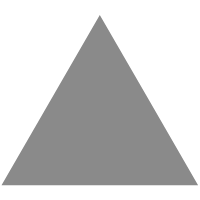
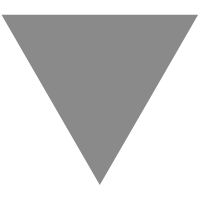
【译】值得推荐的十大React Hook 库
source link: http://www.cnblogs.com/sunny-lucky/p/13887307.html
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
十大React Hook库
- 原文地址: https://dev.to/bornfightcompany/top-10-react-hook-libraries-4065
- 原文作者:Juraj Pavlović
- 译者:培歌行(阳光是sunny)
React Hook来了,并在暴风雨中占领了React社区。自最初发布以来已经有一段时间了,这意味着有很多支持库。在搜索与React相关的内容时,很难不看到“ hook”这个词语。如果你还没有遇到的话,应该尽快将它们加入代码库学习起来。它们将使您的编码生活变得更加轻松和愉快。
在React开发中,保持干净的代码风格,可读性,可维护性,更少的代码行以及可重用性至关重要。该博客将向您展示应当被立即开始使用的 十大React Hook库 。不用再拖延了,让我们开始吧。
1.use-http
use-http
是一个非常有用的库,可用来替代Fetch API。它使您的编码更简单易懂,更精确地讲是数据操作部分。 use-http
本身使用TypeScript,甚至支持SSR和GraphQL。它返回响应,加载,错误数据和不同的请求方法,例如Get,Post,Put,Patch和Delete。
它提供的主要功能是:
- 请求/响应拦截器
- 暂停(目前处于实验状态)
- 重发功能
- 缓存
CodeSandbox示例和Youtube视频以及GitHub自述文件都对此进行了很好的记录。
用法示例:
import useFetch from "use-http" const Example = () => { const [todos, setTodos] = useState([]) const { get, post, response, loading, error } = useFetch("https://example.com") useEffect(() => { get("/todos") }, []) const addTodo = async () => { await post("/todos", { title: "example todo" }); if (response.ok) setTodos([...todos, newTodo]) } return ( <> <button onClick={addTodo}>Add Todo</button> {error && 'Error!'} {loading && 'Loading...'} {todos.map(todo => ( <span key={todo.id}>{todo.title}</span> )} </> ); };
2. useMedia
您是否需要一种跟踪CSS媒体查询的方法?useMedia提供一个简单的方法解决这个问题。这是一个能够准确跟踪的感官钩子。在任何应用程序或网站上,媒体查询以及响应能力都非常重要。
它以TypeScript编写。该软件包具有定义明确的文档,它的用法以及测试方法也解释的非常好。
用法示例:
import useMedia from 'use-media'; const Example = () => { const isWide = useMedia({minWidth: '1000px'}); return ( <span> Screen is wide: {isWide ? "WideScreen" : "NarrowScreen"} </span> ); };
3.Constate
Constate可将本地状态提升到React Context。这意味着可以以最小的努力轻松地将任何组件的任何状态提升到上下文。如果您想在多个位置使用相同的状态,或者为多个组件提供相同的状态,这很有用。该名称来自合并 Con text和 state 。
它是基于typescript实现的,而且库非常的小。虽然该文档不是很详细,但是看例子,我们就可以轻易地学会使用。
用法示例:
import React, { useState } from "react"; import constate from "constate"; // custom hook function useCounter() { const [count, setCount] = useState(0); const increment = () => setCount(prevCount => prevCount + 1); return { count, increment }; } // hook passed in constate const [CounterProvider, useCounterContext] = constate(useCounter); function Button() { // use the context const { increment } = useCounterContext(); return <button onClick={increment}>+</button>; } function Count() { // use the context const { count } = useCounterContext(); return <span>{count}</span>; } function App() { // wrap the component with provider return ( <CounterProvider> <Count /> <Button /> </CounterProvider> );
4. Redux hooks
Redux是许多(即使不是全部)React开发人员的知名工具。在整个应用程序中,它用作全局状态管理器。在React的最初版本发布几个月后,它就随hooks而出现了。它通过利用现有的connect()方法 提供了 一种 替代HOC(高阶组件)模式的方法。
提供的最著名的挂钩是:
- useSelector
- useDispatch
- useStore
该文档非常好,虽然有点复杂,但是它将为您提供开始使用它们所需的任何信息。
用法示例:
import {useSelector, useDispatch} from "react-redux"; import * as actions from "./actions"; const Example = () => { const dispatch = useDispatch() const counter = useSelector(state => state.counter) return ( <div> <span> {counter.value} </span> <button onClick={() => dispatch(actions.incrementCounter)}> Counter +1 </button> </div> ); };
5. React hook form
React hook form
是一个表单钩子库,类似于Formik和Redux表单,但是更好!凭借其更简单的语法,速度,更少的重新渲染数和更好的可维护性,它开始爬上GitHub的阶梯。
它的体积很小,并且是考虑到性能而构建起来的。该库甚至提供了非常棒的表单生成器!它是React钩子库中GitHub star数量最多的库之一(14.8k)。
用法示例:
import React from "react"; import { useForm } from "react-hook-form"; function App() { const { register, handleSubmit, errors } = useForm(); const onSubmit = (data) => { // logs {firstName:"exampleFirstName", lastName:"exampleLastName"} console.log(data); }; return ( <form onSubmit={handleSubmit(onSubmit)}> <input name="firstName" ref={register} /> <input name="lastName" ref={register({ required: true })} /> {errors.lastName && <span>"Last name is a required field."</span>} <input name="age" ref={register({ required: true })} /> {errors.age && <span>"Please enter number for age."</span>} <input type="submit" /> </form> ); }
6. useDebounce
useDebounce表示一个用于防抖的小钩子。它用于将功能的执行推迟到以后。常用于获取数据的输入框和表格中。
用法示例:
import React, { useState } from "react"; import { useDebounce } from "use-debounce"; export default function Input() { const [text, setText] = useState("Hello"); const [value] = useDebounce(text, 1000); return ( <div> <input defaultValue={"Hello"} onChange={(e) => { setText(e.target.value); }} /> <p>Value: {text}</p> <p>Debounced value: {value}</p> </div> ); }
7. useLocalStorage
useLocalStorage是一个小钩子,与上面的钩子一样。它对于在localStorage中提取和设置数据非常有用。使用它之后操作变得很容易。
提供自动JSON序列化和同步的功能
文档以高质量的方式编写,并且通过扩展示例可以完全理解。
用法示例:
import React, { useState } from "react"; import { writeStorage } from '@rehooks/local-storage'; export default function Example() { let counter = 0; const [counterValue] = useLocalStorage('counterValue'); return ( <div> <span>{counterValue}</span> <button onClick={() => writeStorage('i', ++counter)}> Click Me </button> </div> ); }
8. usePortal
usePortal使得创建下拉菜单,弹出层,通知弹出窗口,提示等变得非常容易!它提供了在应用程序的DOM层次结构之外创建元素的功能。
它还提供了门户样式和大量其他选项的完全定制。
编写的文档非常好,其中展示了许多示例,这些示例足够用于开始使用库/自己做钩子。
用法示例:
import React, { useState } from "react"; import usePortal from "react-useportal"; const Example = () => { const { ref, openPortal, closePortal, isOpen, Portal } = usePortal() return ( <> <button ref={ref} onClick={() => openPortal()}> Open Portal </button> {isOpen && ( <Portal> <p> This Portal handles its own state.{' '} <button onClick={closePortal}>Close me!</button>, hit ESC or click outside of me. </p> </Portal> )} </> ) }
9. useHover
它确定是否正在处于hover的React元素。简单易用。该库很小,易于使用,但如果您有足够的创造力,它可能会很强大。
它还提供了悬停效果的延迟功能。支持TypeScript。虽然文档没有那么详细,但是它将向您展示如何正确地使用它。
用法示例:
import useHover from "react-use-hover"; const Example = () => { const [isHovering, hoverProps] = useHover(); return ( <> <span {...hoverProps} aria-describedby="overlay">Hover me</span> {isHovering ? <div> I’m a little tooltip! </div> : null} </> ); }
10. React router hooks
React router 是React最受欢迎的库之一。它用于路由和获取应用程序URL历史记录等。它与Redux一起实现了用于获取此类有用的数据
提供的挂钩有:
- useHistory
- useLocation
- useParams
- useRouteMatch
它的名字很不言自明。UseHistory将获取应用程序历史记录和方法的数据,例如push一个new route。UseLocation将返回代表当前URL的对象。UseParams将返回当前URL的参数的键-值对的对象。最后,useRouteMatch将尝试将当前URL与给定URL进行匹配,给定URL可以是字符串,也可以是具有不同选项的对象。
文档很好,写了很多例子
用法示例:
import { useHistory, useLocation, useRouteMatch } from "react-router-dom"; const Example = () => { let history = useHistory(); let location = useLoction(); let isMatchingURL = useRouteMatch("/post/11"); function handleClick() { history.push("/home"); } return ( <div> <span>Current URL: {location.pathname}</span> {isMatchingURL ? <span>Matching provided URL! Yay! </span> : null} <button type="button" onClick={handleClick}> Go home </button> </div> ); }
还有更多的钩子库,但是这些是我决定要谈论的。请尝试一下,我保证您不会后悔。如果您确实很喜欢它们,请以任何方式去支持他们。hooks仍然是执行此操作的一种相对较新的方法。在接下来的几个月中,我们希望有更多出色的库和钩子示例浮出水面。
希望您发现这篇文章有趣,并且您学到了一些新东西。在进一步探索hooks中玩得开心!发展愉快。
公众号:《前端阳光》 后台回复加群,欢迎和大佬们交流技术
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK