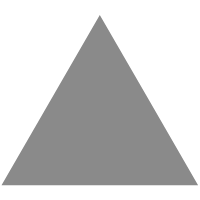
3
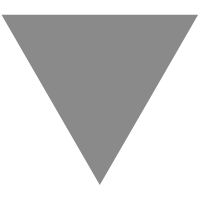
Can I somehow get my program to convert characters to integers?
source link: https://www.codesd.com/item/can-i-somehow-get-my-program-to-convert-characters-to-integers.html
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
Can I somehow get my program to convert characters to integers?
advertisements
I have been running this and typing "12+"
as the expression. 'm down to trying to add the top value and next value and it keeps giving me the result 'c'
but I want the result to be 3. So is there any way to get my program to convert char 'c' to int '3' and char 'd' to int 4 and so on ?
//array based stack implementation
class Stack
{
private:
int capacity; //max size of stack
int top; //index for top element
char *listArray; //array holding stack elements
public:
Stack (int size = 50){ //constructor
capacity = size;
top = 0;
listArray = new char[size];
}
~Stack() { delete [] listArray; } //destructor
void push(char it) { //Put "it" on stack
listArray[top++] = it;
}
char pop() { //pop top element
return listArray [--top];
}
char& topValue() const { //return top element
return listArray[top-1];
}
char& nextValue() const {//return second to top element
return listArray[top-2];
}
int length() const { return top; } //return length
};
int main()
{
string exp;
char it = ' ';
int count;
int push_length;
cout << "Enter an expression in postfix notation:\n";
cin >> exp;
cout << "The number of characters in your expression is " << exp.length() << ".\n";
Stack STK;
for(count= 0; count < exp.length() ;count++)
{
if (exp[count] == '+')
{
it = exp[count - 1];
cout << it << "?\n";
while (!isdigit(it))
{
cout << it << "!\n";
it = exp[count--];
}
STK.push(it);
cout << STK.topValue() << "\n";
it = exp[count - 2];
cout << it << "\n";
if (isdigit(it))
{
STK.push(it);
}
cout << STK.topValue() << "\n";
cout << STK.nextValue() << "\n";
it = STK.topValue() + STK.nextValue();
cout << it << "\n";
STK.pop();
STK.pop();
STK.push(it);
cout << STK.topValue() << "\n";
}
}
cout << "The number of characters pushed into the stack is " << STK.length() << ".\n";
push_length = STK.length();
return(0);
}
You can simply use something like STK.push(it-'0') instead of STK.push(it).
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK