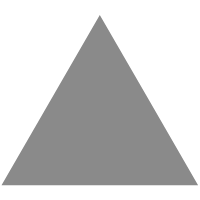
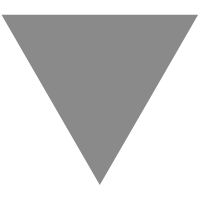
Can OptionParser ignore unknown options, to be processed later in a Ruby program...
source link: https://www.codesd.com/item/can-optionparser-ignore-unknown-options-to-be-processed-later-in-a-ruby-program.html
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
Can OptionParser ignore unknown options, to be processed later in a Ruby program?
Is there any way to kick off OptionParser several times in one Ruby program, each with different sets of options?
For example:
$ myscript.rb --subsys1opt a --subsys2opt b
Here, myscript.rb would use subsys1 and subsys2, delegating their options handling logic to them, possibly in a sequence where 'a' is processed first, followed by 'b' in separate OptionParser object; each time picking options only relevant for that context. A final phase could check that there is nothing unknown left after each part processed theirs.
The use cases are:
In a loosely coupled front-end program, where various components have different arguments, I don't want 'main' to know about everything, just to delegate sets of arguments/options to each part.
Embedding some larger system like RSpec into my application, and I'd to simply pass a command-line through their options without my wrapper knowing those.
I'd be OK with some delimiter option as well, like --
or --vmargs
in some Java apps.
There are lots of real world examples for similar things in the Unix world (startx/X, git plumbing and porcelain), where one layer handles some options but propagates the rest to the lower layer.
Out of the box, this doesn't seem to work. Each OptionParse.parse!
call will do exhaustive processing, failing on anything it doesn't know about. I guess I'd happy to skip unknown options.
Any hints, perhaps alternative approaches are welcome.
Assuming the order in which the parsers will run is well defined, you can just store the extra options in a temporary global variable and run OptionParser#parse!
on each set of options.
The easiest way to do this is to use a delimiter like you alluded to. Suppose the second set of arguments is separated from the first by the delimiter --
. Then this will do what you want:
opts = OptionParser.new do |opts|
# set up one OptionParser here
end
both_args = $*.join(" ").split(" -- ")
$extra_args = both_args[1].split(/\s+/)
opts.parse!(both_args[0].split(/\s+/))
Then, in the second code/context, you could do:
other_opts = OptionParser.new do |opts|
# set up the other OptionParser here
end
other_opts.parse!($extra_args)
Alternatively, and this is probably the "more proper" way to do this, you could simply use OptionParser#parse
, without the exclamation point, which won't remove the command-line switches from the $*
array, and make sure that there aren't options defined the same in both sets. I would advise against modifying the $*
array by hand, since it makes your code harder to understand if you are only looking at the second part, but you could do that. You would have to ignore invalid options in this case:
begin
opts.parse
rescue OptionParser::InvalidOption
puts "Warning: Invalid option"
end
The second method doesn't actually work, as was pointed out in a comment. However, if you have to modify the $*
array anyway, you can do this instead:
tmp = Array.new
while($*.size > 0)
begin
opts.parse!
rescue OptionParser::InvalidOption => e
tmp.push(e.to_s.sub(/invalid option:\s+/,''))
end
end
tmp.each { |a| $*.push(a) }
It's more than a little bit hack-y, but it should do what you want.
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK