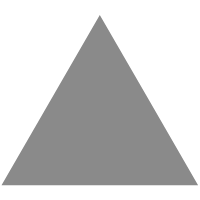
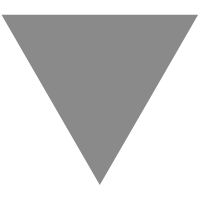
Linux loader for flat binary (DOS like .COM) files
source link: https://www.tuicool.com/articles/Rb2Ejui
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
Back in the olden days of DOS, there was a thing called a .com executable . They were obsolete even then, being basically inherited from CP/M and replaced by DOS MZ executables. Compared to modern binary formats like ELF, .com files were exceedingly simple. There was no file header, no metadata, no division between code and data sections. The operating system would load the entire file into a 16-bit memory segment at offset 0x100, set the stack and the program segment prefix and jump to the first instruction. It was more of a convention than a file format.
While this simplicity created many limitations, it also gave rise to many fun little hacks. You could write a binary executable directly in a text editor . The absence of headers meant that you could make extremely small programs . A minimal executable in Linux these days is somewhere in the vicinity of 10 kB. With some clever hacking you might get it down to a hundred bytes or so. A small .com executable can be on the order of bytes.
A comment on Hacker News recently caught my attention. One could write a .com loader for Linux pretty easily. How easily would that be? I had to try it out.
/* Map address space from 0x00000 to 0x10000. */ <b>void *p = mmap( (void*)0x00000, 0x10000, PROT_EXEC|PROT_READ|PROT_WRITE, MAP_PRIVATE|MAP_FIXED|MAP_ANONYMOUS, -1, 0);</b> /* Load file at address 0x100. */ (a boring loop here reading from a file to memory.) /* Set stack pointer to end of allocated area, jump to 0x100. */ <b>asm( "mov $0x10000, %rsp\n" "jmp 0x100\n" );</b>
This is the gist of it for Linux on x86_64. First we have to map 64 kB of memory at the bottom of our virtual address space. This is where NULL pointers and other such beasts roam, so it's unallocated by default on Linux. In fact, as a security feature the kernel will actively prevent such calls to mmap() . We have to disable this protection first with:
$ <b>sysctl vm.mmap_min_addr=0</b>
Memory mappings have to be aligned with page boundaries, so we can't simply map the file at address 0x100. To work around this we use an anonymous map starting at address 0 and then fill it in manually with the contents of the .com file at the correct offset. Since we have the whole 64 bit linear address space to play with, choosing 0x100 is a bit silly (code section usually lives somewhere around 0x400000 on Linux), but then again, so is this entire exercise.
Once we have everything set up, we set the stack pointer to the top of our 64 kB and jump to the code using some in-line assembly. If we were being pedantic, we could set up the PSP as well. Most of it doesn't make much sense on Linux though (except for command-line parameters maybe). I didn't bother.
Now that we have the loader ready, how do we create a .com binary that will work with it? We have to turn to assembly:
<b> call greet mov rax, 60 mov rdi, 0 syscall </b> # A function call, just to show off our working stack. <b>greet: mov rax, 1 mov rdi, 1 mov rsi, msg mov rdx, 14 syscall ret msg db "Hello, World!", 10</b>
This will compile nicely into an x86_64 ELF object file with NASM . We then have to link it into an ELF executable using a custom linker script that tells the linker that all sections will be placed in a chunk of memory starting at 0x100:
MEMORY { RAM (rwx) : ORIGIN = 0x0100, LENGTH = 0xff00 }
Linker will create an executable which contains our code with all the proper offsets, but still has all the ELF cruft around it (it will segfault nicely if you try to run it with kernel's default ELF loader). As the final step, we must dump its contents into a bare binary file using objcopy :
$ <b>objcopy -S --output-target=binary hello.elf hello.com</b>
Finally, we can run our .com file with our loader:
$ <b>./loader hello.com</b> Hello, World!
As an extra convenience, we can register our new loader with the kernel, so that it will be invoked each time you try to execute a file with the .com extension ( update-binfmts is part of the binfmt-support package on Debian):
$ <b>update-binfmts --install com /path/to/loader --extension com</b> $ <b>./hello.com</b> Hello, World!
And there you have it, a nice 59 byte "Hello, World" binary for Linux. If you want to play with it yourself, see the complete example on GitHub that has a Makefile for your convenience. If you make something small and fun with it, please drop me a note.
One more thing. In case it's not clear at this point, this loader will not work with DOS executables (or CP/M in that case). Those expect to be run in 16-bit real mode and rely on DOS services. Needless to say, my loader makes no attempts to provide those. Code will run in the same environment as other Linux user space processes (albeit at a weird address) and must use the usual kernel syscalls. If you want to run old DOS stuff, use DOSBox or something similar.
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK