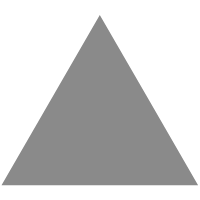
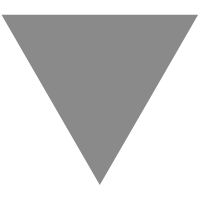
RESTful web service with Java EE (JAX-RS) and JSON: Hello World tutorial
source link: https://marco.dev/2016/02/05/restful-web-service-with-java-ee-jax-rs-and-json-beginners-tutorial/
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
You can find the java code here: https://github.com/marco76/simpleRestExample
For this example we use Netbeans 8.1 (with the new and nice darkula theme :)) that comes with Glassfish Server out-of-the-box.
The goal is to create and show the structure of a basic REST service in Java.
In the tutorial:
-
basic REST application with Java EE (no Spring)
-
response to a get with : text, list on JSON objects
-
simple integration test using Client
File structure
For this example we create 4 java classes (2 for the code, 1 for the unit test, 1 for the integration test).
Configuration
In RestConfig.java we tell to the server that there is a REST service and his address (endpoint).
import javax.ws.rs.ApplicationPath; import javax.ws.rs.core.Application; /** * * This class is used to tell to the server that there are REST services. * An alternative is to declare a servlet in the web.xml. * * @author marco */ @ApplicationPath("rest") // the 'rest' adress is mapped to the REST services public class RestConfig extends Application{ // a javax.ws.rs.core.Application must be extended }
We can configure the service with an annotation like in our example or declaring a Servlet in web.xml. In this example we don’t even create web.xml.
We leave the code of the class empty because we implements the methods in a second class: RestHelloWorld.java
Service class
Simple text answer
// @Provider tell the server that this is a REST class @Provider // @Path defines the path for this class: [server]/rest/examples @Path("examples") public class RestHelloWorld {
@Provider: tells to the server that this is a service class
@Path: part of the URL used to call the service
In this class we declare 1 method that return a simple text:
// path used to call the method: [server]/rest/examples/examples/helloWorld @Path("helloWorld") // answer only to a http get request @GET // return a simple string (text/plain by default) public String hello(){ return "Hello World"; // string to be returned }
@Path: is the url to be used to call the service. It is added to the server URL + @ApplicationPath + @Path of the class. The name of the method is not important.
@GET : reads the calls with the http get method (ex. url written directly in the address bar of the browser)
This method return a simple text (no HTML) that is shown in the browser:
Java List to JSON Array answer
The second method is more interesting because it return a list of String in JSON format
@Path("helloJSON") @GET @Produces("application/json") public List<String> helloJSONList(){ List<String> jsonList = new ArrayList<String>(); jsonList.add("Hello World"); jsonList.add("Bonjour monde"); return jsonList; }
@Produces: defines in which format the answer should be returned. It automatically transform our object (List of strings) in JSON format.
Netbeans recognize the services declared in this class and show them in the project structure:
To transform Java Objects in JSON and vice versa we need to import some converters in our project to avoid some MessageBodyWriter or MessageBodyReader media type error:
<!-- JSON support --> <!-- List to JSON --> <dependency> <groupId>com.sun.jersey</groupId> <artifactId>jersey-json</artifactId> <version>1.19</version> </dependency> <!-- JSON to List - MessageBodyReader media type --> <dependency> <groupId>org.glassfish.jersey.media</groupId> <artifactId>jersey-media-json-jackson</artifactId> <version>2.22.1</version> </dependency>
Integration Test
We can test our services directly using an explorer or, better, with some integration tests.
The class RestHelloWorldTestIT.java is used to test the REST service if it is up and running.
In the following code the test try to read the JSON list from the server converting it in a java String:
@Test public void testIntegrationHelloJSON(){ // the client connect to the REST service Client client = ClientBuilder.newClient(); List<String> helloWorldString = client.target(helloWorldURL+"helloJSON") // connection to the pre-defined URL .request() .get(ArrayList.class); // we call the 'get' method and we transform the answer in a String assertEquals(2, helloWorldString.size()); }
Because of the name convention Maven recognize that this is an integration test and it doesn’t execute it with the unit tests.
You need the failsafe plugin to profit from this feature:
<plugin> <groupId>org.apache.maven.plugins</groupId> <artifactId>maven-failsafe-plugin</artifactId> ...
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK