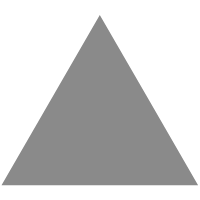
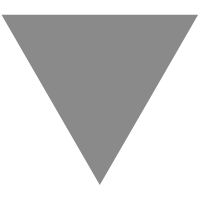
Flutter绘制弯曲虚线
source link: https://tryenough.com/flutter-curved-line?amp%3Butm_medium=referral
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
效果
开始
修改main.dart文件:
import 'package:flutter/material.dart'; void main() => runApp(MyApp()); class MyApp extends StatelessWidget { @override Widget build(BuildContext context) { return MaterialApp( title: "Drawing Shapes", home: DrawingPage(), ); } } class DrawingPage extends StatelessWidget { @override Widget build(BuildContext context) { return Scaffold( appBar: AppBar( title: Text("Curved Line"), ), body: CustomPaint( painter: CurvePainter(), child: Container(), ), ); } } class CurvePainter extends CustomPainter { @override void paint(Canvas canvas, Size size) { } @override bool shouldRepaint(CustomPainter oldDelegate) { return false; } }
接下来我们在CurvePainter中实现绘制:
这里使用贝塞尔曲线绘制,需要4个点,分别是:起点,终点和两个控制点,像图中那样,移动控制点就能改变曲线形状。你可以在 在线工具 中尝试:
我们使用 cubicTo
方法设置路径path:
void cubicTo(double x1, double y1, double x2, double y2, double x3, double y3)
函数绘制路径从当前点到(x3,y3),控制点是(x1,y1),(x2,y2)
正如你看到的, cubicTo
方法接受3个点作为参数,前两个代表控制点,最后一个是结束点。开始点就是你的pen当前坐在canvas中的点。
canvas的坐标系是左上角是(0,0)。右下角是(size.width, size.height)。所以可以尝试调整4个点:
void paint(Canvas canvas, Size size) { var paint = Paint(); paint.color = Colors.lightBlue; paint.style = PaintingStyle.stroke; paint.strokeWidth = 3; var startPoint = Offset(0, size.height / 2); var controlPoint1 = Offset(size.width / 4, size.height / 3); var controlPoint2 = Offset(3 * size.width / 4, size.height / 3); var endPoint = Offset(size.width, size.height / 2); var path = Path(); path.moveTo(startPoint.dx, startPoint.dy); path.cubicTo(controlPoint1.dx, controlPoint1.dy, controlPoint2.dx, controlPoint2.dy, endPoint.dx, endPoint.dy); canvas.drawPath(path, paint); }
paint
对象就是相当于我们的笔,被设置了蓝色和宽度为3.
我们使用path表述贝塞尔曲线。moveTo方法移动笔到开始点的位置。最后使用drawPath方法绘制出来。
虚线
可以是使用三方库绘制虚线: https://pub.dartlang.org/packages/path_drawing
要使用它现在 pubspec.yml文件添加这个库:
dependencies: flutter: sdk: flutter # The following adds the Cupertino Icons font to your application. # Use with the CupertinoIcons class for iOS style icons. cupertino_icons: ^0.1.2 path_drawing: ^0.4.0
运行“flutter packages get”获得库,然后导入头文件就可以使用了:
import 'package:path_drawing/path_drawing.dart';
把上面的路径用dashPath包裹一下就可以了:
canvas.drawPath( dashPath( path, dashArray: CircularIntervalList<double>(<double>[15.0, 10.5]), ), paint, );
dashPath
方法接受两个参数,第一个就是要绘制的path,第二个参数定义每一段虚线的长度和两个虚线间的间隔长度。结果如下:
热度: 4
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK