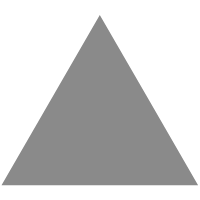
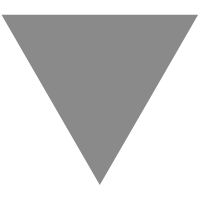
Spring MVC获取HTTP请求头的两种方式
source link: https://www.pkslow.com/archives/spring-mvc-get-headers
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
请求是任何Web
服务要关注的对象,而请求头也是其中非常重要的信息。本文将通过代码讲解如何在Spring MVC
项目中获取请求头的内容。主要通过两种方式获取:
(1)通过注解@RequestHeader
获取,需要在Controller
中显式获取;
(2)通过RequestContextHolder
获取,可以任何地方获取。
接下来通过代码讲解。
2 通过注解@RequestHeader获取
需要在Controller
中显示使用@RequestHeader
。
2.1 获取某个请求头
只获取其中一个请求头,相当容易,代码如下:
@GetMapping("/webSite")
public String webSite(@RequestHeader("webSite")String webSite) {
return "The webSite is " + webSite;
}
这里获取的是webSite
这个请求头,测试如下:
$ curl http://localhost:8088/header/webSite -H 'webSite: www.pkslow.com'
The webSite is www.pkslow.com
我们查看@RequestHeader
的源码,它还有其它属性,如下所示:
public @interface RequestHeader {
@AliasFor("name")
String value() default "";
@AliasFor("value")
String name() default "";
boolean required() default true;
String defaultValue() default "\n\t\t\n\t\t\n\ue000\ue001\ue002\n\t\t\t\t\n";
}
2.1.1 属性required
required
属性默认为true
,如果不配置而客户端没有传递该请求头,会有报错。如果配置为false
且没有请求头,值为null
。代码如下:
@GetMapping("/webSite-not-required")
public String webSiteNotRequired(@RequestHeader(value = "webSite", required = false)String webSite) {
return "The webSite is " + webSite;
}
测试结果如下:
$ curl http://localhost:8088/header/webSite-not-required -H 'webSite: www.pkslow.com'
The webSite is www.pkslow.com
$ curl http://localhost:8088/header/webSite-not-required
The webSite is null
2.1.2 属性defaultValue
defaultValue
更容易理解,通过它来设置默认值。如果有传递则获取客户端传递的值,如果没有传递为默认值。代码如下:
@GetMapping("/webSite-defaultValue")
public String webSiteDefaultValue(@RequestHeader(value = "webSite", defaultValue = "pkslow.com")String webSite) {
return "The webSite is " + webSite;
}
测试结果如下:
$ curl http://localhost:8088/header/webSite-defaultValue -H 'webSite: www.pkslow.com'
The webSite is www.pkslow.com
$ curl http://localhost:8088/header/webSite-defaultValue
The webSite is pkslow.com
2.2 获取所有请求头
注解@RequestHeader
除了可以获取某个请求头,还可以一次性获取所有请求头,这时不需要指定名字。可以通过Map
、MultiValueMap
和HttpHeaders
来获取所有请求头的值,示例代码如下:
@GetMapping("/allMap")
public Map<String, String> allMap(@RequestHeader Map<String, String> headers) {
return headers;
}
@GetMapping("/allMultiValueMap")
public Map<String, String> allMultiValueMap(@RequestHeader MultiValueMap<String, String> headers) {
return headers.toSingleValueMap();
}
@GetMapping("/allHttpHeaders")
public String allHttpHeaders(@RequestHeader HttpHeaders headers) {
return headers.toString();
}
测试如下:
$ curl http://localhost:8088/header/allMap -H 'Authorization: Basic cGtzbG93OjEyMzQ1Ng==' -H 'webSite: www.pkslow.com'
{"host":"localhost:8088","user-agent":"curl/7.64.1","accept":"*/*","authorization":"Basic cGtzbG93OjEyMzQ1Ng==","website":"www.pkslow.com"}
$ curl http://localhost:8088/header/allMultiValueMap -H 'Authorization: Basic cGtzbG93OjEyMzQ1Ng==' -H 'webSite: www.pkslow.com'
{"host":"localhost:8088","user-agent":"curl/7.64.1","accept":"*/*","authorization":"Basic cGtzbG93OjEyMzQ1Ng==","website":"www.pkslow.com"}
$ curl http://localhost:8088/header/allHttpHeaders -H 'Authorization: Basic cGtzbG93OjEyMzQ1Ng==' -H 'webSite: www.pkslow.com'
[host:"localhost:8088", user-agent:"curl/7.64.1", accept:"*/*", authorization:"Basic cGtzbG93OjEyMzQ1Ng==", website:"www.pkslow.com"]
3 通过RequestContextHolder获取
通过RequestContextHolder
来获取则可以在其它层获取。它先获取Request
对象,再获取请求头。代码如下:
@GetMapping("/webSite-RequestContextHolder")
public String webSiteRequestContextHolder() {
ServletRequestAttributes requestAttributes = (ServletRequestAttributes)RequestContextHolder.getRequestAttributes();
// get the request
HttpServletRequest request = requestAttributes.getRequest();
return "The webSite is " + request.getHeader("webSite");
}
测试如下:
$ curl http://localhost:8088/header/webSite-RequestContextHolder -H 'webSite: www.pkslow.com'
The webSite is www.pkslow.com
以上两个方法,对于WebFlux
而言,通过注解@RequestHeader
获取是可行的;通过RequestContextHolder
获取则不行,可参考【在Spring WebFlux的任何地方获取Request对象】。
代码请查看:https://github.com/LarryDpk/pkslow-samples
欢迎关注微信公众号<南瓜慢说>,将持续为你更新...
推荐阅读:
如何制定切实可行的计划并好好执行
容器技术(Docker-Kubernetes)
SpringBoot-Cloud相关
Https专题
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK