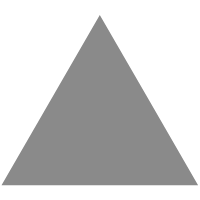
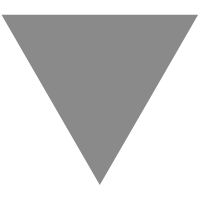
Spring Cloud Alibaba 之 Sentinel 限流规则和控制台实例
source link: https://segmentfault.com/a/1190000021671616
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
这一节我们通过一个简单的实例,学习Sentinel的基本应用。
一、Sentinel 限流核心概念
在学习Sentinel的具体应用之前,我们先来了解一下Sentinel中两个核心的概念,资源和规则。
资源
资源 是 Sentinel 中的核心概念之一。既然是限流,或者系统保护,那么是针对什么做限流?保护的是什么?就是我们所说的资源。
其实 Sentinel 对资源的定义,和并发编程中 Synchronized的使用很类似,这里的资源,可以是服务里的方法,也可以是一段代码。
规则
定义了资源之后,就是如何对资源进行保护,对资源进行保护的手段,就是我们说的规则。
使用 Sentinel 来进行资源保护,主要分为几个步骤:
- 定义资源
- 定义规则
- 检验规则是否生效
Sentinel 的所有规则都可以在内存态中动态地查询及修改,修改之后立即生效。
二、Spring Boot 限流实例
下面我实现一个使用 Sentinel 的 Hello World 实例。
1、引入 Sentinel 依赖
首先要在工程中加入 Sentinel依赖,
使用Maven管理依赖的项目,只要在 pom.xml 文件中加入以下代码即可:
<dependency> <groupId>com.alibaba.csp</groupId> <artifactId>sentinel-core</artifactId> <version>1.7.1</version> </dependency>
非Maven项目,可以下载Jar包文件到本地,中央仓库地址 Maven Center Repository 。
这里使用注解的方式,还需要加入 Sentinel 的切面支持依赖,Pom文件如下
<dependency> <groupId>com.alibaba.csp</groupId> <artifactId>sentinel-annotation-aspectj</artifactId> <version>1.7.1</version> </dependency>
2、配置资源和规则
首先编写启动类和代码入口,
@SpringBootApplication public class Application { public static void main(String[] args) { SpringApplication.run(ServiceApplication.class, args); } } @RestController public class TestController { @Autowired private TestService service; @GetMapping(value = "/hello/{name}") public String apiHello(@PathVariable String name) { return service.sayHello(name); } }
在服务层定义资源,这里使用注解 @SentinelResource,@SentinelResource 注解用来标识资源是否被限流、降级。
@Service public class TestService { @PostConstruct private void initFlowRules(){ List<FlowRule> rules = new ArrayList<>(); FlowRule rule = new FlowRule(); rule.setResource("HelloWorld"); rule.setGrade(RuleConstant.FLOW_GRADE_QPS); // 设置QPS为1 rule.setCount(1); rules.add(rule); FlowRuleManager.loadRules(rules); } @SentinelResource(value = "HelloWorld",blockHandler="degradeMethod") public String sayHello(String name) { return "Hello, " + name; } /** * 降级方法,限流后应用 * @return */ public String degradeMethod(String name, BlockException blockException){ return "请求被限流,触发限流规则="+blockException.getRule().getResource(); } }
定义了被隔离的资源以后,就是规定具体的限流和降级的规则,这里在initFlowRules()方法中初始化规则,添加了一个服务限流后触发的degradeMethod()方法。
3、限流演示
我在限流规则里定义了最大QPS不能超过1,反复刷新几次页面请求,就会被限流。
4、连接控制台
连接控制台需要添加下面的依赖:
<dependency> <groupId>com.alibaba.csp</groupId> <artifactId>sentinel-transport-simple-http</artifactId> <version>1.7.1</version> </dependency>
启动时加入 JVM 参数 -Dcsp.sentinel.dashboard.server=consoleIp:port。
-Dcsp.sentinel.dashboard.server=192.168.43.120:8719
这里我把启动参数添加在Idea Configuration下的VM options中,
启动应用后刷新控制台,可以看到应用已经注册上去。
三、主流框架的快速适配
上面演示的是一个极简单的限流例子,资源需要开发者自己去定义,那么在一些约定的场景,比如在微服务调用下,Sentinel能否自动识别服务调用,不改动代码就可以进行限流降级呢?
答案是肯定的,针对主流的框架,Sentinel 提供了许多开箱即用的特性支持,包括阿里自家的 Dubbo,Spring Cloud,以及Spring WebFlux,gRPC等框架。
适配 Dubbo
Sentinel 提供 Dubbo 的相关适配 Sentinel Dubbo Adapter,主要包括针对 Service Provider 和 Service Consumer 实现的 Filter。
sentinel-apache-dubbo-adapter(兼容 Apache Dubbo 2.7.x 及以上版本,自 Sentinel 1.5.1 开始支持)
sentinel-dubbo-adapter(兼容 Dubbo 2.6.x 版本)
对于 Apache Dubbo 2.7.x 及以上版本,使用时需引入以下模块(以 Maven 为例):
<dependency> <groupId>com.alibaba.csp</groupId> <artifactId>sentinel-apache-dubbo-adapter</artifactId> <version>${version}</version> </dependency>
对于 Dubbo 2.6.x 及以下版本,使用时需引入以下模块(以 Maven 为例):
<dependency> <groupId>com.alibaba.csp</groupId> <artifactId>sentinel-dubbo-adapter</artifactId> <version>${version}</version> </dependency>
引入此依赖后,Dubbo 的服务接口和方法(包括调用端和服务端)就会成为 Sentinel 中的资源,在配置了规则后就可以自动享受到 Sentinel 的防护能力。
适配 Spring Cloud
Sentinel 对 Spring Cloud 的支持,也就是 Spring Cloud Alibaba全家桶,默认为 Sentinel 整合了 Servlet、RestTemplate、FeignClient 和 Spring WebFlux。Sentinel 在 Spring Cloud 生态中,不仅补全了 Hystrix 在 Servlet 和 RestTemplate 这一块的空白,而且还完全兼容了 Hystrix 在 FeignClient 中限流降级的用法,并且支持运行时灵活地配置和调整限流降级规则。
在Spring Cloud 项目中引入 Sentinel,添加如下依赖就可以,
<dependency> <groupId>com.alibaba.cloud</groupId> <artifactId>spring-cloud-starter-alibaba-sentinel</artifactId> </dependency>
总结
这节给大家演示了一个 Sentinel的应用实例,并且介绍了Sentinel 和主流框架的集成,下一节的内容会深入解析 @SentinelResource注解。
关注公众号:架构进化论,获得第一手的技术资讯和原创文章
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK