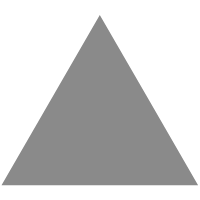
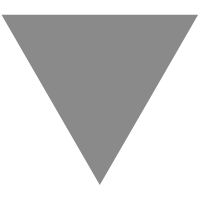
Revit插件开发快速教程【含源码】
source link: http://blog.hubwiz.com/2021/06/07/revit-addin-tutorial/
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
在这个教程中我们将学习如何开发一个Revit插件,并利用此插件实现设计自动化。课程中的源代码 可以从github下载。
第一步是创建 C# Libray新项目,这就是我们的”DeleteDoors”插件项目。
1、Visual Studio配置
在VS 界面中需要配置大量元素,以便能够正确编译代码。在工具栏中单击 Debug >🛠 DeleteDors 并按照 以下步骤操作:
1- 使用 Revit v2021 的用户应确保目标框架为 .NET 框架 4.8
2- 将调试器定义为外部程序,并指向revit.exe的路径
3- 定义在构建事件时保存插件的路径
由于是在命令行,因此你可以创建插件事件:
xcopy "$(TargetPath)" "%ProgramData%/Autodesk\Revit\Addins\2021\"
xcopy "$(ProjectDir)DeleteDoors.addin" "%ProgramData%/Autodesk\Revit\Addins\2021\"
2、定义引用项
在 VS 的解决方案浏览器中,添加 2 个重要的引用项(扩展.dll): RevitAPI和RevitAPIUI。 你可以浏览”C:\Program Files\Autodesk\Revit 2021”来找到这些引用项。
将这两个引用项的Copy Local属性设置为False。
3、DeleteDoors功能实现
本课程的目标是创建一个外部命令,该命令将能够删除项目中的所有门,并在没有门构件时发出提示消息。
代码中包含 3 个函数:Execute、DeleteAllDoors和DeleteElement,前一个函数会调用后一个。 因此,Execute调用DeleteAllDoors,搜索项目中的所有门并删除。调用DeleteAllDoors功能时, 它调用DeleteElement函数。
如果你想要了解每行代码的详细信息,请参阅我以前关于RevitAPI 的课程。
using System;
using System.IO;
using System.Collections.Generic;
using Autodesk.Revit.ApplicationServices;
using Autodesk.Revit.DB;
using Autodesk.Revit.UI;
namespace DeleteDoors
{
[Autodesk.Revit.Attributes.Regeneration(Autodesk.Revit.Attributes.RegenerationOption.Manual)]
[Autodesk.Revit.Attributes.Transaction(Autodesk.Revit.Attributes.TransactionMode.Manual)]
public class DeleteDoorsApp : IExternalCommand
{
public Result Execute(ExternalCommandData commandData, ref string message, ElementSet elements)
{
var app = commandData.Application.Application;
var doc = commandData.Application.ActiveUIDocument?.Document;
DeleteAllDoors(app, doc);
return Result.Succeeded;
}
public static void DeleteElement(Document document, Element element)
{
// Delete a selected element
ICollection<Autodesk.Revit.DB.ElementId> deletedIdSet = document.Delete(element.Id);
if (0 == deletedIdSet.Count)
{
throw new Exception("Deleting the selected element in Revit failed.");
}
String prompt = "The selected element has been removed and ";
prompt += deletedIdSet.Count - 1;
prompt += " more dependent elements have also been removed.";
// Give the user some information
TaskDialog.Show("Revit", prompt);
}
public static void DeleteAllDoors(Application rvtApp, Document doc)
{
if (rvtApp == null) throw new InvalidDataException(nameof(rvtApp));
if (doc == null) throw new InvalidOperationException("Could not open document.");
IList<Element> listOfDoors = new FilteredElementCollector(doc).OfCategory(BuiltInCategory.OST_Doors).WhereElementIsNotElementType().ToElements();
int taille = listOfDoors.Count;
if (taille == 0)
{
TaskDialog.Show("Dialog", "There isn't any door in the project");
}
else
{
using (Transaction tr = new Transaction(doc))
{
tr.Start("do");
for (int i = 0; i < taille; i++)
{
DeleteElement(doc, listOfDoors[i]);
}
tr.Commit();
}
}
}
}
}
4、插件Manifest
插件manifest使用 XML 创建,它 Revit能够识别扩展命令或应用程序。
<?xml version="1.0" encoding="utf-8"?>
<RevitAddIns>
<AddIn Type="Command">
<Name>Delete Doors</Name>
<Assembly>.\Autodesk.DeleteDoors.Command.dll</Assembly>
<Text>Delete Doors</Text>
<AddInId>50930344-E64B-4AF6-9AD8-3D23311D4B56</AddInId>
<FullClassName>DeleteDoors.DeleteDoorsApp</FullClassName>
<Description>"Deletes Doors"</Description>
<VendorId>ADSK</VendorId>
<VendorDescription>
https://forge.autodesk.com/en/docs/design-automation/v3/tutorials/revit/
</VendorDescription>
</AddIn>
</RevitAddIns>
有两种方法可以将插件manifest保存到其目录中:手动创建.txt文件,然后使用扩展.addin将其保存, 然后通过向VS解决方案添加配置文件并编写命令行自动保存(正如我在上面解释的那样)。
将”DeleteDoors.addin”的Copy Local属性设置为”Copy if newer”。如果插件已修改或目录中不存在, 此属性可将插件复制到正确的位置。
5、PackageContents配置
为了添加.XML 文件到包内容,单击解决方案并添加新项目。选择”XML 文件”并将其重命名 为”PackageContents”,并将下面代码复制进文件。
<?xml version="1.0" encoding="utf-8" ?>
<ApplicationPackage>
<Components Description="Delete Doors">
<RuntimeRequirements OS="Win64"
Platform="Revit"
SeriesMin="R2021"
SeriesMax="R2021" />
<ComponentEntry AppName="DeleteDoors"
Version="1.0.0"
ModuleName="./DeleteDoors.addin"
AppDescription="Deletes all doors"
LoadOnCommandInvocation="False"
LoadOnRevitStartup="True" />
</Components>
</ApplicationPackage>
6、测试DeleteDoors插件
我们已经完成了我们的Revit插件项目,现在是测试它的时候了。单击”开始”或按”F5”以启动Revit应用。 Revit打开后,当看到弹出窗口时,请单击”始终加载”按钮。
如果你查看Add-Ins>External Tools,会看到DeleteDoors插件已加载。
尝试创建一个带墙壁和门的项目,并执行DeleteDoors插件:
如果你尝试执行插件,它将删除门,然后你会得到这个:
果你继续执行该插件,会得到提示消息,指出当前项目中没有任何门。
外部命令:是加载插件到外部工具工具栏的声明界面:
原文链接:Revit插件开发入门 — BimAnt
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK