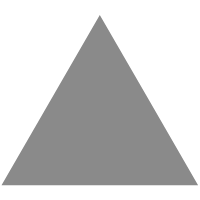
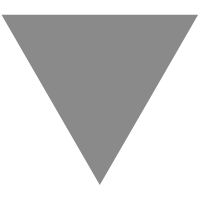
What is Lambda Expression in java? How to Use it?
source link: https://blog.knoldus.com/what-is-lambda-expression-in-java-how-to-use-it/
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
What is Lambda Expression in java? How to Use it?
Reading Time: 3 minutes
Lambda Expressions get add-in Java 8. Java lambda expressions are Java’s first step into functional programming. Its main objective is to increase the expressive power of the language.
The syntax of a basic lambda expression is:
parameter -> expression
The expression use as the code body for the abstract method within the paired functional interface. lambda expressions exist outside of any object’s scope. This means they are callable anywhere in the program.
What is Functional Interface in Java?
If a Java interface contains one and only one abstract method then it is termed a functional interface. This only one method specifies the intended purpose of the interface.
The syntax of a basic Functional Interface is:
@FunctionalInterface
interface interfacename{
void methodName(parameters...);
}
Here, we have used the annotation @FunctionalInterface
. The annotation forces the Java compiler to indicate that the interface is a functional interface.
Code Example :
@FunctionalInterface
interface sayable{
void say(String msg);
}
public class BlogExample implements sayable{
public void say(String msg){
System.out.println(msg);
}
public static void main(String[] args) {
BlogExample obj = new BlogExample();
obj.say("Hello there");
}
}
Output :
Types of Lambda Body in Java
In Java, the lambda body is of two types.
A body with a single expression
This type of lambda body is known as the expression body.
() -> System.out.println("Blog on Lambdas Expression");
A body that consists of a block of code
This type of lambda body is known as a block body.
() -> {
String name = "Knoldus Blogs";
return name;
};
Lambda Expression in Java
A lambda expression can be :
- No Parameter Syntax
- One Parameter Syntax
- Multiple Parameters Syntax
No Parameter Syntax
The syntax of a No Parameter lambda expression is:
import java.util.concurrent.Callable;
public class BlogExample {
public static void main(String[] args) throws Exception {
Callable<String> callMe = () -> "Zero parameter lambda expression";
System.out.println(callMe.call());
}
}
Output :
One Parameter Syntax
The syntax of a No One Parameter lambda expression is:
import java.util.ArrayList;
public class BlogExample {
public static void main(String[] args) {
ArrayList<Integer> numbers = new ArrayList<Integer>();
numbers.add(4);
numbers.add(3);
numbers.add(2);
numbers.add(1);
numbers.forEach( (n) -> { System.out.println(n); } );
}
}
Output :
Multiple Parameters Syntax
The syntax of a Multiple Parameter lambda expression is:
import java.util.function.*;
public class BlogExample {
public static void main(String args[]) {
Message m = new Message();
m.printStringInteger("Java", 75,
(String str, Integer number) -> {
System.out.println("The values are: " + str + " " + number);
});
}
private static class Message {
public void printStringInteger(String str, Integer number, BiConsumer<String, Integer> biConsumber) {
biConsumber.accept(str, number);
}
}
}
Output :
Features of Lambda Expressions
- A lambda expression can have zero, one or more parameters.
- When there is a single statement curly brackets are not mandatory and the return type of the anonymous function is the same as that of the body expression.
- The compiler will allow us to use an inner class to instantiate a functional interface; however, this can lead to very verbose code.
Thanks for Reading !!
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK