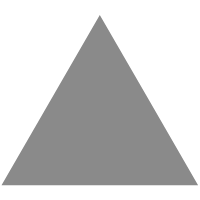
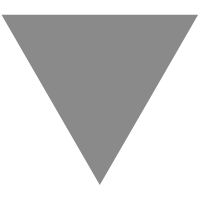
Using the user input to call an integer
source link: https://www.codesd.com/item/using-the-user-input-to-call-an-integer.html
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
Using the user input to call an integer
I am a beginner in coding, and I am trying to create a simple program that when a reader types in their name, the program shows how much money they owe. I was thinking of using a Scanner
, next(String)
, and int
.
import java.util.Scanner;
public class moneyLender {
//This program will ask for reader input of their name and then will output how much
//they owe me. (The amount they owe is already in the database)
public static void main(String[] args) {
int John = 5; // John owes me 5 dollars
int Kyle = 7; // Kyle owes me 7 dollars
//Asking for reader input of their name
Scanner reader = new Scanner(System.in);
System.out.print("Please enter in your first name:");
String name = reader.next();
//my goal is to have the same effect as System.out.println("You owe me " + John);
System.out.println("You owe me: " + name) // but not John as a string but John
// as the integer 5
//Basically, i want to use a string to call an integer variable with
//the same value as the string.
}
}
As a beginner, You might be want to use a simple HashMap
, which will store these mappings as key, value pair. Key
will be name
and value
will be money
. Here is example:
import java.util.HashMap;
import java.util.Map;
import java.util.Scanner;
public class moneyLender {
public static void main(String[] args) {
Map<String, Integer> map = new HashMap<String, Integer>();
map.put("John", 5);
map.put("Kyle", 7);
Scanner reader = new Scanner(System.in);
System.out.print("Please enter in your first name:");
String name = reader.next();
System.out.println("You owe me: " + map.get(name)); //
}
}
Output :
Please enter in your first name:John
You owe me: 5
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK