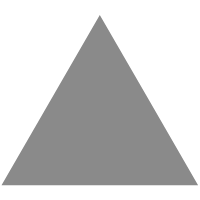
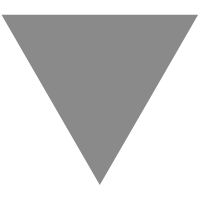
Easy console.log() inside one liner functions
source link: https://dev.to/js_bits_bill/easy-consolelog-inside-one-liner-functions-2mja
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
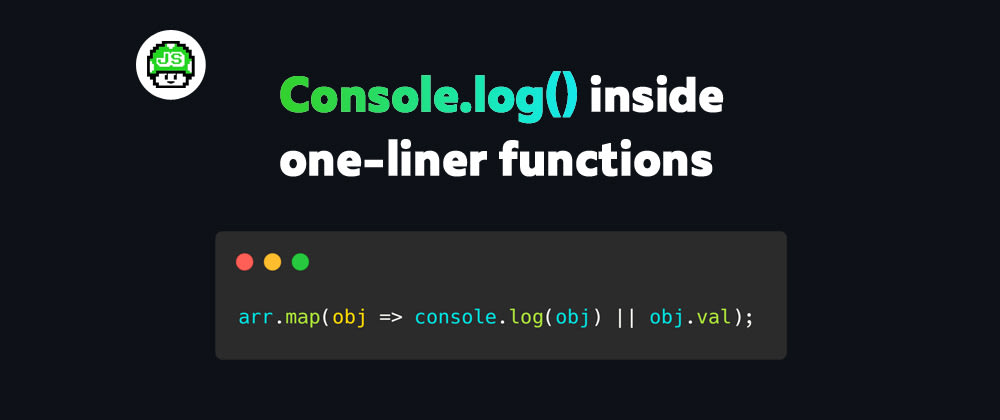
Easy console.log() inside one liner functions
Let's say we want to log obj
inside this .map()
function here:
const arr = [
{ val: 1 },
{ val: 2 },
{ val: 3 }
];
const nums = arr.map(obj => obj.val*2);
Well, dang! Now we have to convert this to a multi-line function, right?
const nums = arr.map(obj => {
console.log(obj);
return obj.val * 2;
});
Instead we can use the logical OR (||
) operator with console.log()
to short-circuit evaluate the return statement:
const nums = arr.map(obj => console.log(obj) || obj.val*2);
This works because console.log()
evaluates to undefined
so our OR (||
) opertator will evalutate the next operand which is the return portion of the function and will return the same result as the original example!
This is especially usefull with JSX where we commonly render components with implicit return statements:
export default function App() {
return (
<div>
<h2>Short-circuit Logging</h2>
<ul>
{nums.map((num) => console.log(num) || (
<li key={num}>{num}</li>
))}
</ul>
</div>
);
}
Huzzah! 😃
Yo! I post byte-sized tips like these often. Follow me if you crave more! 🍿
I'm on Twitter, TikTok and I have a new debugging course dropping soon!
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK