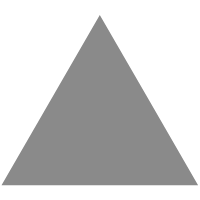
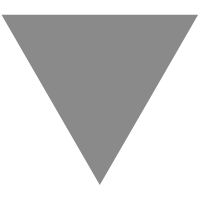
Introduction to PixiJS: An HTML5 2D rendering engine
source link: https://www.tuicool.com/articles/uIfY7bY
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
JavaScript is becoming more and more powerful. There are many games built with JavaScript usually using 2D or 3D libraries. PixiJS is one of those 2D libraries which works across all the devices. It is an HTML5 2D rendering engine that supports WebGL API and falls back to canvas when needed.
Prerequisites
Include PixiJS library by downloading the prebuilt build ! It can also be installed via npm or embed CDN URL directly
Installing via NPM:
npm install pixi.js
Then in your script file:
import * as PIXI from 'pixi.js'
If you want to embed CDN URL:
<script src=" https://cdnjs.cloudflare.com/ajax/libs/pixi.js/5.0.4/pixi.min.js"></script>
Creating Sprites
In this article, we are going to create Tiling sprite. Create a new PIXI application as follows:
const app = new PIXI.Application();
This creates the renderer, ticker and root container for you.
In the next step, create a canvas element and attach it to HTML document.
document.body.appendChild(app.view);
Here, app.view creates the canvas element. Let us create a texture that we are going to use in tiling sprite.
const texture = PIXI.Texture.from('https://i1.wp.com/techshard.com/wp-content/uploads/2017/04/animation.jpg?ssl=1&resize=438%2C438');
A tiling sprite is a fast way of rendering a tiling image. Initialize a tiling sprite object as follows:
const tilingSprite = new PIXI.extras.TilingSprite( texture, app.renderer.width, app.renderer.height );
We are now going to attach the tiling sprite object to root display container that’s rendered.
app.stage.addChild(tilingSprite);
We will make use of the Ticker class that runs an update loop for the objects that are listening.
let count = 0; app.ticker.add(() => { count += 0.0005; tilingSprite.tileScale.x = 2 + Math.sin(count); tilingSprite.tileScale.y = 2 + Math.cos(count); tilingSprite.tilePosition.x += 1; tilingSprite.tilePosition.y += 1; });
Here, tilingSprite.tileScale is to scale the image that is being tiled. tilingSprite.tilePosition is to the offset the image that is being tiled.
That’s it! Run the application.
Check out the complete demo here .
Wrapping Up
Working with PixiJS is easy and fun. It lets you create rich, interactive graphics, cross platform games and applications. It offers a lot of cool features. If you have not checked PixiJS yet, check out their documentation and examples .
Advertisements
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK