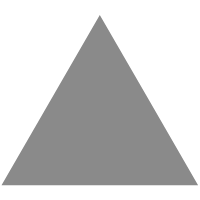
17
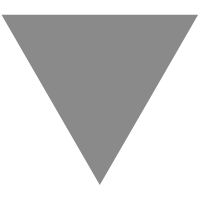
GitHub - SwiftDocOrg/CommonMark: Create, parse, and render (spec-compliant) Comm...
source link: https://github.com/SwiftDocOrg/CommonMark
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
README.md
CommonMark
A Swift package for working with CommonMark text. It's built on top of libcmark and fully compliant with the CommonMark Spec.
Usage
import CommonMark let markdown = #""" # [Universal Declaration of Human Rights][uhdr] ## Article 1. All human beings are born free and equal in dignity and rights. They are endowed with reason and conscience and should act towards one another in a spirit of brotherhood. [uhdr]: https://www.un.org/en/universal-declaration-human-rights/ "View full version" """# let document = try Document(markdown)
Inspecting Document Nodes
document.children.count // 3 let heading = document.children[0] as! Heading heading.headerLevel // 1 heading.children.count // 1 let link = heading.children[0] as! Link link.urlString // "https://www.un.org/en/universal-declaration-human-rights/") link.title // "View full version" let subheading = document.children[1] as! Heading subheading.headerLevel // 2 subheading.children.count // 1 let subheadingText = subheading.children[0] as! Text subheadingText.literal // "Article 1." let paragraph = document.children[2] as! Paragraph paragraph.description // "All human beings [ ... ]" paragraph.range.lowerBound // (line: 5, column: 1) paragraph.range.upperBound // (line: 7, column: 62)
Rendering to HTML, XML, LaTeX, and Manpage
let html = document.render(format: .html) // <h1> [ ... ] let xml = document.render(format: .xml) // <?xml [ ... ] let latex = document.render(format: .latex) // \section{ [ ... ] let manpage = document.render(format: .manpage) // .SH [ ... ] // To get back CommonMark text, // you can either render with the `.commonmark` format... document.render(format: .commonmark) // # [Universal [ ... ] // ...or call `description` // (individual nodes also return their CommonMark representation as their description) document.description // # [Universal [ ... ]
Creating Documents From Scratch
let link = Link(urlString: "https://www.un.org/en/universal-declaration-human-rights/", title: "View full version", text: "Universal Declaration of Human Rights") let heading = Heading(level: 1, children: [link]) let subheading = Heading(level: 2, text: "Article 1.") let paragraph = Paragraph(children: #""" All human beings are born free and equal in dignity and rights. They are endowed with reason and conscience and should act towards one another in a spirit of brotherhood. """#.split(separator: "\n") .flatMap { [Text(String($0)), SoftLineBreak()] }) Document(children: [heading, subheading, paragraph]).description == document.description // true
CommonMark Spec Compliance
This package passes all of the 649 test cases in the latest version (0.29) of the CommonMark Spec:
$ swift test Executed 649 tests, with 0 failures (0 unexpected) in 0.178 (0.201) seconds
Requirements
- Swift 5.1+
Installation
Swift Package Manager
Add the CommonMark package to your target dependencies in Package.swift
:
import PackageDescription let package = Package( name: "YourProject", dependencies: [ .package( url: "https://github.com/SwiftDocOrg/CommonMark", from: "0.1.2" ), ] )
Then run the swift build
command to build your project.
License
MIT
Contact
Mattt (@mattt)
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK