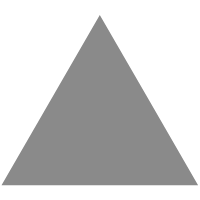
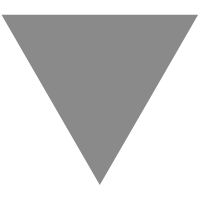
How To Build a Bot That Sends Dog Images - Better Programming - Medium
source link: https://medium.com/better-programming/learn-how-to-create-your-own-texting-bot-that-sends-dog-pictures-532e32c611b3
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
How To Build a Bot That Sends Dog Images
A step by step guide
Before we dive into this post, I would like to send a message to anyone entering the technology/programming world:
When I first started to learn how to program, it felt as if I was in a rut of learning the fundamentals and never really knowing what I can apply with the toolkit I am slowly coming to understand. In my opinion, not knowing how you can apply your programming knowledge to daily activities is a difficult obstacle to overcome. It has led several friends of mine to drift away from programming.
Whenever I learn a new technology, after getting my feet wet with the syntax, I always pose a question to myself, “What can I build with this?” Regardless of your skill level, I highly suggest you do the same. As developers, we grow by tackling difficult problems. And we end up building some pretty cool things!
One of the projects that I took up when learning a new language was creating an API that generates dog pictures! I want to walk you through how to create a text-messaging bot that can send you dog pictures on command using some pretty cool technologies. Let’s get started!

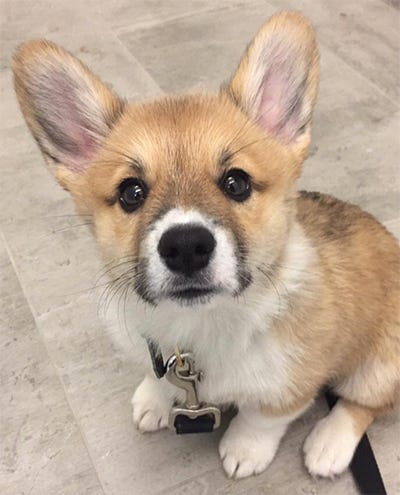
First Things First — Prerequisites and the Workflow:
We will be utilizing Twilio for this tutorial. You’’ll need a phone number registered on your Twilio account. If you do not have a phone number registered, read this tutorial on how to get your own phone number utilizing the Twilio trial and come back when you have a phone number registered.
Twilio offers a pretty neat, serverless function feature for us. A serverless function allows the developer to deploy their code to the cloud. The third party service, in our case Twilio, will host that code for us. This is awesome because it eliminates the hassle of an external server to host the code!
To generate dog pictures for us, we will be using woofbot.io — a free API service that generates dog pictures for you. You can view the documentation for this API here, but it isn’t necessary for the scope of this tutorial.
When dealing with any API that is returning JSON, a chrome extension I swear by is JSON Formatter. You can thank me later!
In this program, our flow will look something like the following:
- Our Twilio phone number receives an incoming text message requesting a dog image of a particular breed.
- We contact the woofbot API saying, “Hey woofbot, I need a picture of this breed!”
- We validate the API response and parse out the image URL that was given to us.
- We then send that image URL over to the phone number that texted the Twilio phone number.
This flow may seem a bit overwhelming at first glance, but we’ll focus on one small part at a time. Before you know it, we’ll have completed the whole flow.

Setting up a Twilio Serverless Function
Head over to your Twilio Dashboard. On the left sidebar, click the All Products & Services Icon:

In the expanded sidebar, scroll down to the Runtime section and click the Functions item. You should be on this page:

Click the plus icon. Twilio is kind enough to offer us a set of boilerplate templates. Go ahead and choose the Hello SMS template and click the Create button:

We have now created a runtime function with an SMS template. If you want, you can go ahead and modify the Function Name field to be something that is more reflective of this project. I’ll update mine to Dog Bot.
In the flow we described above, it sounds like the whole flow is initiated in the event of an incoming text message. Under Configuration, click the Event dropdown and select Incoming Messages and click the Save button. For the path, you can make it whatever you want — I will use woofbot.

Right now, our function flow is expressing: “On the event of an incoming message, run this code that replies to the person saying Hello World.”
After saving, we can text the Twilio phone number to validate this:
Great! Let’s move on to sending a picture of a dog in our message. On line three we can replacetwiml.message("Hello World")
withtwiml.message().media(“https://direct_link_to_image.com/image.png”);
. This will attach a media object with the link provided to our twiml.message()
object.
We can test this by generating a call to the woofbot API. First, we need to figure out what breeds the API is supporting by visiting this URL:

Based on this list, we can generate an API call to grab a picture of any these breeds through the following format: https://api.woofbot.io/v1/breeds/{breed}/image
where {breed}
is the breed you’re interested in.
I want a corgi picture, so I’ll visit https://api.woofbot.io/v1/breeds/corgi/image
. After visiting, we will have a url
field that contains the direct link to a corgi image:

Copy the URL of the picture and we can paste it into our serverless function code:
After saving this change, we should now be able to text our Twilio phone number and receive a dog picture.
Hooray! We have made a program that sends out a dog picture on request! Our current solution is okay, but how can we make this program more dynamic, as our initial flow stated? Let’s take a look.
Creating a Dynamic Serverless Function
To describe what we just did manually when finding a dog picture:
- We visited the woofbot breed list and selected a breed.
- We then visited the generate image API URL for that specific breed and copied the direct image link the
url
field gave us.
How can we transfer this flow into our program? By using an HTTP client library. To put that into ordinary language, an HTTP client library translates to a web browser that is used in an application. For this tutorial, we’ll be utilizing the Axios library.
Let’s install this library so our function can utilize it.
Under Functions, head over to the Configure area on the left sidebar:

Under Dependencies, click the Plus icon and for the Name, enter axios
and for the version, enter 0.19.0
. Click the Save button and head on over back to the Manage section of our Runtime Function.
To import Axios into the function, add the following line to the top of our program:
const axios = require(‘axios’);

If you want to read up on the methods Axios provides, look at the documentation, which includes excellent examples. From here, let’s create a GET request to the endpoint of our desired breed right below line four.

This call returns a promise. If you are unfamiliar with promises, that’s fine — here’s the basic idea of dealing with code that returns a promise:

The content of our HTTP response is always stored within response.data
. The direct image URL will specifically be in response.data.response.url
— which should make sense if we look at the JSON:

With this knowledge, we can shift around a few lines in our program to dynamically grab a new URL after we contact the API each time. We should also account for the error by invoking our callback function with the error we received:
After saving, here comes the moment of truth! We should be able to text our Twilio phone number twice and receive two different dog images!
We’ve accomplished some serious work! However, there is one significant improvement we can add: support for multiple breeds!
In our serverless function, we passed in an event
variable that contains all relevant information pertaining to the current event, such as the sender’s phone number and the body of the text message. To retrieve the string of the text message we access it through event.Body
.
Let’s create a variable that makes our API call dependent on the user input:
You have now set up fetching dynamic dog pictures by accepting user input.
Good job!
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK