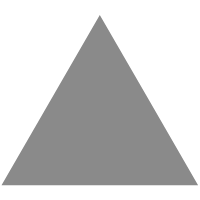
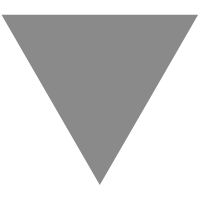
Casting a C# Object From Its Parent
source link: https://www.tuicool.com/articles/jqaeamU
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
Have you ever tried to do something akin to the following?
[Fact] public void ConvertClassToSubClass_Converts() { // Arrange var parentClass = new SimpleTestClass(); parentClass.Property1 = "test"; // Act var childClass = parentClass as SimpleTestSubClass; // Assert Assert.Equal("test", childClass.Property1); }
This is a simple Xunit (failing) test. The reason it fails is because you (or I) am trying to cast a general type to a specific, and C# is complaining that this may not be possible; consequently, you will get null (or for a hard cast, you'll get an InvalidCastException).
Okay, that makes sense. After all, parentClass
could actually be a SimpleTestSubClass2
and, as a result, C# is being safe because there's (presumably, I don't work for MS) too many possibilities for edge cases.
This is, however, a solvable problem; there are a few ways to do it, but you can simply use reflection:
public TNewClass CastAsClass<TNewClass>() where TNewClass : class { var newObject = Activator.CreateInstance<TNewClass>(); var newProps = typeof(TNewClass).GetProperties(); foreach (var prop in newProps) { if (!prop.CanWrite) continue; var existingPropertyInfo = typeof(TExistingClass).GetProperty(prop.Name); if (existingPropertyInfo == null || !existingPropertyInfo.CanRead) continue; var value = existingPropertyInfo.GetValue(_existingClass); prop.SetValue(newObject, value, null); } return newObject; }
This code will effectively transfer any class over to any other class.
If you'd rather use an existing library, you can always use this one . It's also o pen sourced on GitHib .
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK