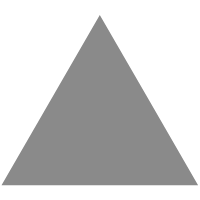
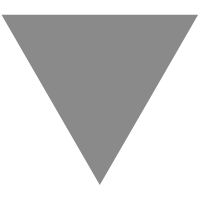
Functional Programming in JavaScript: How and Why
source link: https://www.tuicool.com/articles/FZfQR3R
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
How to write ultra-modular, easily testable, easily maintainable, and highly reusable Javascript w/o React
Aug 21 ·9min read
With the growing trend in usage of Functional Programming for JavaScript these days, it’s only natural to ask things like “What’s with all the hype?” “What are the benefits?” “What are the real-world applications of Functional Programming?” “Does it fit in our use-case?”
If you are one of those people, we will answer them here.
In this article, we will answer what Functional Programming is, its features and benefits. We will also use Bit as our tool to export and share our reusable components/modules and provide some real-world use-cases for that programming paradigm. Feel free to check it out on GitHub.
Before the popularity of Functional Programming
Before its popularity, we used to use Object-Oriented Programming in most of our real-world use-cases for better or for worse. Most use-cases of Functional Programming back then rely solely on mathematical computations whether simple or complex and the usage of it in components like buttons, textbox, checkbox never really fit in most of our scenarios.
In the early years of my career as a Software Engineer around 2013, I don’t remember using Functional Programming at all. If anything, I was only making use of Object-Oriented Programming because I was developing desktop applications using .net at the time and it’s heavily using OOP paradigm.
Although somewhere around 2015 I tried to delve in the world of Functional Programming using F#, I only have a few use-cases for FP in mind at the time so my interest in it didn’t take off by a mile and started to lose interest altogether.
I also have started my Web Development experience during these years and was using jQuery in most of my work. So far, no patterns were introduced here, not unless you use AngularJS which is google’s first version of their framework. I have used it a couple of times as well, but its pattern is based on MVC which stands for Model-View-Controller.
Then came 2017 when these big libraries and frameworks like Angular, React, Vue started to gain traction among web developers which were built with component-based patterns in mind.
Things started to change for JavaScript since then and Functional Programming in Front-End web development were starting to have its appeal thanks to those frameworks. Apparently, it was React which encourages functional-based design when using their library since it enforces you not to mutate your objects and not introduce side-effects as well. It was also thanks to React that I got to appreciate Functional Programming even more.
What is Functional Programming?
So then, what is Functional Programming?
Functional Programming is a programming paradigm where you mostly construct and structure your code using functions. These functions take input which is called as arguments then shows the output based on the inputs being taken which, given the same input always results in the same output.
It doesn’t allow any mutation nor shared state, as functions should remain pure and true to their expression under this paradigm. It is declarative rather than imperative/procedural.
You could also say that Functional Programming was simply a bunch of functions that don’t allow outside scope nor mutation of objects. Rather than we mutate arrays by adding/removing inside the scope to achieve a solution like OOP does, functional is pure, straightforward and doesn’t mutate. It presents an answer to its elegant function in a straightforward manner.
It’s also worth noting that JavaScript is a multi-paradigm programming language and Functional Programming is one of them. Just because it’s capable of declaring functions doesn’t mean you’re using the paradigm. It needs to observe proper implementation to fully use it.
Features of Functional Programming
We can’t discuss the benefits of Functional Programming without discussing its features. Here are some of the most notable features of Functional Programming:
First-Class Citizen Functions
What’s good about Functional Programming is its functions are first-class citizens: you can always insert functions inside a function without any restrictions present.
function executeFunctions(x, y) { const add = (x, y) => x + y; const subtract = (x, y) => x — y; console.log(`sum: ${add(x,y)}`); console.log(`difference: ${subtract(x,y)}`);}
Higher-Order Functions
A higher-order function is a function that gets a function as an argument. It may or may not return a function as its resulting output.
Here’s an example of Higher-Order Function:
function greaterThan(n) { return x => x > n; }let greaterThanTwo = greaterThan(2);console.log(greaterThanTwo(5));
Function Composition
Functional Programming won’t be completely functional without this feature. Function Composition is an act of composing/creating functions that allow you to further simplify and compress your functions by taking functions as an argument and return an output. It may also return another function as its output other than numerical/string values.
Here is an example of Function Composition:
var compose = (f, g) => (x) => f(g(x));
There is also a variation in the way we compose functions by the name of Monads. Monads are just another variation of Function Composition wherein it requires a context in addition to its output. Monads can type lift, flatten, and map so that it can make functions composable.
We won’t be discussing monads in this article.
Benefits of Functional Programming
One of the reasons Functional Programming became popular were its benefits. Let’s take a look at the benefits of using Functional Programming:
It doesn’t have side-effects and it’s immutable
One of the killer benefits of using Functional Programming is its ability to not show any side-effects in the code. It reduces the likelihood that the code will introduce some bugs since it’s not going to mutate and the bug is easy to spot since it’s within the scope of the function. Once a variable has a value assigned to it, it is no longer subject to change.
It’s not that side-effects are necessarily bad. In fact, it’s impossible to avoid side-effects altogether in building apps as we’ve been dealing with procedural programming in the past with no regard to whether data structures have side-effects or not.
If we ever introduce immutability in some use-cases, you can be sure that it’s definitely not going to cause you any headaches than any other programming paradigms that introduce mutable objects.
Since Functional Programming is a Pure Function, this means that it won’t be borrowing any data outside of its scope, let alone allow any mutable objects. It only cares about what’s happening within their scope, provides an output based on its input and nothing else.
It’s clean, straightforward, succinct
Functional Programming has always been straightforward so it’s easy to spot some inconsistencies and debug some bugs in the function. In fact, constructing functions are cleaner and easier to maintain than constructing a class as in OOP since you need to think in terms of imperative/procedural pattern such as designing class hierarchies, encapsulation, inheritance, methods, polymorphism.
However, straightforward doesn’t mean it’s easier to adapt. If you came from OOP background, you need to make a shift in your thinking and especially make sure that your objects don’t mutate. You can’t call it a Functional Programming if there’s a shared state, objects mutate, or there’s some leakage outside of its function scope in any way. It should be limited to its function scope.
When you declare a function, it’s true to its intentions which is to give you the function scope that you need. It clearly follows the KISS principle of Computer Science which in other words is to simplify things up in order to produce an output.
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK