进行静态检查成为可能。 mypy就是这么一个python type checker. 安装mypy 我们可以使用
-
3
[vscode]如何忽略 vendor 文件夹下的静态检查 V2EX = way to explore V2EX 是一个关于分享和探索的地方 V2E...
-
4
vuex 4.x TS增强更好的支持智能提示及TS检查,在不影响已有TS支持的功能情况下, 增强 state, getters 无限层级属性提示,并支持只读校验; 增强commit、dispache方法感知所有操作类型名称并对载荷参数检查,类型名称支持namespaced配置进行拼接。...
-
5
11.5K Star!一个开源的 Python 静态类型检查库发布于 54 分钟前【导语】:Python 静态类型检查库,可以发现程序中潜在的错误。Mypy 是 Python 的静态类型检...
-
0
在JavaScript中,数据类型分为两大类,一种是基础数据类型,另一种则是复杂数据类型,又叫引用数据类型基础数据类型:数字Number 字符串String 布尔Boolean Null Undefined...
-
3
V2EX › 程序员 [求助] typescript 的嵌套类型检查问题
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK