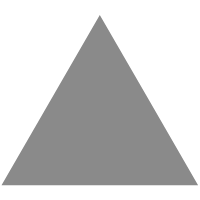
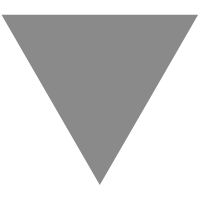
Creating a C# Client, and Listing, and Adding Data in MongoDB
source link: https://www.pmichaels.net/2021/05/22/creating-a-c-client-and-listing-and-adding-data-in-mongodb/?utm_campaign=creating-a-c-client-and-listing-and-adding-data-in-mongodb
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
In this previous post I wrote about how you can install MongoDB, and use the built in clients to interrogate the data. In this post I’ll cover setting up a simple C# client to interrogate the database.
Bring in a NuGet Package
As with many integrations in the last 10 years, 90% of the job is installing the right NuGet package:
Install-Package MongoDB.Driver
Once that’s installed, you should have access to the SDK. You’ll need the following using statement:
using MongoDB.Driver;
Connecting to the MonoDB Instance
It actually took me a while to work out the correct format here; for the default instance, you can simply use this for the connection string:
mongodb://localhost:27017
So to connect to the DB, you would use this:
MongoClient dbClient =
new
MongoClient(
"mongodb://localhost:27017"
);
var
db = dbClient.GetDatabase(
"testdb"
);
See the referenced previous post if you’re interested where testdb came from.
Collections
As we saw in the previous post, Mongo works around the concept of collections – it’s roughly analogous to a table. The list the collections:
MongoClient dbClient =
new
MongoClient(
"mongodb://localhost:27017"
);
var
db = dbClient.GetDatabase(
"testdb"
);
var
collections =
await
db.ListCollectionsAsync();
foreach
(
var
col
in
collections.ToList())
{
Console.WriteLine(col.ToString());
}
Let’s see what inserting data would look like.
Inserting Data
In fact, inserting data is very straightforward. We need to introduce a new type here, called a BsonDocument. BSON is a Binary JSON document, and to all intents and purposes, it’s JSON; this means that you can create a document such as this:
var
document =
new
BsonDocument()
{
{
"test"
,
"test1"
},
{
"test2"
,
"test2"
},
{
"test3"
,
"test3"
}
};
Or, indeed, any valid JSON document.
To insert this into the DB, you would just call InsertOne:
var
collection = db.GetCollection<BsonDocument>(
"testcollection"
);
await
collection.InsertOneAsync(document);
You can insert many records by calling InsertMany:
var
documents =
new
List<BsonDocument>()
{
new
BsonDocument()
{
{
"test"
,
"test1"
},
{
"test2"
,
"test2"
},
{
"test3"
,
"test3"
}
},
new
BsonDocument()
{
{
"Date"
, DateTime.Now },
{
"test2"
, 12 },
{
"test3"
,
"hello"
}
}
};
var
collection = db.GetCollection<BsonDocument>(
"testcollection"
);
await
collection.InsertManyAsync(documents);
References
https://www.mongodb.com/blog/post/quick-start-c-sharp-and-mongodb-starting-and-setup
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK