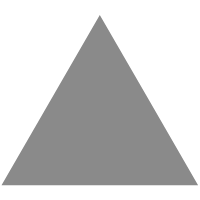
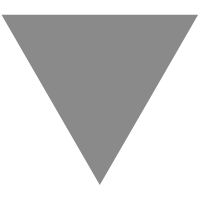
How to serialize the object property to the XML element attribute?
source link: https://www.codesd.com/item/how-to-serialize-the-object-property-to-the-xml-element-attribute.html
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
How to serialize the object property to the XML element attribute?
I've created a object that is serializable and I want to serialize it to XML and then later deserialize back. What I want though is to save one property of this object as XML attribute. Here is what I mean:
[Serializable]
public class ProgramInfo
{
public string Name { get; set; }
public Version Version { get; set; }
}
public class Version
{
public int Major { get; set; }
public int Minor { get; set; }
public int Build { get; set; }
}
I want to save ProgramInfo to XML file that looks like this:
<?xml version="1.0" encoding="utf-8" ?>
<ProgramInfo Name="MyApp" Version="1.00.0000">
</ProgramInfo>
Notice the Version property and its corresponding attribute in XML. I already have parser that turns string "1.00.0000" to valid Version object and vice-versa, but I don't know how to put it to use with this XML serialization scenario.
What you need is a property for the string representation that gets serialized:
[Serializable]
public class ProgramInfo
{
[XmlAttribute]
public string Name { get; set; }
[XmlIgnore]
public Version Version { get; set; }
[XmlAttribute("Version")
public string VersionString
{
get { return this.Version.ToString(); }
set{ this.Version = Parse(value);}
}
}
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK