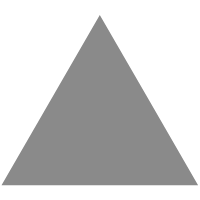
1
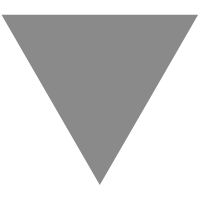
Reverse Each word in a String
source link: https://dev.to/sureshayyanna/reverse-each-word-in-a-string-1868
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
Reverse Each word in a String
Program to Reverse each word in String
package InterviewPrograms;
import java.util.Scanner;
public class ReverseEachWord {
// Method 01
public static String revWordsOfString(String str) {
// s1.Split the String with whitespace
String[] words = str.split(" ");
// s2. Using for each loop read each word and reverse it
String revstr = "";
for (String w : words) {
String revword = "";
for (int i = w.length() - 1; i >= 0; i--) {
revword = revword + w.charAt(i);
}
revstr = revstr + revword + " ";
}
return revstr;
}
//Method 02
public static String revWordOfString(String str) {
// s1.Split the String with Space Reg.Expression(\\s)
String[] word = str.split(" \\s");
String revword = "";
for (String w : word) {
StringBuilder sb = new StringBuilder(w);
sb.reverse();
revword = revword + sb.toString() + " ";
}
return revword;
}
public static void main(String[] args) {
System.out.println("Enter the Actual String:");
Scanner input = new Scanner(System.in);
String actualString = input.nextLine();
String ExpectedString = revWordsOfString(actualString);
System.out.println("The Reverse word of each string of given String from Method 1: " + ExpectedString);
System.out.println(
"The Reverse word of each string of given String from Method 2:" + revWordOfString(actualString));
}
}
Enter fullscreen mode
Exit fullscreen mode
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK