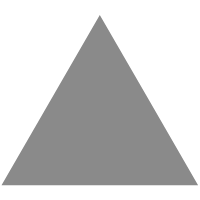
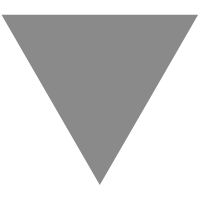
[JavaScript] Modal (Popup)
source link: http://siongui.github.io/2017/05/13/javascript-modal-popup/
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
[JavaScript] Modal (Popup)
May 13, 2017
Modal (Popup) implementation via JavaScript and CSS. According to w3schools, modal is dialog box/popup window that is displayed on top of the current page. The modal here is a simplified version of Bootstrap modal.
The following is the source code for above demo.
HTML:
<!-- Button trigger modal --> <button type="button" data-toggle="modal" data-target="#myModal"> Launch demo modal </button> <!-- Modal --> <div class="modal" id="myModal"> <h4>Modal Title</h4> <p> Lorem ipsum dolor sit amet, consectetur adipiscing elit. Maecenas turpis felis, tempus sit amet sollicitudin quis, aliquet ut felis. Nam a malesuada sem. </p> <button type="button" data-dismiss="modal">Close</button> </div>
The HTML code here is simplified version of Bootstrap modal, we use button element with data-toggle and data-target attributes to launch the modal, and button element with data-dismiss attribute in the modal to close the modal.
CSS:
.modal { position: absolute; top: 50%; left: 50%; transform: translateX(-50%) translateY(-50%); background: azure; padding: 1rem; border-radius: 1rem; border: 1px solid gray; display: none; }
The CSS here is to center the modal horizontally and vertically [2], and also set the modal invisible in the beginning.
JavaScript:
'use strict'; var launchBtns = document.querySelectorAll("button[data-toggle='modal']"); for (var i=0; i < launchBtns.length; i++) { var launchBtn = launchBtns[i]; // when user click launch button, show modal. launchBtn.addEventListener("click", function(e) { var selector = e.target.dataset.target; var modal = document.querySelector(selector); modal.style.display = "block"; if (modal.dataset.isSetClose != "true") { // when user click button with `data-dismiss="modal"` in the modal, // close the modal var closeBtns = modal.querySelectorAll("button[data-dismiss='modal']"); for (var j=0; j < closeBtns.length; j++) { var closeBtn = closeBtns[j]; closeBtn.addEventListener("click", function(e) { modal.style.display = "none"; }); } modal.dataset.isSetClose = "true"; } }); }
First we search for button elements with data-toggle="modal". If users click on such buttons, show the modal referenced by data-target attribute of the button element. Also in the click event handler of modal launching buttons, we set the click event handler of modal closing button, which has the data-dismiss="modal" attribute in the modal HTML code.
Tested on:
- Chromium Version 58.0.3029.96 Built on Ubuntu , running on Ubuntu 17.04 (64-bit)
References:
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK