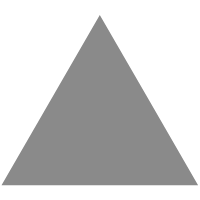
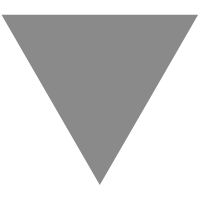
Check If a String is a valid IP Address in Python
source link: https://thispointer.com/check-if-a-string-is-a-valid-ip-address-in-python/
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
In this article, we will discuss three different ways to check if a string is a valid IP Address in Python.
Table of Contents
Check If a String is a valid IP Address using Regex
In Python, the regex module provides a member function search(), which accepts a string and a regex pattern as arguments. Then it looks for the substrings in the given string based on that regex pattern. If the pattern is found then it returns a Match object, otherwise returns None. We are going to use the same regex.search() function with a regex pattern –> “^(\d{1,3})\.(\d{1,3})\.(\d{1,3})\.(\d{1,3})$”. This regex pattern looks for a valid IP Address string. It verifies that the string contains four numbers (three digit), seperated by dots. Then we need to check that each of this number is in a range of 0 to 255.
We have created a separate function, that will use this regex pattern to check if a given string is valid or not i.e.
import re def valid_IP_Address(sample_str): ''' Returns True if given string is a valid IP Address, else returns False''' result = True match_obj = re.search( r"^(\d{1,3})\.(\d{1,3})\.(\d{1,3})\.(\d{1,3})$", sample_str) if match_obj is None: result = False else: for value in match_obj.groups(): if int(value) > 255: result = False break return result
Let’s use this function to with certain strings to verify if they contain a valid IP Address. For example,
Advertisements
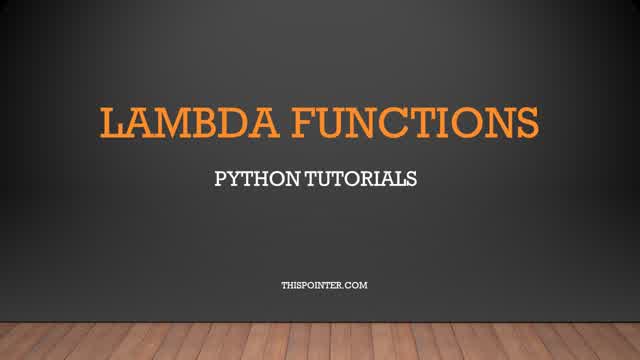
def test_func(ip_str): if valid_IP_Address(ip_str): print(f'Yes, string "%s" is a valid Ip Address' % (ip_str)) else: print(f'No, string "%s" is not a valid Ip Address' % (ip_str)) test_func("192.168.1.1") test_func("1234.168.1.1") test_func("192.168.1.ccc") test_func("0.0.0.0") test_func("255.255.255.255") test_func("256.256.256.256") test_func("300.300.300.300") test_func("192.f18.1.1")
Output:
Yes, string "192.168.1.1" is a valid Ip Address No, string "1234.168.1.1" is not a valid Ip Address No, string "192.168.1.ccc" is not a valid Ip Address Yes, string "0.0.0.0" is a valid Ip Address Yes, string "255.255.255.255" is a valid Ip Address No, string "256.256.256.256" is not a valid Ip Address No, string "300.300.300.300" is not a valid Ip Address No, string "192.f18.1.1" is not a valid Ip Address
In the regex pattern we matched each number in the IP Address as a separate group. If the regex match is valid, we check that the each value in the four groups is from range 0 to 255.
Check If a String is a valid IP Address using ipaddress module
We can use the ipaddress module in Python, to verify if a string is a valid IP or not. If the ipaddress module is not installed, then you can install it using command,
pip install ipaddress
The ipaddress module provides a function ip_network(str). It accepts the IP Addtess string as argument and returns object of the correct type if given string is a valid IP Address. It will raise ValueError, if the string passed isn’t either a v4 or a v6 IP Address.
We have created a function that uses the ipaddress module to verify if a string is a valid IP Address or not,
import ipaddress def is_valid_IPAddress(sample_str): ''' Returns True if given string is a valid IP Address, else returns False''' result = True try: ipaddress.ip_network(sample_str) except: result = False return result
Let’s use this function to with certain strings to verify if they contain a valid IP Address. For example,
def test_func(ip_str): if is_valid_IPAddress(ip_str): print(f'Yes, string "%s" is a valid Ip Address' % (ip_str)) else: print(f'No, string "%s" is not a valid Ip Address' % (ip_str)) test_func("192.168.1.1") test_func("1234.168.1.1") test_func("192.168.1.ccc") test_func("0.0.0.0") test_func("255.255.255.255") test_func("256.256.256.256") test_func("300.300.300.300") test_func("192.f18.1.1")
Output:
Yes, string "192.168.1.1" is a valid Ip Address No, string "1234.168.1.1" is not a valid Ip Address No, string "192.168.1.ccc" is not a valid Ip Address Yes, string "0.0.0.0" is a valid Ip Address Yes, string "255.255.255.255" is a valid Ip Address No, string "256.256.256.256" is not a valid Ip Address No, string "300.300.300.300" is not a valid Ip Address No, string "192.f18.1.1" is not a valid Ip Address
Check If a String is a valid IP Address using socket module
Python provides a socket module, which has a function inet_aton(). It converts a string format IPv4 address to a 32-bit packed binary format. If the given string is not a valid IP Address, it raises a error.
We have created a function that uses the socket module to verify if a string is a valid IP Address or not,
import socket def is_valid_IP_Address(ip_str): ''' Returns True if given string is a valid IP Address, else returns False''' result = True try: socket.inet_aton(ip_str) except socket.error: result = False return result
Let’s use this function to with certain strings to verify if they contain a valid IP Address. For example,
def test_func(ip_str): if is_valid_IP_Address(ip_str): print(f'Yes, string "%s" is a valid Ip Address' % (ip_str)) else: print(f'No, string "%s" is not a valid Ip Address' % (ip_str)) test_func("192.168.1.1") test_func("1234.168.1.1") test_func("192.168.1.ccc") test_func("0.0.0.0") test_func("255.255.255.255") test_func("256.256.256.256") test_func("300.300.300.300") test_func("192.f18.1.1")
Output:
Yes, string "192.168.1.1" is a valid Ip Address No, string "1234.168.1.1" is not a valid Ip Address No, string "192.168.1.ccc" is not a valid Ip Address Yes, string "0.0.0.0" is a valid Ip Address Yes, string "255.255.255.255" is a valid Ip Address No, string "256.256.256.256" is not a valid Ip Address No, string "300.300.300.300" is not a valid Ip Address No, string "192.f18.1.1" is not a valid Ip Address
Summary
We learned about three different ways to validate if a string contains a valid IP Address or not.
Advertisements
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK