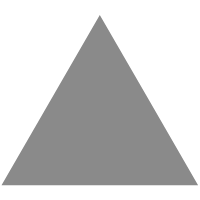
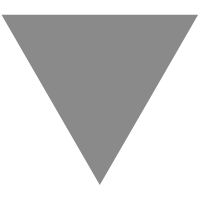
Collection static factory methods in Java 9
source link: https://blog.knoldus.com/collection-static-factory-methods-in-java-9/
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
Collection static factory methods in Java 9
Reading Time: 3 minutes
Hi folks, I hope you are doing good. Today, we came up with a new blog in which we will talk about which factory methods are included in java 9 so let’s start.
Java 9 comes with some static factory methods in Map, Set, and List Interface. The factory methods are super helpful when we are using collections. I will show you both ways how we were doing the things before the factory methods came into existence and after they came into existence. Following are the static factory methods introduced in Java 9 –
- List.of
- Map.of
- Set.of
List.of
If we want to create a list of 4 elements then how the code will look like –
List<String> list = new ArrayList<>();
list.add("Java");
list.add("Scala");
list.add("Go");
list.add("Rust");
for(String elements : list){
System.out.println(elements); // Java,Scala,Go,Rust
}
The above code snippet creates the mutable List but if we want to create immutable List before release of Java 9 then the code will look something like this –
List<String> list = new ArrayList<>(Arrays.asList("Java", "Rust", "Rego"));
List<String> unmodifiableList = Collections.unmodifiableList(list);
// Case 1
for(String elements:unmodifiableList) {
System.out.println(elements); // Java,Rust,Rego
}
// Case 2
unmodifiableList.add("Scala");
for(String elements:unmodifiableList) {
System.out.println(elements); // java.lang.UnsupportedOperationException
}
// Case 3
list.add("Scala");
for(String elements:unmodifiableList) {
System.out.println(elements); // Java,Rust,Rego,Scala
}
If we try to add more elements in unmodifiableList then code will throw UnsupportedOperationException But if we add some elements in list then it will not throw any exception So from here we understand there is big difference b/w Collections.unmodifiableList and immutable. Actually, An unmodifiable collection is just a wrapper around a modifiable collection so we can’t make any change to it if we only have a reference to an unmodifiable collection but if others have a reference to the list then we can add more elements to it. Results, No guarantee that nothing can change the collection. whereas the immutable collection guarantee that nothing can change the collection.
we can create immutable collections in only one single line by using List.of() factory method in java 9. We can specify any number of elements in List.of() while creating List collection.
List<String> list = List.of("Java","Scala","Go","Rust");
for(String elements:list) {
System.out.println(elements); // Java,Scala,Go,Rust
}
List.of() factory method creates an immutable list and if we use the add() function to add more elements after using List.of() then it will throw an exception.
List<String> list = List.of("Java","Scala","Go","C","RUST");
list.add("Extra_field");
for(String elements:list) {
System.out.println(elements);
}
Map.of
The Map.of() factory method used to create immutable Maps in java 9.
Map<Integer, String> langMap = Map.of(1, "Java", 2, "Scala", 3, "Rust");
langMap.forEach((k, v) -> System.out.println(k + " - " + v));
// output
1,Java
2,Scala
3,Rust
and if we try to add more key value entries in the langMap after using the factory method then it will throw java.lang.UnsupportedOperationException.
If we want to create immutable Map with immutable List then code will look like –
List<String> List1 = List.of("20");
List<String> List2 = List.of("22", "33");
Map<Integer, List<String>> listMap = Map.of(10, List1, 11, List2);
System.out.println(listMap);
// 10=[20],11=[22,33]
Here the listMap is immutable because if we use an immutable list to create the entries in the map but if we use mutable List then the newly created map will be immutable or mutable?
Let’s take a example –
List<String> list1 = new ArrayList<>();
list1.add("20");
List<String> list2 = new ArrayList<>();
list2.add("22");
Map<Integer, List<String>> map = Map.of(10, list1, 11, list2);
System.out.println(map); // 10=[20],11=[22]
list1.add("30");
System.out.println(map); // 10=[20,30],11=[22]
Results, the map is mutable as we are able to modify the entries in the map.
Set.of
The of() static method in set is the same as in map interface. There are some properties of set created using of() factory method.
- Set declared using of() static method will be immutable. If we try to add some more elements into the set it will throwUnsupportedOperationException.
- The null is not allowed as an element while declaring set by of() static method because of @NotNull notation used internally by java 9 otherwise it will give java.lang.NullPointerException.
- Other properties is same as described in list section.
Set<Integer> set = new HashSet<>();
set.add(11);
set.add(22);
set.add(null);
System.out.println(set); // [11,22,null]
Set<String> set1 = Set.of("11","22",null);
System.out.println(set1); // java.lang.NullPointerException
That’s all for this blog,we will come back with some other interesting topic. If you have any more queries or want to know more about it you can add the comment. I am happy to answer them.
References
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK