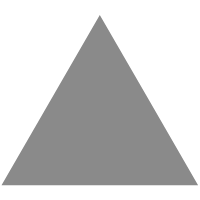
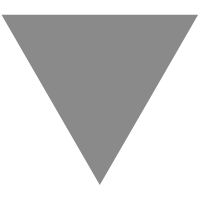
Building Data Visualization with Angular and Ngx-charts
source link: https://www.tuicool.com/articles/uqa67n2
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
Data Visualization is visual representation of quantitative information in the form of charts, graphs, plots and so on. There are so many libraries and frameworks available to visualize data, but the one that we are going to talk about in this article is ngx-charts.
Ngx-charts is a charting framework for Angular which wraps D3 JavaScript library and uses Angular to render and animate SVG elements. It is one of the most popular frameworks for Angular application because it makes so much easier to render charts and provides other possibilities the Angular platform offers such as AoT, Universal, etc.
In this article, we will look at some simple examples using Ngx-charts.
Setting up Angular Project with Ngx-charts
Create a new Angular project and install ngx-charts and d3 dependencies as follows.
npm install @swimlane/ngx-charts --save
If you need to use some specific D3 shapes, then you could install the following dependencies. But, we do not need them for this tutorial.
npm install d3 --save npm install @types/d3-shape --save
Also, install bootstrap to provide styles in this sample project.
npm install bootstrap –save
Include NgxChartsModule and BrowserAnimationsModule in app.module.ts. Ngx-charts uses BrowserAnimationsModule internally .
import { BrowserModule } from '@angular/platform-browser'; import { NgModule } from '@angular/core'; import { AppRoutingModule } from './app-routing.module'; import { AppComponent } from './app.component'; import { BrowserAnimationsModule } from '@angular/platform-browser/animations'; import { NgxChartsModule } from '@swimlane/ngx-charts'; @NgModule({ declarations: [ AppComponent ], imports: [ BrowserModule, AppRoutingModule, NgxChartsModule, BrowserAnimationsModule ], providers: [], bootstrap: [AppComponent] }) export class AppModule { }
Import bootstrap CSS in global styles.scss .
@import "~bootstrap/dist/css/bootstrap.min.css";
Creating Simple Charts
Let us include some sample data to render charts. The app.component.ts would look like this:
import { Component } from '@angular/core'; @Component({ selector: 'app-root', templateUrl: './app.component.html', styleUrls: ['./app.component.scss'] }) export class AppComponent { title = 'Angular Charts'; view: any[] = [600, 400]; // options for the chart showXAxis = true; showYAxis = true; gradient = false; showLegend = true; showXAxisLabel = true; xAxisLabel = 'Country'; showYAxisLabel = true; yAxisLabel = 'Sales'; timeline = true; colorScheme = { domain: ['#9370DB', '#87CEFA', '#FA8072', '#FF7F50', '#90EE90', '#9370DB'] }; //pie showLabels = true; // data goes here public single = [ { "name": "China", "value": 2243772 }, { "name": "USA", "value": 1126000 }, { "name": "Norway", "value": 296215 }, { "name": "Japan", "value": 257363 }, { "name": "Germany", "value": 196750 }, { "name": "France", "value": 204617 } ]; public multi = [ { "name": "China", "series": [ { "name": "2018", "value": 2243772 }, { "name": "2017", "value": 1227770 } ] }, { "name": "USA", "series": [ { "name": "2018", "value": 1126000 }, { "name": "2017", "value": 764666 } ] }, { "name": "Norway", "series": [ { "name": "2018", "value": 296215 }, { "name": "2017", "value": 209122 } ] }, { "name": "Japan", "series": [ { "name": "2018", "value": 257363 }, { "name": "2017", "value": 205350 } ] }, { "name": "Germany", "series": [ { "name": "2018", "value": 196750 }, { "name": "2017", "value": 129246 } ] }, { "name": "France", "series": [ { "name": "2018", "value": 204617 }, { "name": "2017", "value": 149797 } ] } ]; }
Here, we have also hardcoded our view height and width, scheme, yAxis, xAxis, xAxisLabel, yAxisLabel and so on. We can also provide data via JSON file or load them dynamically from backend.
Include the components from ngx-charts to render charts. In this example, we will add components for vertical bar chart, normalized vertical bar chart and stacked vertical bar chart. The complete HTML Markup would look like this:
<!--The content below is only a placeholder and can be replaced.--> <div style="text-align:center"> <h1 class="card-header bg-info"> Welcome to {{ title }}! </h1> <h3 class="text-secondary"> PEV Selling Countries </h3> <div class="row ml-5" <div class="col"> <div class="row"> <h4> Vertical Bar Chart </h4> </div> <div class="row"> <ngx-charts-bar-vertical [view]="view" [scheme]="colorScheme" [results]="single" [gradient]="gradient" [xAxis]="showXAxis" [yAxis]="showYAxis" [legend]="showLegend" [showXAxisLabel]="showXAxisLabel" [showYAxisLabel]="showYAxisLabel" [xAxisLabel]="xAxisLabel" [yAxisLabel]="yAxisLabel" (select)="onSelect($event)"> </ngx-charts-bar-vertical> </div> </div> <div class="col"> <div class="row"> <h4> Vertical Bar Chart Normalized </h4> </div> <div class="row"> <ngx-charts-bar-vertical-normalized [view]="view" [scheme]="colorScheme" [results]="multi" [gradient]="gradient" [xAxis]="showXAxis" [yAxis]="showYAxis" [legend]="showLegend" [showXAxisLabel]="showXAxisLabel" [showYAxisLabel]="showYAxisLabel" [xAxisLabel]="xAxisLabel" [yAxisLabel]="yAxisLabel" (select)="onSelect($event)"> </ngx-charts-bar-vertical-normalized> </div> </div> </div> <div class="row ml-5"> <h4> Stacked Vertical Bar Chart </h4> </div> <div class="form-group row ml-5"> <ngx-charts-bar-vertical-stacked [view]="view" [scheme]="colorScheme" [results]="multi" [gradient]="gradient" [xAxis]="showXAxis" [yAxis]="showYAxis" [legend]="showLegend" [showXAxisLabel]="showXAxisLabel" [showYAxisLabel]="showYAxisLabel" [xAxisLabel]="xAxisLabel" [yAxisLabel]="yAxisLabel" (select)="onSelect($event)"> </ngx-charts-bar-vertical-stacked> </div> </div>
Now, we are ready with our simple app which renders charts. Start the server using ng-serve command. The final app would look like this.
Ngx-charts Demo Conclusion
Ngx-charts is a powerful framework and simple to use in Angular applications. Checkout the documentation and examples for Ngx-charts.
The example described in this article can be found in this GitHub repository.
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK