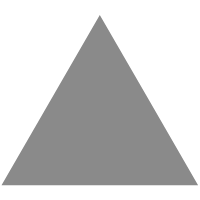
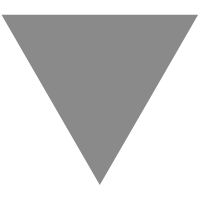
A naturally asynchronous store 🤓
source link: https://dev.to/uppercod/a-naturally-asynchronous-store-57f4
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
In this article you will learn about @atomico/store, a naturally asynchronous, predictable and finite state manager. but first some code 👇.
Preview
interface State {
api: string;
loading: boolean;
products: { id: number; title: string; price: number };
}
const initialState = (state: State) => ({
api: "",
loading: false,
products: [],
});
async function* getProducts(state: State) {
yield { ...state, loading: true };
return {
...(yield),
loading: false,
products: await (await fetch(state.api)).json(),
};
}
const store = new Store(initialState, {
actions: { getProducts },
});
Enter fullscreen mode
Exit fullscreen mode
The above code solves the following goals:
Asynchrony management
Application events and service calls are naturally asynchronous, with @atomico/store you can use asynchronous functions or asynchronous generators to define the update cycle.
update cycle? By this I mean the states that occur sequentially when dispatching the action, example:
async function* getProducts(state: State) {
yield { ...state, loading: true };
return {
...(yield),
loading: false,
products: await (await fetch(state.api)).json(),
};
}
Enter fullscreen mode
Exit fullscreen mode
The previous action will generate 2 states when dispatched:
- state 1:
{loading: true, products:[]}
- state 2:
{loading: false, products:[...product]}
The advantage of this is that the process is clearly observable by the store and by whoever dispatches the action.
Finitely predictable asynchrony
Every action in @atomico/store is wrapped in a promise that defines when it ends its cycle, this will let you execute actions sequentially, example:
await store.actions.orderyBy();
await store.actions.insert({ id: 1000 });
await store.actions.updateAll();
Enter fullscreen mode
Exit fullscreen mode
Modularity and composition
@atomico/store allows to decouple the actions and the state of the store, for a better modularization , example:
actions.js
export interface State {
api: string;
loading: boolean;
products: { id: number; title: string; price: number };
}
export const initialState = (state: State) => ({
api: "",
loading: false,
products: [],
});
export async function* getProducts(state: State) {
yield { ...state, loading: true };
return {
...(yield),
loading: false,
products: await (await fetch(state.api)).json(),
};
}
Enter fullscreen mode
Exit fullscreen mode
store.js
import * as Actions from "./actions";
export default new Store(Actions.initialStore, { actions: { Actions } });
Enter fullscreen mode
Exit fullscreen mode
Example
@atomico/store is AtomicoJS project.
AtomicoJS? Open source project for the creation of interfaces based on webcomponents, @atomico/store was created with Atomico, a library of only 3kB that will allow you to create webcomponents with a functional approach, we invite you to learn more about Atomico and its tools that will improve your experience with webcomponents.
👐 I invite you to join the Atomicojs community and learn more about our projects! 👇
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK