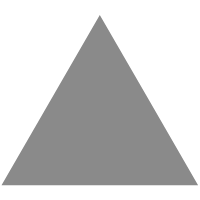
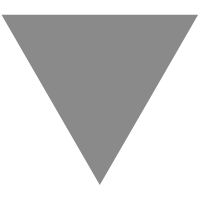
Enabling CORS in Node.js
source link: https://blog.knoldus.com/enabling-cors-in-node-js/
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
Enabling CORS in Node.js
Reading Time: 2 minutes
In the last blog, we’ve learnt the basic about the CORS, how we can setup and how it works. Now ini this blog, we’re going to learn how we can enable the it in node.js
CORS is a browser security feature that restricts cross-origin HTTP requests with other servers and specifies which domains access your resources.
Let’s say accessing images, videos, iframes, or scripts from another server. This means that the website is accessing resources from a different origin or domain. When building an application to serve up these resources with Express, a request to such external origins may fail. This is where CORS comes in to handle cross-origin requests.
If you want know more about it, you can refer here.
Prerequisites:
To follow this article along, prior knowledge of Node.js and Express is essential.
Installation
This is a Node.js module available through the npm registry. Installation is done using the npm install command.
$ npm install cors
Now let’s see CORS usage one by one:
1) Simple Usage (Enable All CORS Requests)
var express = require('express')
var cors = require('cors')
var app = express()
app.use(cors())
app.get('/products/:id', function (req, res, next) {
res.json({msg: 'This is CORS-enabled for all origins!'})
})
app.listen(80, function () {
console.log('CORS-enabled web server listening on port 80')
})
2) Enable CORS for a Single Route
var express = require('express')
var cors = require('cors')
var app = express()
app.get('/products/:id', cors(), function (req, res, next) {
res.json({msg: 'This is CORS-enabled for a Single Route'})
})
app.listen(80, function () {
console.log('CORS-enabled web server listening on port 80')
})
3) Configuring CORS
var express = require('express')
var cors = require('cors')
var app = express()
var corsOptions = {
origin: 'http://example.com',
optionsSuccessStatus: 200 // some legacy browsers (IE11, various SmartTVs) choke on 204
}
app.get('/products/:id', cors(corsOptions), function (req, res, next) {
res.json({msg: 'This is CORS-enabled for only example.com.'})
})
app.listen(80, function () {
console.log('CORS-enabled web server listening on port 80')
})
Configure CORS with Options:
You can also use the configuration options with CORS to customize this further. You can use configuration to allow a single domain or subdomains access, configure HTTP methods that are allowed such as GET
and POST
depending on your requirements.
If we send a POST
request from http://localhost:8080
, it’ll be blocked by the browser as only GET
and PUT
are supported:
fetch('http://localhost:2020', {
method: 'POST',
body: JSON.stringify({name: "ABCD"}),
})
.then(response => response.json())
.then(data => console.log(data))
.catch(err => console.error(err));
To see a full list of configuration options, please refer the official documentation.
The default configuration is the equivalent of:
{
"origin": "*",
"methods": "GET,HEAD,PUT,PATCH,POST,DELETE",
"preflightContinue": false,
"optionsSuccessStatus": 204
}
For details on the effect of each header, read this article on HTML5 Rocks.
Conclusion:
In this blog, we’ve learnt how we can enable the CORS in node.js. We’ve also seen how we can configure them too in the simple way. There are several configurations option available when we wants to enable them.
Hey there, I am glad you have reached the end of this post. If you liked this post or have some questions or want to discuss something let me know in the comment section.
For more info you can check:
https://expressjs.com/en/resources/middleware

Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK