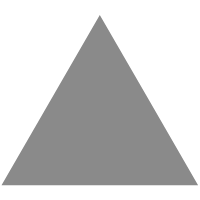
4
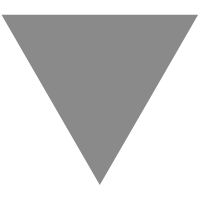
How to Use XMPP/Jabber with PHP
source link: https://www.devdungeon.com/content/how-use-xmppjabber-php
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
XMPP/Jabber is a communication protocol typically used for chat servers. Nathan Fritz wrote a PHP library for XMPP called XMPPHP. These examples demonstrate how to use the library in PHP.
Sending a Message
include("xmpp.php");
$conn = new XMPP(
'talk.google.com',
5222,
'username',
'password',
'xmpphp',
'gmail.com',
);
//$conn->use_encryption = false; // Optional
$conn->connect();
$conn->processUntil('session_start');
$conn->message('[email protected]', 'This is a test message!');
$conn->disconnect();
Command Line Bot
include("xmpp.php");
$conn = new XMPP(
'talk.google.com',
5222,
'username',
'password',
'xmpphp',
'gmail.com',
);
$conn->connect();
while (!$conn->disconnected) {
$payloads = $conn->processUntil(array(
'message',
'presence',
'end_stream',
'session_start'
));
foreach($payloads as $event) {
$pl = $event[1];
switch ($event[0]) {
case 'message':
print "-----------------------------------------------------\n";
print "Message from: {$pl['from']}\n";
if ($pl['subject']) {
print "Subject: {$pl['subject']}\n";
}
print $pl['body'] . "\n";
print "-----------------------------------------------------\n";
$conn->message(
$pl['from'],
$body = "Thanks for sending me "{$pl['body']}".",
$type = $pl['type']);
if ($pl['body'] == 'quit') {
$conn->disconnect();
}
if ($pl['body'] == 'break') {
$conn->send("</end>");
}
break;
case 'presence':
print "Presence: {$pl['from']} [{$pl['show']}] {$pl['status']}\n";
break;
case 'session_start':
$conn->presence($status = "Woo!");
break;
}
}
}
Web Client
session_start();
header('content-type', 'plain/text');
include 'XMPPHP/BOSH.php';
print "<pre>";
$conn = new XMPPHP_BOSH(
'server.tld',
5280,
'user',
'password',
'xmpphp',
'server.tld',
$printlog = true,
$loglevel = XMPPHP_Log::LEVEL_INFO
);
$conn->autoSubscribe();
try {
if(isset($_SESSION['messages'])) {
foreach($_SESSION['messages'] as $msg) {
print $msg;
flush();
}
}
$conn->connect('http://server.tld:5280/xmpp-httpbind', 1, true);
while(true) {
$payloads = $conn->processUntil(array(
'message',
'presence',
'end_stream',
'session_start'
));
foreach($payloads as $event) {
$pl = $event[1];
switch($event[0]) {
case 'message':
if (!isset($_SESSION['messages'])) {
$_SESSION['message'] = array();
}
$msg = "-----\n{$pl['from']}: {$pl['body']}\n";
print $msg;
$_SESSION['messages'][] = $msg;
flush();
$conn->message(
$pl['from'],
$body = "Thanks for sending me "{$pl['body']}".",
$type = $pl['type']
);
if ($pl['body'] == 'quit') {
$conn->disconnect();
}
if ($pl['body'] == 'break') {
$conn->send("</end>");
}
break;
case 'presence':
print "Presence: {$pl['from']} [{$pl['show']}] {$pl['status']}\n";
break;
case 'session_start':
print "Session Start\n";
$conn->getRoster();
$conn->presence($status = "Woo!");
break;
}
}
}
} catch (XMPPHP_Exception $e) {
die($e->getMessage());
}
$conn->saveSession();
print "</pre>";
print "<img src='http://xmpp.org/images/xmpp.png' onload='window.location.reload()' />";
?>
Command Line Client (XMPP)
<?php
include 'XMPPHP/XMPP.php';
$conn = new XMPP(
'talk.google.com',
5222,
'username',
'password',
'xmpphp',
'gmail.com',
);
$conn->autoSubscribe();
$vcard_request = array();
try {
$conn->connect();
while (!$conn->isDisconnected()) {
$payloads = $conn->processUntil(array(
'message',
'presence',
'end_stream',
'session_start',
'vcard'
));
foreach($payloads as $event) {
$pl = $event[1];
switch($event[0]) {
case 'message':
print "-------------------------------------------------\n";
print "Message from: {$pl['from']}\n";
if ($pl['subject']) print "Subject: {$pl['subject']}\n";
print $pl['body'] . "\n";
print "-------------------------------------------------\n";
$conn->message(
$pl['from'],
$body = "Thanks for sending me "{$pl['body']}".",
$type=$pl['type']
);
$cmd = explode(' ', $pl['body']);
if ($cmd[0] == 'quit') $conn->disconnect();
if ($cmd[0] == 'break') $conn->send("</end>");
if ($cmd[0] == 'vcard') {
if(!($cmd[1])) $cmd[1] = $conn->user . '@' . $conn->server;
$vcard_request[$pl['from']] = $cmd[1];
$conn->getVCard($cmd[1]);
}
break;
case 'presence':
print "Presence: {$pl['from']} [{$pl['show']}] {$pl['status']}\n";
break;
case 'session_start':
print "Session Start\n";
$conn->getRoster();
$conn->presence($status = "Woo!");
break;
case 'vcard':
// Check to see who requested this vcard
$deliver = array_keys($vcard_request, $pl['from']);
// Work through the array to generate a message
print_r($pl);
$msg = '';
foreach($pl as $key => $item) {
$msg .= "$key: ";
if (is_array($item)) {
$msg .= "\n";
foreach ($item as $subkey => $subitem) {
$msg .= " $subkey: $subitem\n";
}
} else {
$msg .= "$item\n";
}
}
// Deliver the vcard msg to everyone that requested that vcard
foreach ($deliver as $sendjid) {
// Remove the note on requests as we send out the message
unset($vcard_request[$sendjid]);
$conn->message($sendjid, $msg, 'chat');
}
break;
}
}
}
} catch(XMPPHP_Exception $e) {
die($e->getMessage());
}
Command Line Client (BOSH)
<?php
$conn = new XMPP(
'talk.google.com',
5222,
'username',
'password',
'xmpphp',
'gmail.com',
);
$conn->autoSubscribe();
try {
$conn->connect('http://server.tld:5280/xmpp-httpbind');
while (!$conn->isDisconnected()) {
$payloads = $conn->processUntil(array(
'message',
'presence',
'end_stream',
'session_start'
));
foreach ($payloads as $event) {
$pl = $event[1];
switch($event[0]) {
case 'message':
print "-------------------------------------------------\n";
print "Message from: {$pl['from']}\n";
if ($pl['subject']) print "Subject: {$pl['subject']}\n";
print $pl['body'] . "\n";
print "-------------------------------------------------\n";
$conn->message(
$pl['from'],
$body = "Thanks for sending me "{$pl['body']}".",
$type = $pl['type']
);
if ($pl['body'] == 'quit') $conn->disconnect();
if ($pl['body'] == 'break') $conn->send("</end>");
break;
case 'presence':
print "Presence: {$pl['from']} [{$pl['show']}] {$pl['status']}\n";
break;
case 'session_start':
print "Session Start\n";
$conn->getRoster();
$conn->presence($status="Cheese!");
break;
}
}
}
} catch (XMPPHP_Exception $e) {
die($e->getMessage());
}
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK