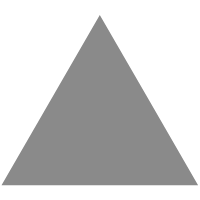
3
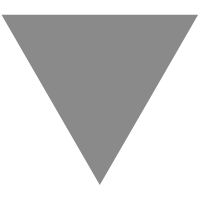
【C#】简易人机对抗“石头剪刀布”游戏
source link: https://blog.csdn.net/JeronZhou/article/details/121088532
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
需要实现如下图所示的人机猜拳小游戏:
我们需要建立一个玩家类Player、一个电脑类Computer、一个裁判类Judge来分别模拟各自的操作:
【Player.cs】
/*
* 作者:JeronZhou
* 时间:2021-11-01
* 功能:石头剪刀布游戏
*/
using System;
namespace Test2_2
{
public class Player
{
public string FistName { get; set; }
public int Play(string name)
{
FistName = name;
switch (FistName)
{
case "石头":
return 1;
case "剪刀":
return 2;
case "布":
return 3;
default:
return 0;
}
}
}
}
【Computer.cs】
/*
* 作者:JeronZhou
* 时间:2021-11-01
* 功能:石头剪刀布游戏
*/
using System;
namespace Test2_2
{
public class Computer
{
public string FistName { get; set; }
public int RandomPlay()
{
Random random = new Random(Guid.NewGuid().GetHashCode());
int num = random.Next(1, 4);
switch (num)
{
case 1:
FistName = "石头";
break;
case 2:
FistName = "剪刀";
break;
case 3:
FistName = "布";
break;
}
return num;
}
}
}
【Judge.cs】
/*
* 作者:JeronZhou
* 时间:2021-11-01
* 功能:石头剪刀布游戏
*/
using System;
namespace Test2_2
{
public class Judge
{
public string Win(int play, int computer)
{
int result = play - computer;
switch (result)
{
case -1:
return "你赢了";
case 2:
return "你赢了";
case -2:
return "你输了";
case 1:
return "你输了";
default:
return "平手";
}
}
}
}
【窗体设计】
共有5个标签(3个空标签),三个按钮。
【MainForm.cs】
/*
* 作者:JeronZhou
* 时间:2021-11-01
* 功能:石头剪刀布游戏
*/
using System;
using System.Windows.Forms;
namespace Test2_2
{
public partial class MainForm : Form
{
public MainForm()
{
InitializeComponent();
}
void Button1Click(object sender, EventArgs e)
{
Player p = new Player();
int playerName = p.Play(button1.Text);
label3.Text = p.FistName;
Computer c = new Computer();
int computerName = c.RandomPlay();
label4.Text = c.FistName;
Judge judge = new Judge();
label5.Text = judge.Win(playerName, computerName);
}
void Button2Click(object sender, EventArgs e)
{
Player p = new Player();
int playerName = p.Play(button2.Text);
label3.Text = p.FistName;
Computer c = new Computer();
int computerName = c.RandomPlay();
label4.Text = c.FistName;
Judge judge = new Judge();
label5.Text = judge.Win(playerName, computerName);
}
void Button3Click(object sender, EventArgs e)
{
Player p = new Player();
int playerName = p.Play(button3.Text);
label3.Text = p.FistName;
Computer c = new Computer();
int computerName = c.RandomPlay();
label4.Text = c.FistName;
Judge judge = new Judge();
label5.Text = judge.Win(playerName, computerName);
}
}
}
【Program.cs】
/*
* 作者:JeronZhou
* 时间:2021-11-01
* 功能:石头剪刀布游戏
*/
using System;
using System.Windows.Forms;
namespace Test2_2
{
internal sealed class Program
{
[STAThread]
private static void Main(string[] args)
{
Application.EnableVisualStyles();
Application.SetCompatibleTextRenderingDefault(false);
Application.Run(new MainForm());
}
}
}
【测试结果】
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK