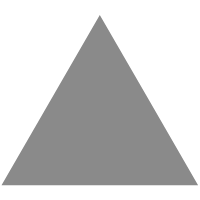
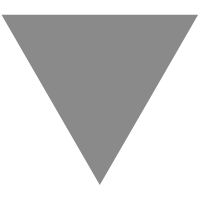
Static Type Checking vs Dynamic Type Checking in JavaScript
source link: https://blog.bitsrc.io/static-type-checking-vs-dynamic-type-checking-in-javascript-13643f4952a9
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
Do you prefer discovering bugs during execution time or during the bundling (or any other stage you have before publishing your code)? Would you rather let the code figure out your data types or do you prefer leaving clues about them for other tools to perform the checks for you?
This is not about right vs wrong or having a sure way to always perform type checking correctly. In fact, it’s all about circumstances and personal preferences.
Let me explain: Type Checking takes care of making sure you’re using the data as expected. In other words, it’ll help you check you’re not using a number like a string, or that you’re not trying to subtract 42 from the word “cat”. These examples might sound crazy, but for a loosely typed language, such as JavaScript, they are, not only possible but likely to happen.
Whatever the case is, in this article I’m will be covering what both methods of performing type checking are and how you can achieve them in JavaScript.
Tip: When sharing reusable JS components using tools like Bit ( Github ) — use type-checking. It will make your shared components more developer-friendly and less prone to errors.

Static Type Checking in JavaScript
This type of type-checking is performed before execution, it can happen during compilation (if you’re working with a compiled language) or during the bundling stage (if you’re working with a scripting language). Considering that this is another form of code-verification, performing it whenever you do your unit tests is a good idea.
That being said, the concept of static type checking for JavaScript is “unnatural”, since by definition, the language is weakly typed (or dynamically typed, depending on which definition you want to go with). So performing type checking on JavaScript means dealing with the following facts:
- The language has types attached to values
-
Operations with those values react in different ways depending on their types (i.e you can
divide 2 by “hello”, and you’ll get
NaN
, while 2 divided by 2 would yield a number). - Variables don’t have a type, so you can use the same variable to store a string value, then assign it a number and it’ll still be valid code and it will execute correctly.
With this in mind, the following code would be perfectly valid in JavaScript:
Reading the above code, what would you say is the type of x
or the return type of fn
? Really, you can’t tell, and the language will even try its best to accommodate for some strange, unforeseen use cases, such as:
fn("hello") => "hello1" fn(_ => 3) => "_ => 31" fn({prop:1}) => "[object Object]1"
With that in mind I think I’ve answered the following question:
Why would you go through the trouble of adding static type checking into a language that was designed to work without it?
Clearly, trying to avoid scenarios like the ones described above is a very common answer.
Others might include:
- Code becomes less error-prone since you have an extra layer of verification on top of your code that catches simple (yet very common) mistakes.
- Potential for code optimization, if your compiler (and I’m using that word lightly here, but you can think of transpilers as well) can pick up on better ways to write some sentences based on the types you’re using.
- Your code is easier to read by others. Not having to mentally parse types is a great help for developers trying to understand code from others.
- Support for better tooling. With static type declarations, your IDEs can help you write better code by making suggestions or performing checks while you write.
I’m sure you can also add some more things to that list, and that alone shows how much potential a static type checker for JavaScript has, so what are our options?
Existing Static Type Checkers for JavaScript
Although the idea (and the added benefits) of having a static type checker for this language might sound so compelling, the actual effort required to do so seems to have hindered most efforts.
Therefore, there are two really useful options out there: Flow and TypeScript .
Using Flow
Flow allows you to add type annotations to your Vanilla JavaScript and then process that code to check for problems.
You can install it with npm
writing:
$
npm install --save-dev flow-bin
And then installing its type-remover (since you’ll need to strip away the added annotations for your code to be correctly interpreted):
$ npm install --save-dev flow-remove-types
You’ll also need to add a few script to the package.json
file of your project:
That is, of course, assuming your code is inside the src
folder and that you want the final version (the “compiled” JS code,if you will) to be stored inside the lib
folder.
You can then start annotating your code like this (notice the first line, without it, Flow will ignore your files, so don’t forget about it!):
Later, if you were to test your code with Flow, you’d get something like this:
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK