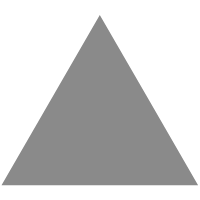
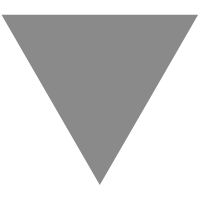
Locator strategy using Selenium 4.0
source link: https://blog.knoldus.com/locator-strategy-using-selenium-4-0/
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
In the last blog, we saw how we can setup a maven project in Selenium (Getting started with Selenium 4.0) and now we will see different Locator strategy using Selenium 4.0
Why Locators are required?
- When we go to any website it has multiple fields, buttons, forms, etc. We need to uniquely identify the elements present on the website in order to successfully automate different flows.
- If we want to click a particular button, it is possible that the page contains multiple buttons. So, how will you identify which button to click?
- This is where the locators come into play. They help us identify each and every element uniquely present on the web page.
How to get the locators?
- Go to any web page, right click anywhere on the page, and select the inspect option from the menu.
- You can select any particular element on the page you can use the inbuilt selector tool to see it’s attributes (ID, name,etc).
- You can also use the web browser auto-generated XPath or CSS selector as well.
- Go to the element —> Right click —> Copy —> Copy XPath or Copy selector (For CSS selector).
Locators in Selenium
- Link text
- XPath
- CSS selector
ID and name
- ID and name are the most common locators that we use to identify the elements on a page.
- The main issue with using ID or name for locating elements is that multiple elements can have the same ID or name. And in some cases, ID and name are not present at all.
- Also if the ID is an alphanumeric value then it is possible that it might change its value once the web page refreshes.
- For example – On a web page there can be multiple buttons all with the same ID or same names, So, it becomes difficult to identify and interact with the element.
In the given code we will be trying to hit the Salesforce login using ID and name locators.
public class SeleniumChromeIDLocatorTest {
public static void main(String[] args) {
System.setProperty("webdriver.chrome.driver", "/home/ankur/Documents/Selenium/chromedriver");
//Setting up the driver and giving the path to the driver file
WebDriver driver = new ChromeDriver();
//Initialize the driver
driver.get("https://login.salesforce.com/?locale=in");
//Hitting the URL we want to test
driver.findElement(By.id("username")).sendKeys("[email protected]");
driver.findElement(By.id("password")).sendKeys("[email protected]");
driver.findElement(By.id("Login")).click();
//Finding the elements using the id locator
driver.close();
// To close window that has the focus
}
}
public class SeleniumChromeNameLocatorTest {
public static void main(String[] args) {
System.setProperty("webdriver.chrome.driver", "/home/ankur/Documents/Selenium/chromedriver");
//Setting up the driver and giving the path to the driver file
WebDriver driver = new ChromeDriver();
//Initialize the driver
driver.get("https://login.salesforce.com/?locale=in");
//Hitting the URL we want to test
driver.findElement(By.name("username")).sendKeys("[email protected]");
driver.findElement(By.name("pw")).sendKeys("[email protected]");
driver.findElement(By.name("Login")).click();
//Finding the elements using the name locator
driver.close();
// To close window that has the focus
}
}
Link text
- The link text can be used in the case where there are ID and name both attributes are not available and the element with which we want to interact has an anchor tag to it.
- We use the hyperlink’s text to find the element.
In the given code we will be trying to hit the facebook login and try to hit the Forgotten password link using the link text locator.
public class SeleniumChromeLinkTextLocatorTest {
public static void main(String[] args) {
System.setProperty("webdriver.chrome.driver", "/home/ankur/Documents/Selenium/chromedriver");
//Setting up the driver and giving the path to the driver file
WebDriver driver = new ChromeDriver();
//Initialize the driver
driver.get("https://facebook.com");
//Hitting the URL we want to test
driver.findElement(By.linkText("Forgotten password?")).click();
//Finding the elements using the linkText locator
driver.close();
// To close window that has the focus
}
}
XPath and CSS selector
- XPath and CSS selectors are used for finding the elements when locators like ID or name are not available to be used.
- We can generate and use XPath or CSS selector using our web browsers but they are not 100% reliable. They might change when the user refreshes the page or changes are made to the web-page.
- We can also generate our own XPaths or CSS selectors which are much more reliable than the browser generated ones.
- If id or name attributes are available then we should use them as they are always unique and does not change very often.
In the given code we will be trying to hit the Salesforce login using XPath and CSS selectors.
public class SeleniumChromeXpathLocatorTest {
public static void main(String[] args) {
System.setProperty("webdriver.chrome.driver", "/home/ankur/Documents/Selenium/chromedriver");
//Setting up the driver and giving the path to the driver file
WebDriver driver = new ChromeDriver();
//Initialize the driver
driver.get("https://login.salesforce.com/?locale=in");
//Hitting the URL we want to test
driver.findElement(By.xpath("//*[@id=\'username\']")).sendKeys("[email protected]");
driver.findElement(By.xpath("//*[@id=\'password\']")).sendKeys("incorrectPassword");
driver.findElement(By.xpath("//*[@id=\'Login\']")).click();
System.out.println(driver.findElement(By.cssSelector("#error")).getText());
//Finding the elements using the xpath locator
System.out.println();
driver.close();
// To close window that has the focus
}
}
public class SeleniumChromeCSSLocatorTest {
public static void main(String[] args) {
System.setProperty("webdriver.chrome.driver", "/home/ankur/Documents/Selenium/chromedriver");
//Setting up the driver and giving the path to the driver file
WebDriver driver = new ChromeDriver();
//Initialize the driver
driver.get("https://login.salesforce.com/?locale=in");
//Hitting the URL we want to test
driver.findElement(By.cssSelector("#username")).sendKeys("[email protected]");
driver.findElement(By.cssSelector("#password")).sendKeys("password");
driver.findElement(By.cssSelector("#Login")).click();
//Finding the elements using the cssSelector locator
driver.close();
// To close window that has the focus
}
}
So, this was a short blog on Locator strategy using Selenium 4.0. In the next blog we will see how we can work with drop-down menu and checkbox .
References
Techhub template
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK