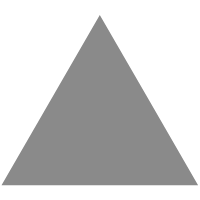
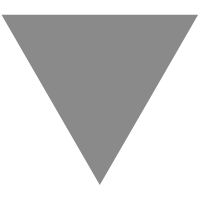
Global Using Statements and Code Clues
source link: https://www.stevefenton.co.uk/2021/08/global-using-statements-and-code-clues/
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
Global Using Statements and Code Clues
I wrote back in 2018 about how you can use your using
statements as a clue to how you might dismember a big class. This makes it easier to see the important parts as you remove things that you “kinda have to have” to make the important stuff work. The trick is pretty simple…
- You open your class file
- You look at the using statements
- You chunk your using statements into purposes
- You extract out those purposes
- You push the extractions out of the class
A practical example is shown below…
using Microsoft.WindowsAzure.Storage; using Microsoft.WindowsAzure.Storage.Blob; using Microsoft.WindowsAzure.Storage.Queue; using System; using System.Collections.Generic; using System.Data.SqlClient; using System.IO; using System.Linq; using System.Net.Http; using System.Text.RegularExpressions;
That’s a lot of usings. This is a clue the class does too much. Let’s organise them visually (temporarily) and work out how we might push them into other places. Perhaps like this:
// Web Jobs using Microsoft.WindowsAzure.Storage; using Microsoft.WindowsAzure.Storage.Blob; using Microsoft.WindowsAzure.Storage.Queue; // SQL using System.Data.SqlClient; // File System using System.IO; // HTTP using System.Net.Http; // Pretty basic stuff using System; using System.Collections.Generic; using System.Linq; using System.Text.RegularExpressions;
C# 10 Global Usings
C# 10 is going to get a really neat new feature called global usings. It will allow you to remove a lot of repetition from your classes by declaring a using statement that applies everywhere.
Now, this could interrupt our ability to use using statements as clues to improve our code, unless we use it smartly. Going back to the previous example, we should be looking to include the “pretty basic stuff” with global using statements, but not the rest. Your team’s definition of “pretty basic stuff” may vary, but it should reflect both existing common usage and stuff that would not be surprising.
Let’s add a new file and add our global usings:
global using System; global using System.Collections.Generic; global using System.Linq; global using System.Text.RegularExpressions;
This prevents repetition of pretty obvious using statements, but it doesn’t prevent us using “local” using statements as a trigger for a clean up. Our starting point is just simpler:
using Microsoft.WindowsAzure.Storage; using Microsoft.WindowsAzure.Storage.Blob; using Microsoft.WindowsAzure.Storage.Queue; using System.Data.SqlClient; using System.IO; using System.Net.Http;
Summary
You can look at your using statements and get a list of suggestions of things that might be better if they were moved out of your class. Maybe that amazing mortgage calculator shouldn’t actually directly use System.IO
, and moving that stuff elsewhere would make the mortgage calculator logic more readable. Global using statements, if used correctly, make this technique even easier by removing common and unsurprising using statements from the list, making the unusual ones easier to analyse.
Written by Steve Fenton on 11th August 2021
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK