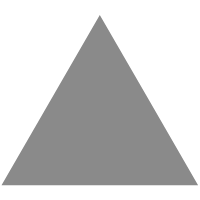
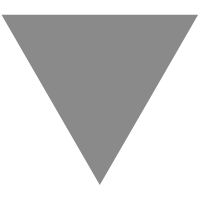
(八)React Ant Design Pro + .Net5 WebApi:后端环境搭建-Aop
source link: https://www.cnblogs.com/WinterSir/p/15939848.html
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
一、Aop#
Aop 面向切面编程(Aspect Oriented Program),在项目中,很多地方都会用到Aop的概念,比如:过滤器(Filter),中间件(Middleware) 通常用来处理数据请求、切面缓存、记录日志、异常捕获等等。但是想在服务层中使用Aop,前面说的就不好使了,目的是减少代码入侵,降低解耦,又能实现业务需求,才是Aop意义所在。前面介绍使用了Autofac,在这还能发挥作用。
1、安装#
安装Autofac.Extras.DynamicProxy,Autofac实现Aop用的是Castle.Core动态代理,Castle.Core可以单独使用,跟Autofac配合起来更方便。Autofac.Extras.DynamicProxy依赖Autofac,所以有的文章是直接就装了这个包,一个效果。
2、异步处理#
Castle.Core本身是不支持异步的,所以参考封装异步Aop类 AsyncInterceptorBase 继承 IInterceptor。
public abstract class AsyncInterceptorBase : IInterceptor
{
public AsyncInterceptorBase()
{
}
public void Intercept(IInvocation invocation)
{
BeforeProceed(invocation);
invocation.Proceed();
if (IsAsyncMethod(invocation.MethodInvocationTarget))
{
InterceptAsync((dynamic)invocation.ReturnValue, invocation);
}
else
{
AfterProceedSync(invocation);
}
}
private bool CheckMethodReturnTypeIsTaskType(MethodInfo method)
{
var methodReturnType = method.ReturnType;
if (methodReturnType.IsGenericType)
{
if (methodReturnType.GetGenericTypeDefinition() == typeof(Task<>) ||
methodReturnType.GetGenericTypeDefinition() == typeof(ValueTask<>))
return true;
}
else
{
if (methodReturnType == typeof(Task) ||
methodReturnType == typeof(ValueTask))
return true;
}
return false;
}
private bool IsAsyncMethod(MethodInfo method)
{
bool isDefAsync = Attribute.IsDefined(method, typeof(AsyncStateMachineAttribute), false);
bool isTaskType = CheckMethodReturnTypeIsTaskType(method);
bool isAsync = isDefAsync && isTaskType;
return isAsync;
}
private async Task InterceptAsync(Task task, IInvocation invocation)
{
await task.ConfigureAwait(false);
AfterProceedAsync(invocation, false);
}
private async Task<TResult> InterceptAsync<TResult>(Task<TResult> task, IInvocation invocation)
{
TResult ProceedAsyncResult = await task.ConfigureAwait(false);
invocation.ReturnValue = ProceedAsyncResult;
AfterProceedAsync(invocation, true);
return ProceedAsyncResult;
}
private async ValueTask InterceptAsync(ValueTask task, IInvocation invocation)
{
await task.ConfigureAwait(false);
AfterProceedAsync(invocation, false);
}
private async ValueTask<TResult> InterceptAsync<TResult>(ValueTask<TResult> task, IInvocation invocation)
{
TResult ProceedAsyncResult = await task.ConfigureAwait(false);
invocation.ReturnValue = ProceedAsyncResult;
AfterProceedAsync(invocation, true);
return ProceedAsyncResult;
}
protected virtual void BeforeProceed(IInvocation invocation) { }
protected virtual void AfterProceedSync(IInvocation invocation) { }
protected virtual void AfterProceedAsync(IInvocation invocation, bool hasAsynResult) { }
}
新建一个服务切面类 ServiceAop 继承 AsyncInterceptorBase
public class ServiceAop : AsyncInterceptorBase
{
private readonly ILogger<ServiceAop> _logger;
public ServiceAop(ILogger<ServiceAop> logger) {
_logger = logger;
}
protected override void BeforeProceed(IInvocation invocation)
{
_logger.LogInformation($"ServiceAop调用方法:{invocation.Method.Name},参数:{JsonConvert.SerializeObject(invocation.Arguments) }");
}
protected override void AfterProceedSync(IInvocation invocation)
{
_logger.LogInformation($"ServiceAop同步返回结果:{JsonConvert.SerializeObject(invocation.ReturnValue)}");
}
protected override void AfterProceedAsync(IInvocation invocation, bool hasAsynResult)
{
_logger.LogInformation($"ServiceAop异步返回结果:{JsonConvert.SerializeObject(invocation.ReturnValue)}");
}
}
两个类放在了新建的Aop文件夹里,通过Autofac注入进行使用,修改 Startup.cs 代码如图:(不太明白的请看:(五)Autofac)
3、使用效果#
二、前人栽树,后人乘凉#
https://blog.csdn.net/q932104843/article/details/97611912
https://www.cnblogs.com/wswind/p/13863104.html
Recommend
-
8
靴子落地,期盼已久的.Net5终于来了! 在3月16号正式发布了第一个预览版本。号称一统江湖的.Net5究竟为我们带来了什么,是...
-
12
“ 11月了,期待已久的.NET5即将发布,你做好准备了吗?如果还只是在Windows上用Visual Studio +...
-
7
让 .NET 开发更简单,更通用,更流行。 Furion 介绍 Furion 是基于 .NET5 平台下打造的现代化 Web 框架。旨在
-
19
#Net5 – Migrating a fully functional Windows Form app to Net 5 in 10 minutes ! elbruno EnglishP...
-
3
Net/NetCore/.NET5 ORM 六大查询体系 - SqlSugar 高级篇 SqlSugar ORM是一款老牌国产ORM框架,生命力也比较顽强,从早期ORM不成熟阶段,一...
-
5
我们知道,当下最火的前端框架,非蚂蚁金服的 AntDesign 莫属,...
-
6
NCF 如何通过WebApi实现前后端分离 昨天参加了《Best Of Microsoft Build》上海...
-
1
一、前言# 先交代一下整个Demo项目结构: 一个认证服务(端口5000)IdentityServer4.Authentic...
-
2
搭建一个简易框架 3秒创建一个WebApi接口
-
2
Generator A React-Native project that creates random users with the help of a webAPI Jul 22, 2023 1 min read
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK