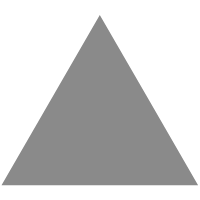
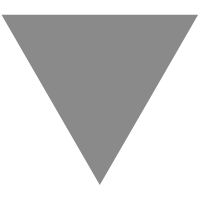
Quick Guide Bitbucket Pipelines on Running Selenium C# Tests
source link: https://www.automatetheplanet.com/bitbucket-pipelines-csharp-tests/?utm_campaign=bitbucket-pipelines-csharp-tests
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
Quick Guide Bitbucket Pipelines on Running Selenium C# Tests
In this article from the series Automation Tools, I am going to guide you on how you can set up a Bitbucket Pipelines job for a Selenium C# project, run your Selenium tests, and display the report with the test results, all that without ever leaving the Bitbucket website.
What Is Bitbucket Pipelines?
Bitbucket Pipelines is one of the most recent workflow mechanisms that are quick and easy to set up directly from the Bitbucket repository. It allows you to automatically build, test, and even deploy your code based on a configuration file in your repository. Essentially, it creates containers in the cloud for you. Inside these containers, you can run commands (like you might on a local machine) but with all the advantages of a fresh system, customized and configured for your needs.
Why Do You Need CI/CD?
CI/CD, short for Continuous Integration and Continuous Delivery/Deployment, is a collection of practices and operating principles that enable teams to deliver code changes more frequently and reliably. The implementation is usually called CI/CD pipeline.
Continuous Integration establishes a consistent automated way of building, packaging, and testing applications. This helps teams deliver code changes more frequently, which improves collaboration and software quality.
Continuous Delivery starts where Continuous Integration ends. It automates the delivery of applications to selected environments. For example, teams usually work on multiple environments (development, testing, staging, production), and Continuous Delivery helps with an automated way to push code changes to them.
The logic behind using such principles is, so teams commit smaller code changes more frequently. Continuous Integration usually checks if the code builds and the tests run successfully on each commit to the repository, so it's easier to identify defects earlier and improve software quality. Continuous Deployment helps those changes get to the production environment seamlessly when all the tests have been carried out.
Setting Up The Tests
In the example, we're going to build a simple NUnit .NET Core project, which will test the proper validation of the fields of Bootstrap 5's example Checkout form. We're checking if the appropriate error message is displayed on each required field, if it is empty when submitting and if the form submits if all the fields have valid info.

I create a page object split into 3 files using partial classes. We have one file for holding all actions: Map, which contains all elements with their respective locators, and the last one with all assertions.
Actions
We pass the initialized driver through the constructor, and the most important method here is FillInfo. Finally, we give as a parameter the client information that we need to set into the form. I used the C# 9.0 record to ease the process.
A record is still a class, but the record keyword imbues it with several additional value-like behaviors. Generally speaking, records are defined by their contents, not their identity. In this regard, records are much closer to structs, but records are still reference types. While records can be mutable, they are primarily built for better supporting immutable data models.
In the CheckoutPage.Map.cs file I defined all elements as expression-body-get properties. So if needed, you can easily update the locator in a single place. Also, for web pages with many elements, this approach better organizes the actions, elements, and assertions, instead of having everything in one huge file.
Assertions
For a more detailed overview and usage of many more design patterns and best practices in automated testing, stay tuned for my upcoming book "Design Patterns for High-Quality Automated Tests, C# Edition, High-Quality Tests Attributes, and Best Practices". It will be published at the end of February. You can read part of three of the chapters:
Defining High-Quality Test Attributes for Automated Tests
Benchmarking for Assessing Automated Test Components Performance
Checkout Tests
NOTE: We're setting up Chrome to run in the headlessmode because the runners don't display output and will probably crash the driver.
NOTE: We use the WebDriverManagerlibrary, which automatically detects my machine's browser version and downloads the proper driver for it.
To be able to publish the results to Bitbucket Pipelines you need to install the JUnitTestLogger NuGet package.
Getting Started with Bitbucket Pipelines
1. Create a new Atlassian account and create a new Bitbucket account.

2. Create a new Bitbucket project
3. Create a new Git repository

4. Fill the required information

Below you can see the result.

5. Click the Clone button and add the tests' code
We are ready to proceed with creating a new pipeline that will execute the tests in CI.
6. Click the Pipelines button and select "Build and test a .Net code" pipeline template

7. To proceed with the setup, you need to enable the Two-step verification of your account. First, follow the steps described in the official documentation.
8. The .NET code template contains most of the things you need to download the source code and run the Selenium tests.
9. Edit the code before committing the file and change it with the following one:
10. After you make a push to the remote repository a new pipeline build will be triggered.

From my experience with Bitbucket Pipelines and other CI tools, I would suggest configuring your automated Selenium tests to run in the cloud, custom Selenium grid, or in Docker containers. Also, to ease the test failure analysis, consider integrating a solution such as Allureor ReportPortal since the information displayed in the builds here is insufficient. Also, you can check another superb AI-powered solution for healing automatically some of your tests - Healenium.
Summary
Bitbucket Pipelines is one of the most recent workflow mechanisms that are quick and easy to set up directly from the Bitbucket website. It allows you to automatically build, test, and even deploy your code based on a configuration file in your repository. Essentially, it creates containers in the cloud for you. Inside these containers, you can run commands.
Online Training
NON-FUNCTIONAL
START:13 October 2021
Enterprise Test Automation Framework
LEVEL: 3 (Master Class)
After discussing the core characteristics, we will start writing the core feature piece by piece.
We will continuously elaborate on why we design the code the way it is and look into different designs and compare them. You will have exercises to finish a particular part or extend it further along with discussing design patterns and best practices in programming.
Duration: 30 hours
4 hour per day
-20% coupon code:
BELLATRIX20
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK