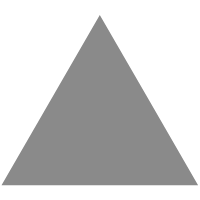
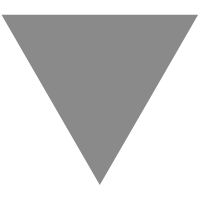
How to Generate an Ansible Inventory with Terraform
source link: https://www.percona.com/blog/2021/06/01/how-to-generate-an-ansible-inventory-with-terraform/
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
Creating and maintaining an inventory file is one of the common tasks we have to deal with when working with Ansible. When dealing with a large number of hosts, it can be complex to handle this task manually.
There are some plugins available to automatically generate inventory files by interacting with most cloud providers’ APIs. In this post, however, I am going to show you how to generate and maintain an inventory file directly from within the Terraform code.
To illustrate this, we will generate an Ansible inventory file to deploy a MongoDB sharded cluster on the Google Cloud Platform. If you want to know more about Ansible provisioning, you might want to check out my talk at Percona Live Online 2021.
The Ansible Inventory
The format of the inventory file is closely related to the actual Ansible code that is used to deploy the software. The structure we need is defined as follows:
We have to specify groups for each shard’s replicaset (shardN), as well as the config servers (cfg) and mongos routers (mongos).
The mongodb_primary tag is used to designate a primary server by giving it higher priority in the replicaset configuration.
Now that we know the structure we need, let’s start by provisioning our hardware resources with Terraform.
Creating Instances in GCP
Here is an example of a Terraform script to generate some instances for the shards. The Terraform count directive is used to create many copies of a given resource, so we define it dynamically.
The above code shuffles the instances through the available zones within a region for high availability. As you can see, there are some expressions used to generate the name and the labels. I will get to this shortly. We can use similar scripts for creating the instances for the config servers and the mongos nodes.
This is an example of the variables file:
Identifying the Resources
We need an easy way to identify our resources to work with them effectively. One way is to define labels on our Terraform code that is used to create the instances. We could also use instance tagging instead.
For the shards, the key part is that we need to generate a group for each shard dynamically, depending on how many shards we are provisioning. We need some extra information:
- the group names (i.e. shard0, shard1) for each host
- which member within a group we are working with
We define two labels for the purpose of iterating through the resources:
For the ansible-group, the variable shardsvr_replicas defines how many members each shard’s replica set has (e.g. 3). So for 2 shards, the expression above gives us the following output:
These values will be useful for matching each host with the corresponding group.
Now let’s see how to generate the list of servers per group. For the ansible-index, the expression gives us the following output:
For mongos and config servers, it is easier:
So with the above, we should have every piece of information we need.
Creating an Output File
Terraform provides the local_file resource to generate a file. We will use this to render our inventory file, based on the contents of the inventory.tmpl template.
We have to pass the values we need as arguments to the template, as it is not possible to reference outside variables from within it. In addition to the counters we had defined, we need the actual hostnames and the total number of shards. This is what it looks like:
The output will be a file called inventory, stored on the machine where we are running Terraform.
Template Generation
On the template, we need to loop through the different groups and print the information we have in the proper format.
To figure out whether to print the mongodb_primary attribute, we test the loop index for the first value and print the empty string otherwise. For the actual shards, we can generate the group name easily using our previously defined variable, and check if a host should be included in the group.
Anything between %{} contains a directive, while the ${} are used to substitute the arguments we fed the template. The ~ is used to remove the extra newlines.
Final Words
Terraform and Ansible together is a powerful combination for infrastructure provisioning and management. We can automate everything from hardware deployment to software installation.
The ability to generate a file from Terraform is quite handy for the purpose of creating our Ansible inventory. Since it is responsible for the actual provisioning, Terraform has all the information we need, although it can be a bit tricky to get the templating right.
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK