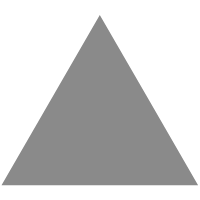
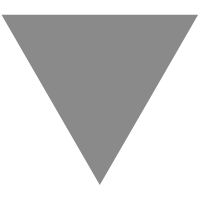
Read Replicas and Spring Data, Part 2: Configuring the Base Project
source link: https://www.tuicool.com/articles/aMru2eQ
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
In our previouspost, we set up multiple PostgreSQL instances with the same data. Our next step would be to configure our Spring project by using both servers.
As stated previously, we shall use some of the code taken from theSpring Boot JPA post, since we use exactly the same database.
You may also like: The Magic of Spring Data
This will be our Gradle build file:
plugins { id 'org.springframework.boot' version '2.1.9.RELEASE' id 'io.spring.dependency-management' version '1.0.8.RELEASE' id 'java' } group = 'com.gkatzioura' version = '0.0.1-SNAPSHOT' sourceCompatibility = '1.8' repositories { mavenCentral() } dependencies { implementation 'org.springframework.boot:spring-boot-starter-data-jpa' implementation 'org.springframework.boot:spring-boot-starter-web' implementation "org.postgresql:postgresql:42.2.8" testImplementation 'org.springframework.boot:spring-boot-starter-test' }
Now, let's proceed with creating the model based on the table created inthe previous blog.
package com.gkatzioura.springdatareadreplica.entity; import javax.persistence.Column; import javax.persistence.Entity; import javax.persistence.GeneratedValue; import javax.persistence.GenerationType; import javax.persistence.Id; import javax.persistence.Table; @Entity @Table(name = "employee", catalog="spring_data_jpa_example") public class Employee { @Id @Column(name = "id") @GeneratedValue(strategy = GenerationType.IDENTITY) private Long id; @Column(name = "firstname") private String firstName; @Column(name = "lastname") private String lastname; @Column(name = "email") private String email; @Column(name = "age") private Integer age; @Column(name = "salary") private Integer salary; public Long getId() { return id; } public void setId(Long id) { this.id = id; } public String getFirstName() { return firstName; } public void setFirstName(String firstName) { this.firstName = firstName; } public String getLastname() { return lastname; } public void setLastname(String lastname) { this.lastname = lastname; } public String getEmail() { return email; } public void setEmail(String email) { this.email = email; } public Integer getAge() { return age; } public void setAge(Integer age) { this.age = age; } public Integer getSalary() { return salary; } public void setSalary(Integer salary) { this.salary = salary; } }
The next step is to create a Spring Data repository.
package com.gkatzioura.springdatareadreplica.repository; import org.springframework.data.jpa.repository.JpaRepository; import com.gkatzioura.springdatareadreplica.entity.Employee; public interface EmployeeRepository extends JpaRepository<Employee,Long> { }
We are also going to add a controller.
package com.gkatzioura.springdatareadreplica.controller; import java.util.List; import org.springframework.web.bind.annotation.RequestMapping; import org.springframework.web.bind.annotation.RestController; import com.gkatzioura.springdatareadreplica.entity.Employee; import com.gkatzioura.springdatareadreplica.repository.EmployeeRepository; @RestController public class EmployeeContoller { private final EmployeeRepository employeeRepository; public EmployeeContoller(EmployeeRepository employeeRepository) { this.employeeRepository = employeeRepository; } @RequestMapping("/employee") public List<Employee> getEmployees() { return employeeRepository.findAll(); } }
All that it takes is to just add the right properties in your application.yaml:
spring: datasource: platform: postgres driverClassName: org.postgresql.Driver username: db-user password: your-password url: jdbc:postgresql://127.0.0.2:5432/postgres
Spring Boot has made it possible nowadays not to bother with any JPA configurations.
This is all you need in order to run the application. Once your application is running, just try to fetch the employees.
curl http://localhost:8080/employee
As you have seen, we did not do any JPA configurations. Since Spring Boot 2, specifying the database url is sufficient for the auto-configuration to kick in and do all this configuration for you.
However, in our case, we want to have multiple data source and entity manager configurations. In the next post, we shall configure the entity managers for our application. Stay tuned!
Further Reading
Switch Your PostgreSQL Primary for a Read Replica Without Downtime
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK