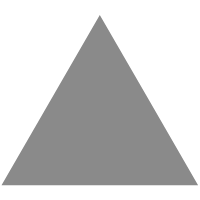
4
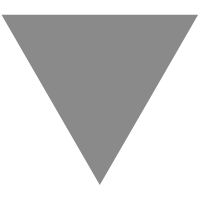
从零开始学Python - 02.容器类型
source link: https://blog.mute-g.com/post/python/container.html
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
- 了解列表、元组、字典与集合等容器类型
- 了解各容器类型的常用操作
- 列表可以包含零个或多个元素,元素间用逗号隔开,整个列表被方括号包裹。
- 空列表可以用
[]
或list()
创建。 - 列表中的各个元素的类型并不要求一致。
列表的基本操作
- 使用
[offset]
提取列表中的元素,并可以通过赋值对它进行修改。 - 使用
[start:end:step]
切片。 - 使用
append()
方法添加单个元素至尾部:
appendList = ['A', 'B']
appendList.append('C')
print(appendList)
# 会输出 ['A', 'B', 'C']
- 使用
extend()
方法或者+=
操作符合并列表:
extendList = ['A', 'B']
extendList += ['C', 'D']
print(extendList)
# 会输出 ['A', 'B', 'C', 'D']
- 使用
insert()
方法在指定位置插入单个元素:
insertList = ['A', 'B']
insertList.insert(1, 'C')
print(insertList)
# 会输出 ['A', 'C', 'B']
- 使用
del
语句删除指定位置的元素:
delList = ['A', 'B', 'C']
del delList[1]
print(delList)
# 会输出 ['A', 'C']
- 使用
remove()
方法移除指定元素:
removeList = ['A', 'B', 'C']
removeList.remove('B')
print(removeList)
# 会输出 ['A', 'C']
- 使用
pop()
方法获取并移除指定位置的元素,如果不指定偏移量,则获取并移除末尾元素:
popList = ['A', 'B', 'C', 'D']
print(popList.pop())
# 会输出 D
print(popList)
# 会输出 ['A', 'B', 'C']
print(popList.pop(1))
# 会输出 B
print(popList)
# 会输出 ['A', 'C']
- 使用
index()
方法查询指定元素的位置:
indexList = ['A', 'B', 'C']
print(indexList.index('B'))
# 会输出 1
- 使用
in
语句判断指定元素是否存在于列表中:
inList = ['A', 'B', 'C']
print('B' in inList)
# 会输出 True
print('D' in inList)
# 会输出 False
- 使用
count()
方法查询指定元素在列表中出现的次数:
countList = ['A', 'B', 'C', 'B']
print(countList.count('D'))
# 会输出 0
print(countList.count('C'))
# 会输出 1
print(countList.count('B'))
# 会输出 2
- 使用
sort()
方法重新排序列表中的元素:
sortList = ['B', 'A', 'C']
sortList.sort()
print(sortList)
# 会输出 ['A', 'B', 'C']
- 使用
sorted()
方法返回排序后的列表:
sortList = ['B', 'A', 'C']
sortedList = sorted(sortList)
print(sortList)
# 会输出 ['B', 'A', 'C']
print(sortedList)
# 会输出 ['A', 'B', 'C']
- 使用
len()
方法返回列表长度:
lenList = ['B', 'A', 'C']
print(len(lenList))
# 会输出 3
- 使用
copy()
方法返回列表的一个副本:
sortList = ['B', 'A', 'C']
copyList = sortList.copy()
sortList.sort()
print(sortList)
# 会输出 ['A', 'B', 'C']
print(copyList)
# 会输出 ['B', 'A', 'C']
- 使用
()
创建元组。 - 元组内的每一个元素后面都要跟着一个逗号。
- 如果创建的元组所包含的元素数量超过1,则最后一个元素后面的逗号可以省略。
emptyTuple = ()
print(emptyTuple)
# 会输出 ()
oneTuple = ('a',)
print(oneTuple)
# 会输出 ('a',)
normalTuple = ('a', 'b', 'c')
print(normalTuple)
# 会输出 ('a', 'b', 'c')
- 可以将元组内的元素同时分别赋值给都多个变量,这称为“元组解包”。
normalTuple = ('A', 'B', 'C')
print(normalTuple)
# 会输出 ('A', 'B', 'C')
a, b, c = normalTuple
print(a, b, c)
# 会输出 A B C
元组与列表
很多时候都可以用元组代替列表,它们的主要区别是:
- 但由于元组无法修改,所以元组没有
append()
等方法。 - 元组占用的空间较小。
- 可以将元组用作字典的“键”。
- 命名元组可以作为对象的替代。
- 方法的参数是以元组形式传递的(后续文章会介绍)。
- 使用
{}
与被它包含起来的一系列键值对创建字典,键值对之间使用逗号隔开。
emptyDict = {}
print(emptyDict)
# 会输出 {}
normalDict = {'A': 'aaa', 'B': 'bbb'}
print(normalDict)
# 会输出 {'A': 'aaa', 'B': 'bbb'}
注意 字典的键是无序的。
- 使用
[key]
操作符添加或者修改元素。
modifyDict = {'A': 'aaa', 'B': 'bbb'}
modifyDict['A'] = 'aaaA'
modifyDict['C'] = 'ccc'
print(modifyDict)
# 会输出 {'A': 'aaaA', 'B': 'bbb', 'C': 'ccc'}
- 使用
update()
方法合并字典。
updateDict = {'A': 'aaa', 'B': 'bbb'}
updateDict.update({'C': 'ccc', 'B': 'bbbB'})
print(updateDict)
# 会输出 {'A': 'aaa', 'B': 'bbbB', 'C': 'ccc'}
- 使用
clear()
方法删除所有元素。
clearDict = {'A': 'aaa', 'B': 'bbb'}
clearDict.clear()
print(clearDict)
# 会输出 {}
- 使用
del
语句删除具有指定key
的元素。 - 使用
in
语句判断指定key
是否存在于一个字典中。 - 使用
[key]
操作符获取具有指定key
的元素。 - 使用
copy()
方法将字典复制到一个新的字典中。 - 使用
keys()
方法获取字典中所有的键。 - 使用
values()
方法获取字典中所有的值。 - 使用
items()
方法获取字典中所有的键值对,每一个键值对以元组的形式返回。
注意 在 Python2
里上述三个方法会返回一个列表;而在 Python3
里则会返回一个迭代器,如果需要一个列表,需要自行利用 list()
方法进行类型转换。
keysDict = {1: '111', '2': 222}
print(keysDict.keys())
# 会输出 dict_keys([1, '2'])
print(keysDict.values())
# 会输出 dict_values(['111', 222])
print(keysDict.items())
# 会输出 dict_items([(1, '111'), ('2', 222)])
- 使用
{}
与被它包含起来的一系列值创建集合,值之间使用逗号隔开。
normalSet = {0, 1, 2, 3, 4, 5, 6}
print(normalSet)
# 会输出 {0, 1, 2, 3, 4, 5, 6}
注意 集合是无序的。
- 使用
set()
方法创建一个空集合。
emptySet = set()
print(emptySet)
# 会输出 set()
注意 为什么使用 set()
而不是 {}
?没有什么特殊原因,只是因为大括号已经被 字典
使用了。
- 使用
in
语句判断指定值是否存在于一个集合中。
- 使用
&
操作符或者intersection()
方法获取集合的交集。 - 使用
|
操作符或者union()
方法获取集合的并集。 - 使用
-
操作符或者difference()
方法获取集合的差集。 - 使用
^
操作符或者symmetric_difference()
方法获取集合的异或集。 - 使用
<=
操作符或者issubset()
方法判断一个集合是否是另一个集合的子集。 - 使用
<
操作符判断一个集合是否是另一个集合的真子集。 - 使用
>=
操作符或者issuperset()
方法判断一个集合是否是另一个集合的超集。 - 使用
>
操作符判断一个集合是否是另一个集合的真超集。
print(aSet <= abSet)
print(aSet.issubset(abSet))
# 会输出 True
print(abSet <= abSet)
# 会输出 True
print(abSet < abSet)
# 会输出 False
print(abSet >= bSet)
print(abSet.issuperset(bSet))
# 会输出 True
print(abSet >= abSet)
# 会输出 True
print(abSet > abSet)
# 会输出 False
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK