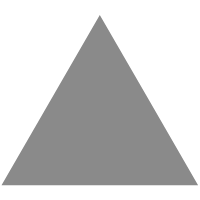
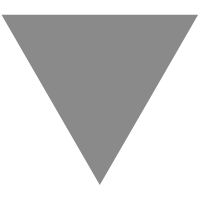
Pattern Matching Proposal
source link: https://www.tuicool.com/articles/FRRNN3
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
If you’re coming from a functional programming language such as Elixir , Haskell or ReasonML , you may know how frustrating is to switch to a language which does not support pattern matching natively.
Once you start to approach problem using pattern matching, you’ll never go back to the messy if/else if/ else
statements!
Let’s see what I’m talking about, coding the famous FizzBuzz interview question in both ReasonML and plain JavaScript :


So as you can see in the ReasonML implementation above, we’re using a switch
statement which pattern matches agains a tuple , returning the desired output on specific conditions.
I’ll explain for you how does it work, just in case you’re not familiar with ReasonML:
- On line 1, we define a new function which takes an integer
i
as a parameter and returns astring
. Pretty similar to TypeScript , isn’t it? - On line 2 we declare a
switch
statement, which works similarly to the JavaScriptswitch
. In that case, we’re switching against a tuple, which is a ReasonML immutable, ordered and fix-sized data structure that contains two integers: the result ofi % 3
and the result ofi % 5
. (mod
stays for the modulo operator). - On line 3, we check if the tuple matches the declared condition, just like a JavaScript
case
statement. If the tuple is(0, 0)
(so the number is evenly divisible by 15), we return"FizzBuzz"
. Otherwise we continue with the next statement. - On line 4, we check if the given tuple is equal to
(0, _)
, where the underscore means “any value”. So, if the given parameteri
is evenly divisible just by 3, we return"Fizz"
, and so on with the other statements.
So, what about JavaScript? How can we implement a similar solution, given that we don’t have any native pattern matching implementation?


Believe it or not, this is the closest ReasonML to JavaScript implementation I came up with!
Let’s break it down:
- On line 3, we use the ES6 Destructuring Assignement in order to get an array containing just two values (
x
andy
) wherex
is the result ofi%3
andy
is the result ofi%5
. - At time of writing, the only real alternative to pattern matching in JavaScript is the
switch/case
control structure, testing agains thetrue
boolean value. So we use thatswitch/case
to check if our valuei
should returnFizzBuzz
,Fizz
and so on.
This is not the most elegant solution for the FizzBuzz challenge, but I hope that it allowed you to understand what pattern matching is and why we need it!
The Proposal


And here it is, the FizzBuzz solution using the Pattern Matching Proposal !
It looks incredibly similar to the ReasonML solution, doesn’t it?
In JavaScript we don’t have tuples, so we still use arrays, but look at the code: we don’t even need to destructure our array and get x
and y
values!
Just like in ReasonML , OCaml , Elixir etc, we use the underscore as a “catch-all” character.
- On line 3 we define our new
case
statement, which accepts any kind of value as argument. In that case, we pass in just an array with two integers. - On line 4, we check if the array we previously passed to the
case
statement is equal to[0, 0]
. If that is the case, we return"FizzBuzz"
. - On line 5, we check if the first element of our array is equal to
0
. We don’t mind about the second element, which can be literally anything. Same for the next cases.
Data Fetching


Let’s pretend we need to call a REST API service, and we need to check the response status code.
It’s a perfect task for our new pattern matching friend! We can pattern match against our fetch
response and return the informations we need.
React and JSX


Mixing the newest case
control structure with JSX is really an amazing way to explore its potential. It really helps loading the right component and adds an extra layer of safety thanks to the _
(catch-all) char! With it, you can both throw a new error, or load a “generic-error-component” without causing runtime exceptions.
Using Pattern Matching Today
At time of writing (May 2019), the Pattern Matching proposal is in stage-1 , so you can use it thanks to Babel !
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK