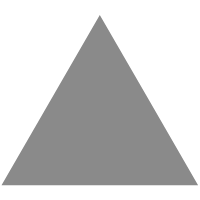
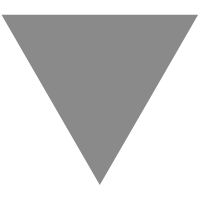
Lightning & thunder: Arduino Halloween DIY project
source link: https://oneguyoneblog.com/2017/11/01/lightning-thunder-arduino-halloween-diy/
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
Lightning & thunder: Arduino Halloween DIY project

For this year’s Halloween, I wanted to do some fun DIY projects with stuff I already had laying around. This project is about simulating the lightning and thunder of a thunderstorm with an Arduino, LEDs and a DFPlayer Mini MP3 player module (see the documentation). It is easy to build and it worked out really well. So here it is, for anyone who also wants to surprise (or scare) his/her guests next year.
Note that this is not a “sound to light” system. The sketch (randomly) flashes the LEDs first and then plays the sound file after a short (random) delay. I think that’s more realistic than flashing the LEDs in reaction to the volume of the sound like other projects/systems do. Light travels much faster than sound. Therefore, during a real-life thunderstorm, you will usually observe the flashes first. The sound will be delayed, depending on how far away the lightning occurred.
Lightning simulator project: parts list
The parts used for the project:
Of course, you can use some other 12V LED lights. The MP3 files (see below) are only about 6MB so any SD card will do.
Lightning and thunder Arduino Halloween DIY project: DFPLayer Mini MP3 moduleWiring the Arduino lightning simulator
The wiring is pretty straightforward, see the images below:
- The 12V power supply is connected to the red breadboard power rail on the breadboard.
The LEDs get 12V from the power rail (red wire).
The Arduino Nano gets 12V power from the power rail to its Vin pin (red wire) and the Nano GND pin is connected to the common ground rail (blue wire). - The DFPlayer Mini gets 5V on its VCC pin from the Nano (red wire).
The RX pin from the DFPlayer goes to pin 10 on the Nano (black wire) via a 1K resistor.
The TX pin from the DFPlayer goes to pin 11 on the Nano (purple wire) via a 1K resistor.
The BUSY pin from the DFPlayer goes to pin 12 on the Nano (white wire).
The DAC-L (green wire) and DAC-R (yellow wire) go to the stereo audio amplifier.
The GND pin from the DFPlayer goes to the common ground rail (blue wire). - The MOSFET GATE pin (left) goes to the Nano pin 9 (orange wire).
The MOSFET DRAIN pin (center) goes to the LED’s ground wire (black wire).
The MOSFET SOURCE pin (right) goes to the common ground rail (black wire). - The amplifier’s ground is connected to the common ground rail.
Make sure that the power supply, Arduino, MOSFET, DFplayer and audio connection share the same common ground. Also, make sure the 12V is connected to the right pin (Vin) on the Nano.
Make sure you have the polarization of 12V and ground of the power supply and the LEDs right.
I used some wires with alligator clips to easily connect the LEDs and audio to the breadboard.
Lightning and thunder-Arduino Halloween DIY project: wiringLightning and thunder sound files
I have collected 17 different lightning and thunder sound samples in a .zip file. They can be downloaded using the link below. The files (0001.mp3 to 0017.mp3) should be put in a folder called ‘mp3’ on the SD card. The card may be formatted FAT16 or FAT32.
Programming the Arduino
You can upload the sketch to the Arduino the usual way, using the USB cable. The Nano gets its power from the 12V power supply, not from USB. So after uploading you can disconnect the USB cable.
The sketch uses the DFRobotDFPlayerMini library, check out the GitHub page and documentation for details.
#include "Arduino.h" #include "SoftwareSerial.h" #include "DFRobotDFPlayerMini.h" int ledPin = 9; // LEDs (via MOSFET) connected to pin 9 int rxPin = 10; // DFplayer RX to Arduino pin 10 int txPin = 11; // DFplayer TX toArduinopin 11 int busyPin = 12; // DFplayer BUSY connected to pin 12 SoftwareSerial mySoftwareSerial(rxPin, txPin); DFRobotDFPlayerMini myDFPlayer; void setup() { pinMode(ledPin, OUTPUT); pinMode(busyPin, INPUT); mySoftwareSerial.begin(9600); Serial.begin(115200); Serial.println(F("Initializing DFPlayer...")); if (!myDFPlayer.begin(mySoftwareSerial)) { //Use softwareSerial to communicate with mp3. Serial.println(F("Unable to begin. Check connection and SD card, or reset the Arduino.")); while (true); } Serial.println(F("DFPlayer Mini online.")); myDFPlayer.setTimeOut(500); // Set serial communictaion time out 500ms myDFPlayer.volume(30); // Set volume value (0~30). myDFPlayer.EQ(DFPLAYER_EQ_BASS); // Set EQ to BASS (normal/pop/rock/jazz/classic/bass) myDFPlayer.outputDevice(DFPLAYER_DEVICE_SD); // Set device we use SD as default myDFPlayer.enableDAC(); // Enable On-chip DAC } void loop() { int flashCount = random (3, 15); // Min. and max. number of flashes each loop int flashBrightnessMin = 10; // LED flash min. brightness (0-255) int flashBrightnessMax = 255; // LED flash max. brightness (0-255) int flashDurationMin = 1; // Min. duration of each seperate flash int flashDurationMax = 50; // Max. duration of each seperate flash int nextFlashDelayMin = 1; // Min, delay between each flash and the next int nextFlashDelayMax = 150; // Max, delay between each flash and the next int thunderDelay = random (500, 3000); // Min. and max. delay between flashing and playing sound int thunderFile = random (1, 17); // There are 17 soundfiles: 0001.mp3 ... 0017.mp3 int loopDelay = random (5000, 30000); // Min. and max. delay between each loop Serial.println(); Serial.print(F("Flashing, count: ")); Serial.println( flashCount ); for (int flash = 0 ; flash <= flashCount; flash += 1) { // Flashing LED strip in a loop, random count analogWrite(ledPin, random (flashBrightnessMin, flashBrightnessMax)); // Turn LED strip on, random brightness delay(random(flashDurationMin, flashDurationMax)); // Keep it tured on, random duration analogWrite(ledPin, 0); // Turn the LED strip off delay(random(nextFlashDelayMin, nextFlashDelayMax)); // Random delay before next flash } Serial.print(F("Pausing before playing thunder sound, milliseconds: ")); Serial.println(thunderDelay); delay(thunderDelay); Serial.print(F("Playing thunder sound, file number: ")); Serial.println(thunderFile); myDFPlayer.playMp3Folder(thunderFile); delay(1000); // Give the DFPlayer some time while (digitalRead(busyPin) == LOW) { // Wait for the DFPlayer to finish playing the MP3 file } Serial.print(F("Pausing before next loop, milliseconds: ")); Serial.println(loopDelay); delay(loopDelay); }
DFPlayer Mini MP3 Player Module For Arduino
Banggood.com
65 Pcs Breadboard Jumper Connect Cable Adapter Cable
Banggood.com
8G Micro SD TF Micro SD Card For Cell Phone MP3 MP4 Camera
Banggood.com
DANIU 42cm 10pcs Alligator Clips Electrical DIY Test Leads
Banggood.com
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK