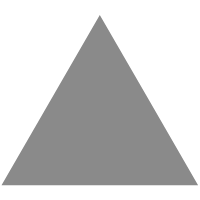
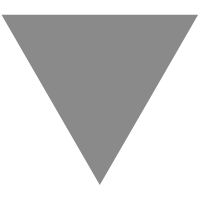
Python 2.7终结于7个月后,这是你需要了解的3.X炫酷新特性
source link: https://www.tuicool.com/articles/vyYFFza
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
许多人在了解到 Python 2.7 即将停止维护后,都开始将他们的 Python 版本从 2 切换到 3。截止到 5 月 19 号上午 10 点,Python 2.7 将终结于...
在这一段时间中,很多优秀开源项目与库已经停止了对 2.7 的支持。例如到今年 1 月份,NumPy 将停止支持 Python 2;到今年年末,Ipython、Cython 和 Pandas 等等都将陆续停止支持 Python 2。
虽然我们都往 3.X 迁移,但许多人编写的 Python 3 代码仍然看起来像 Python 2 一样,只不过加入了一些括号或改了些 API。在本文中,作者将展示一些令人激动的 Python 3.X 新特性。这些特性或方法都是 Python 3 各个版本中新加的,它们相比传统的 Python 方法,更容易解决实践中的一些问题。
所有的示例都是在 Python 3.7 的环境下编写的,每个特性示例都给出了其正常工作所需的最低的 Python 版本。
格式化字符串 f-string(最低 Python 版本为 3.6)
在任何的编程语言中,不使用字符串都是寸步难行的。而为了保持思路清晰,你会希望有一种结构化的方法来处理字符串。大多数使用 Python 的人会偏向于使用「format」方法。
user = "Jane Doe" action = "buy" log_message = 'User {} has logged in and did an action {}.'.format( user, action ) print(log_message) # User Jane Doe has logged in and did an action buy.
除了「format」,Python 3 还提供了一种通过「f-string」进行字符串插入的灵活方法。使用「f-string」编写的与上面功能相同的代码是这样的:
user = "Jane Doe" action = "buy" log_message = f'User {user} has logged in and did an action {action}.' print(log_message) # User Jane Doe has logged in and did an action buy.
相比于常见的字符串格式符 %s 或 format 方法,f-strings 直接在占位符中插入变量显得更加方便,也更好理解。
路径管理库 Pathlib(最低 Python 版本为 3.4)
f-string 非常强大,但是有些像文件路径这样的字符串有他们自己的库,这些库使得对它们的操作更加容易。Python 3 提供了一种处理文件路径的抽象库「pathlib」。如果你不知道为什么应该使用 pathlib,请参阅下面这篇 Trey Hunner 编写的炒鸡棒的博文:「https://treyhunner.com/2018/12/why-you-should-be-using-pathlib/」
from pathlib import Path root = Path('post_sub_folder') print(root) # post_sub_folder path = root / 'happy_user' # Make the path absolute print(path.resolve()) # /home/weenkus/Workspace/Projects/DataWhatNow-Codes/how_your_python3_should_look_like/post_sub_folder/happy_user
如上所示,我们可以直接对路径的字符串进行「/」操作,并在绝对与相对地址间做转换。
类型提示 Type hinting(最低 Python 版本为 3.5)
静态和动态类型是软件工程中一个热门的话题,几乎每个人 对此有自己的看法。读者应该自己决定何时应该编写何种类型,因此你至少需要知道 Python 3 是支持类型提示的。
def sentence_has_animal(sentence: str) -> bool: return "animal" in sentence sentence_has_animal("Donald had a farm without animals") # True
枚举(最低 Python 版本为 3.4)
Python 3 支持通过「Enum」类编写枚举的简单方法。枚举是一种封装常量列表的便捷方法,因此这些列表不会在结构性不强的情况下随机分布在代码中。
from enum import Enum, auto class Monster(Enum): ZOMBIE = auto() WARRIOR = auto() BEAR = auto() print(Monster.ZOMBIE) # Monster.ZOMBIE
枚举是符号名称(成员)的集合,这些符号名称与唯一的常量值绑定在一起。在枚举中,可以通过标识对成员进行比较操作,枚举本身也可以被遍历。
参考:https://docs.python.org/3/library/enum.html
for monster in Monster: print(monster) # Monster.ZOMBIE # Monster.WARRIOR # Monster.BEAR
原生 LRU 缓存(最低 Python 版本为 3.2)
目前,几乎所有层面上的软件和硬件中都需要缓存。Python 3 将 LRU(最近最少使用算法)缓存作为一个名为「lru_cache」的装饰器,使得对缓存的使用非常简单。
下面是一个简单的斐波那契函数,我们知道使用缓存将有助于该函数的计算,因为它会通过递归多次执行相同的工作。
import time def fib(number: int) -> int: if number == 0: return 0 if number == 1: return 1 return fib(number-1) + fib(number-2) start = time.time() fib(40) print(f'Duration: {time.time() - start}s') # Duration: 30.684099674224854s
现在,我们可以使用「lru_cache」来优化它(这种优化技术被称为「memoization」)。通过这种优化,我们将执行时间从几秒降低到了几纳秒。
from functools import lru_cache @lru_cache(maxsize=512) def fib_memoization(number: int) -> int: if number == 0: return 0 if number == 1: return 1 return fib_memoization(number-1) + fib_memoization(number-2) start = time.time() fib_memoization(40) print(f'Duration: {time.time() - start}s') # Duration: 6.866455078125e-05s
扩展的可迭代对象解包(最低 Python 版本为 3.0)
对于这个特性,代码就说明了一切。
参考:https://www.python.org/dev/peps/pep-3132/
head, *body, tail = range(5) print(head, body, tail) # 0 [1, 2, 3] 4 py, filename, *cmds = "python3.7 script.py -n 5 -l 15".split() print(py) print(filename) print(cmds) # python3.7 # script.py # ['-n', '5', '-l', '15'] first, _, third, *_ = range(10) print(first, third) # 0 2
Data class 装饰器(最低 Python 版本为 3.7)
Python 3 引入了「data class」,它们没有太多的限制,可以用来减少对样板代码的使用,因为装饰器会自动生成诸如「__init__()」和「__repr()__」这样的特殊方法。在官方的文档中,它们被描述为「带有缺省值的可变命名元组」。
class Armor: def __init__(self, armor: float, description: str, level: int = 1): self.armor = armor self.level = level self.description = description def power(self) -> float: return self.armor * self.level armor = Armor(5.2, "Common armor.", 2) armor.power() # 10.4 print(armor) # <__main__.Armor object at 0x7fc4800e2cf8>
使用「Data class」实现相同的 Armor 类。
from dataclasses import dataclass @dataclass class Armor: armor: float description: str level: int = 1 def power(self) -> float: return self.armor * self.level armor = Armor(5.2, "Common armor.", 2) armor.power() # 10.4 print(armor) # Armor(armor=5.2, description='Common armor.', level=2)
隐式命名空间包(最低 Python 版本为 3.3)
一种组织 Python 代码文件的方式是将它们封装在程序包中(包含一个「__init__.py」的文件夹)。下面是官方文档提供的示例。
sound/ Top-level package __init__.py Initialize the sound package formats/ Subpackage for file format conversions __init__.py wavread.py wavwrite.py aiffread.py aiffwrite.py auread.py auwrite.py ... effects/ Subpackage for sound effects __init__.py echo.py surround.py reverse.py ... filters/ Subpackage for filters __init__.py equalizer.py vocoder.py karaoke.py ...
在 Python 2 中,上面每个文件夹都必须包含将文件夹转化为 Python 程序包的「__init__.py」文件。在 Python 3 中,随着隐式命名空间包的引入,这些文件不再是必须的了。
sound/ Top-level package __init__.py Initialize the sound package formats/ Subpackage for file format conversions wavread.py wavwrite.py aiffread.py aiffwrite.py auread.py auwrite.py ... effects/ Subpackage for sound effects echo.py surround.py reverse.py ... filters/ Subpackage for filters equalizer.py vocoder.py karaoke.py ...
正如有些人说的那样,这项工作并没有像这篇文章说的那么简单,官方文档「PEP 420 Specification」指出,常规的程序包仍然需要「__init__.py」,把它从一个文件夹中删除会将该文件夹变成一个本地命名空间包,这会带来一些额外的限制。本地命名空间包的官方文档给出了一个很好的示例,并且明确指出了所有的限制。
结语
和网上几乎所有的技术列表一样,本文给出的列表也并不完整。希望这篇文章至少向你展示了一些以前不知道的 Python 3 功能,它将帮助你编写出更加干净、 直观的代码。
最后,本文中给出的所有代码都可以在作者的 GitHub 上找到:https://github.com/Weenkus/DataWhatNow-Codes/blob/master/things_you_are_probably_not_using_in_python_3_but_should/python%203%20examples.ipynb
原文链接:https://datawhatnow.com/things-you-are-probably-not-using-in-python-3-but-should/
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK